


Using Java to develop the inventory distribution analysis function of the warehouse management system
Title: Using Java to develop the inventory distribution analysis function of the warehouse management system
Abstract: Warehouse management systems play an important role in modern logistics and supply chain management. Among them, inventory distribution analysis is a key function, which can help companies understand the inventory situation in real time and make reasonable allocation decisions. This article will introduce how to use Java to develop a warehouse management system and provide code examples to implement the inventory distribution analysis function.
- System Architecture Design
The architectural design of the warehouse management system is the key to building a powerful and scalable system. In this example, we will adopt a three-tier architecture design, including the presentation layer, business logic layer and data access layer.
1.1 Presentation layer: The presentation layer is responsible for interacting with users and displaying the results of inventory distribution analysis. User interfaces can be built using Java Swing or JavaFX. For the inventory distribution analysis function, we can design a main interface that provides options such as querying inventory, analyzing inventory distribution, and displaying the results. Users can select actions through buttons or menus on the interface.
1.2 Business logic layer: The business logic layer is responsible for processing business logic, including inventory query, inventory analysis and other functions. In the inventory distribution analysis function, we need to calculate the quantities of different products in different warehouses based on inventory records, and analyze them according to certain rules. The business logic layer should be responsible for these calculation and analysis processes and return the results to the presentation layer.
1.3 Data access layer: The data access layer is responsible for interacting with the database, including reading inventory records and saving inventory analysis results. We can use Java JDBC to access databases, such as MySQL, Oracle, etc.
- Inventory distribution analysis
The inventory distribution analysis function mainly involves the following steps:
2.1 Query inventory records: through the query conditions provided by the user interface (such as Product name, warehouse name, etc.), the business logic layer can call the data access layer to obtain inventory records. You can define an interface in the data access layer, such as "InventoryDAO", and implement the corresponding methods, such as "getInventoryByProduct", "getInventoryByWarehouse", etc.
2.2 Calculate inventory distribution: After obtaining the inventory record, the business logic layer can calculate the inventory distribution information based on the relationship between products and warehouses. For example, you can accumulate inventory quantities by product and warehouse by traversing inventory records. You can define a data structure, such as "InventoryDistribution", to save inventory distribution information for products and warehouses.
2.3 Analyze inventory distribution: Based on the inventory distribution information, the business logic layer can perform various analyses, such as calculating the proportion of a certain product in each warehouse, calculating the proportion of each product in a certain warehouse, etc. Corresponding methods can be defined and implemented in the business logic layer. For example, the "getProductDistribution" method can be implemented to calculate the proportion of a certain product in each warehouse.
- Code examples
The following are some code examples showing how to use Java to implement the inventory distribution analysis function:
// 数据访问层接口 public interface InventoryDAO { List<Inventory> getInventoryByProduct(String productName); List<Inventory> getInventoryByWarehouse(String warehouseName); } // 数据访问层实现类 public class InventoryDAOImpl implements InventoryDAO { // 实现获取库存记录的方法 public List<Inventory> getInventoryByProduct(String productName) { // TODO: 查询数据库,返回符合条件的库存记录 } public List<Inventory> getInventoryByWarehouse(String warehouseName) { // TODO: 查询数据库,返回符合条件的库存记录 } } // 业务逻辑层 public class InventoryService { private InventoryDAO inventoryDAO; public InventoryService() { this.inventoryDAO = new InventoryDAOImpl(); } public List<InventoryDistribution> calculateInventoryDistribution(String productName) { List<Inventory> inventoryList = inventoryDAO.getInventoryByProduct(productName); // TODO: 实现根据库存记录计算库存分布信息的逻辑 return inventoryDistributionList; } public double getProductDistribution(String productName, String warehouseName) { List<Inventory> inventoryList = inventoryDAO.getInventoryByWarehouse(warehouseName); // TODO: 实现根据库存记录计算某个产品在某个仓库中的占比的逻辑 return productDistribution; } } // 用户界面 public class InventoryManagementUI { private InventoryService inventoryService; public InventoryManagementUI() { this.inventoryService = new InventoryService(); } public void analyzeInventoryDistribution(String productName) { List<InventoryDistribution> inventoryDistributionList = inventoryService.calculateInventoryDistribution(productName); // TODO: 实现展示库存分布分析结果的逻辑 } } public class Main { public static void main(String[] args) { InventoryManagementUI ui = new InventoryManagementUI(); ui.analyzeInventoryDistribution("Product A"); } }
This article introduces how to use Java development The inventory distribution analysis function of the warehouse management system and corresponding code examples are provided. Through these examples, you can use them for reference and practice in actual development, helping companies better manage inventory and make reasonable allocation decisions.
The above is the detailed content of Using Java to develop the inventory distribution analysis function of the warehouse management system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
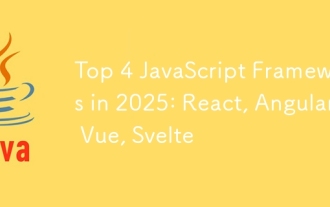
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
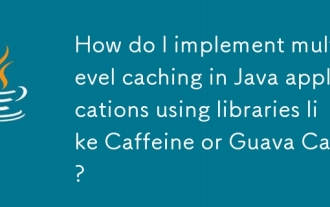
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
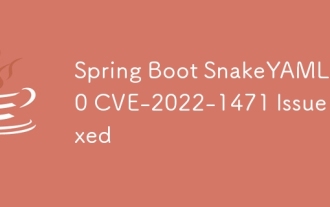
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
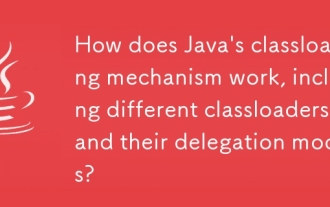
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
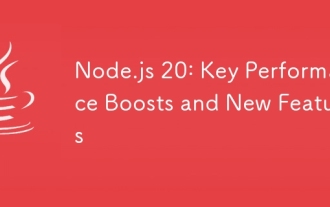
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
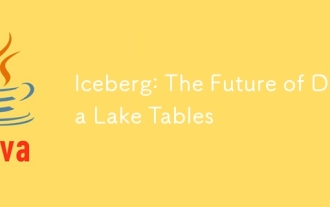
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
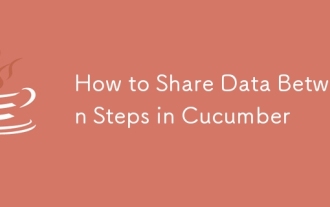
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
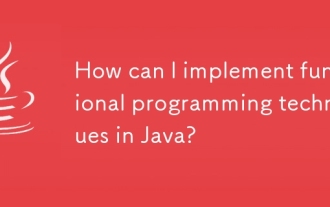
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability
