How to use PHP and Vue to implement data editing functions
How to use PHP and Vue to implement data editing functions
With the development of web applications, data editing functions have become a basic requirement for many applications. In this article, we will introduce how to use PHP and Vue to implement data editing functions, and provide specific code examples.
1. Preparation
Before we start, we need to install PHP and Vue.js and understand their basic concepts and usage.
- Install PHP
PHP is a very popular server-side scripting language that can be used to handle web requests and responses. You can download and install PHP from the official website (https://www.php.net/). - Install Vue.js
Vue.js is a JavaScript framework for building user interfaces. You can download Vue.js from the official website (https://vuejs.org/) and introduce it into your HTML page.
2. Create database and table
Before we start writing code, we need to create a database and a table to store our data. Taking MySQL as an example, you can use the following code to create a database named "users" and a table named "students":
CREATE DATABASE users; USE users; CREATE TABLE students ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(50), age INT, email VARCHAR(50) );
3. Write PHP code
First, we need to write A PHP file to handle the backend logic. We will create a file named "edit.php" and write the following code in it:
<?php // 连接到数据库 $mysqli = new mysqli('localhost', 'root', 'password', 'users'); // 检查连接是否成功 if($mysqli->connect_errno) { die('连接数据库失败:' . $mysqli->connect_error); } // 获取POST数据 $id = $_POST['id']; $name = $_POST['name']; $age = $_POST['age']; $email = $_POST['email']; // 更新数据 $query = "UPDATE students SET name='$name', age='$age', email='$email' WHERE id='$id'"; $result = $mysqli->query($query); // 返回结果 if($result) { echo '数据更新成功'; } else { echo '数据更新失败'; } // 关闭数据库连接 $mysqli->close(); ?>
The above code implements the functions of connecting to the database, updating data and returning results.
4. Write Vue code
Next, we need to write a Vue component to handle front-end interaction. We will create a file named "EditForm.vue" and write the following code in it:
<template> <div> <input v-model="name" type="text" placeholder="姓名"> <input v-model="age" type="text" placeholder="年龄"> <input v-model="email" type="text" placeholder="邮箱"> <button @click="editData">更新</button> </div> </template> <script> export default { data() { return { id: '', // 数据的ID name: '', // 姓名 age: '', // 年龄 email: '' // 邮箱 } }, methods: { editData() { // 发送请求 axios.post('/edit.php', { id: this.id, name: this.name, age: this.age, email: this.email }) .then(response => { console.log(response.data); }) .catch(error => { console.log(error); }) } } } </script>
The above code implements a form in which the user can enter data and update it by clicking the "Update" button The data is sent to the backend for updates.
5. Integrate code
Finally, we need to integrate the PHP and Vue code in an HTML page. We will create a file named "edit.html" and write the following code in it:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>数据编辑</title> </head> <body> <div id="app"> <edit-form></edit-form> </div> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script> <script src="EditForm.vue"></script> <script> new Vue({ el: '#app', components: { EditForm } }) </script> </body> </html>
The above code introduces the Vue component into the HTML page and creates a Vue instance.
6. Test Code
Now, you can open the "edit.html" file in the browser and try to edit the data. When you click the "Update" button, the data will be sent to the backend for update and the results will be displayed in the console.
Summary
In this article, we introduced how to use PHP and Vue to implement data editing functions, and provided specific code examples. By learning and understanding these codes, you can quickly get started and apply them to your own projects. Hope this article can be helpful to you!
The above is the detailed content of How to use PHP and Vue to implement data editing functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


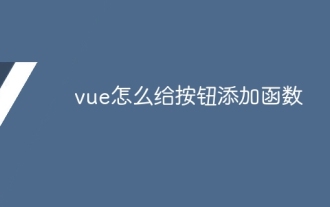
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
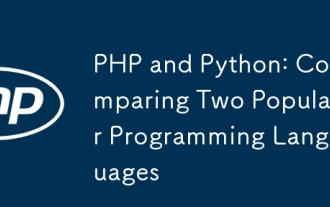
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.

The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
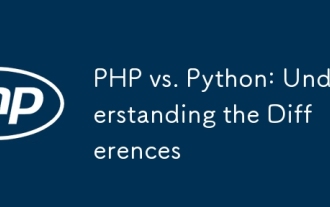
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
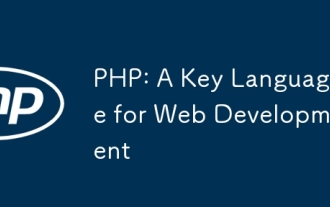
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
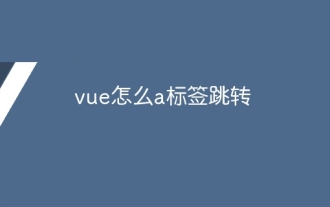
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
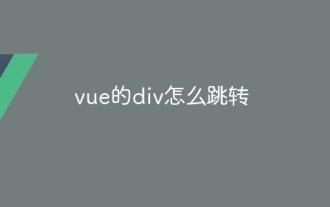
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.
