


PHP and Vue development: How to implement the lottery mechanism for member points
PHP and Vue development: How to implement the lottery mechanism for member points
Lottery activities have always been one of the important ways to attract members to participate. For e-commerce platforms, implementing a lottery mechanism through member points is a very good form of promotion and feedback. This article will introduce how to use PHP and Vue to develop a lottery mechanism for member points, and give specific code examples.
First, we need to create a member points data table to store the points information of each member. This table can contain fields including member ID, number of points, and other related information. On this basis, we also need to create a data table for the lottery, which is used to store relevant information for each lottery, such as event name, start time, end time, participation conditions, etc.
Next, we need to implement the function of increasing and decreasing member points. When members shop or complete certain tasks, we can add corresponding points to them according to certain rules. In PHP, we can implement this function through database operations. The specific code is as follows:
// 增加会员积分 function addMemberPoints($memberID, $points) { $conn = mysqli_connect("localhost", "username", "password", "database"); $sql = "UPDATE members SET points = points + $points WHERE memberID = $memberID"; mysqli_query($conn, $sql); mysqli_close($conn); }
Similarly, if members need to use points to redeem goods or participate in lottery activities, we also need to deduct their corresponding points accordingly. Points, the specific code is as follows:
// 扣除会员积分 function deductMemberPoints($memberID, $points) { $conn = mysqli_connect("localhost", "username", "password", "database"); $sql = "UPDATE members SET points = points - $points WHERE memberID = $memberID"; mysqli_query($conn, $sql); mysqli_close($conn); }
After completing the function of increasing and decreasing member points, we still need to implement the logic of the lottery. Here, we use Vue to implement front-end interactive operations. The specific code is as follows:
<template> <div> <button @click="drawLottery">抽奖</button> <div v-if="prize">{{ prize }}</div> </div> </template> <script> export default { data() { return { prize: "" }; }, methods: { drawLottery() { // 向后端发送请求抽奖 axios.get("/api/lottery").then(response => { this.prize = response.data; }); } } }; </script>
In the Vue page, we trigger the lottery action by clicking the button. On the backend, we need to implement an interface to handle the logic of the lottery. The specific code is as follows:
// 处理抽奖请求 function handleLotteryRequest() { $conn = mysqli_connect("localhost", "username", "password", "database"); $sql = "SELECT * FROM lottery WHERE start_time <= NOW() AND end_time >= NOW()"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { $prizes = array(); while ($row = mysqli_fetch_assoc($result)) { array_push($prizes, $row["prize"]); } $randomIndex = array_rand($prizes); $prize = $prizes[$randomIndex]; // 扣除用户积分 deductMemberPoints($memberID, $points); // 返回抽奖结果 echo $prize; } else { echo "当前没有抽奖活动"; } mysqli_close($conn); }
In the logic of the lottery, we first query the database to obtain the currently ongoing lottery. Then, a prize is randomly selected as the draw result. Subsequently, we deduct the user's corresponding points and return the results to the front-end page.
Finally, in the Vue page, we can display different prompt information based on the lottery results.
Through the above code example, we can implement the lottery mechanism for member points. Using the development technology of PHP and Vue, we can provide members with a better shopping experience, while also promoting their active participation and interaction. However, it should be noted that in practical applications, we also need to consider issues such as security and performance to meet higher actual needs.
The above is the detailed content of PHP and Vue development: How to implement the lottery mechanism for member points. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



In PHP, the final keyword is used to prevent classes from being inherited and methods being overwritten. 1) When marking the class as final, the class cannot be inherited. 2) When marking the method as final, the method cannot be rewritten by the subclass. Using final keywords ensures the stability and security of your code.
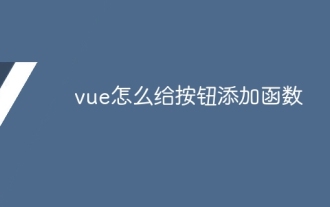
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
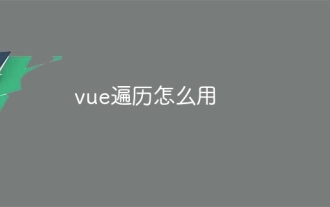
There are three common methods for Vue.js to traverse arrays and objects: the v-for directive is used to traverse each element and render templates; the v-bind directive can be used with v-for to dynamically set attribute values for each element; and the .map method can convert array elements into new arrays.
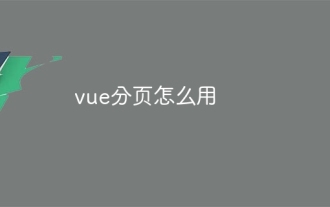
Pagination is a technology that splits large data sets into small pages to improve performance and user experience. In Vue, you can use the following built-in method to paging: Calculate the total number of pages: totalPages() traversal page number: v-for directive to set the current page: currentPage Get the current page data: currentPageData()

Function interception in Vue is a technique used to limit the number of times a function is called within a specified time period and prevent performance problems. The implementation method is: import the lodash library: import { debounce } from 'lodash'; Use the debounce function to create an intercept function: const debouncedFunction = debounce(() => { / Logical / }, 500); Call the intercept function, and the control function is called at most once in 500 milliseconds.
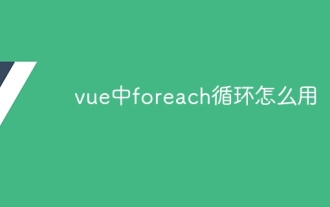
The foreach loop in Vue.js uses the v-for directive, which allows developers to iterate through each element in an array or object and perform specific operations on each element. The syntax is as follows: <template> <ul> <li v-for="item in items>>{{ item }}</li> </ul> </template>&am
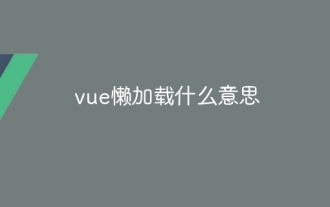
In Vue.js, lazy loading allows components or resources to be loaded dynamically as needed, reducing initial page loading time and improving performance. The specific implementation method includes using <keep-alive> and <component is> components. It should be noted that lazy loading can cause FOUC (splash screen) issues and should be used only for components that need lazy loading to avoid unnecessary performance overhead.
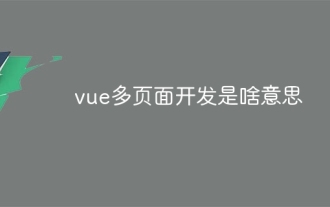
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
