How to use PHP and Vue to implement chart display function
How to use PHP and Vue to implement chart display function
In modern web development, chart display is a very common requirement. With the help of chart display, we can visually present various data to help users better understand and analyze the data. This article will introduce how to use PHP and Vue.js to realize the chart display function, and provide specific code examples.
First, we need to choose a chart library. Here, we choose echarts, a powerful and easy-to-use charting library. echarts provides a wealth of chart types and interactive functions, and supports various data formats. We can introduce the echarts library file through CDN, or download it locally for use.
Next, we need to prepare the data. In this article, we write an interface using PHP to get data from the backend and return it to the frontend. In PHP, we can use PDO extensions and databases for data query operations, or obtain data from other APIs through HTTP requests. The following is a simple PHP interface example:
<?php // 连接数据库 $dsn = 'mysql:host=localhost;dbname=test;charset=utf8'; $username = 'root'; $password = ''; $db = new PDO($dsn, $username, $password); // 查询数据 $sql = 'SELECT * FROM data'; $stmt = $db->query($sql); $data = $stmt->fetchAll(PDO::FETCH_ASSOC); // 返回数据 header('Content-Type: application/json'); echo json_encode($data); ?>
In the above code, we first use PDO to connect to the database and perform a query operation to obtain data. Finally, we set the response header to JSON format and encode the data into a JSON string and return it to the front end.
On the front end, we use Vue.js to build the view and send HTTP requests to obtain data through the axios library. The following is a simple Vue component example:
<template> <div id="app"> <div id="chart"></div> </div> </template> <script> import echarts from 'echarts'; import axios from 'axios'; export default { mounted() { // 发送 HTTP 请求获取数据 axios.get('/api/data.php') .then(response => { const data = response.data; // 渲染图表 const chart = echarts.init(document.getElementById('chart')); const options = { // 选择图表类型和配置 // ... }; chart.setOption(options); }) .catch(error => { console.error(error); }); } } </script>
In the above code, we send an HTTP request to obtain data in the mounted
hook function of the Vue component. After obtaining the data, we use echarts to initialize the chart and select the chart type and configuration according to actual needs. Finally, use the setOption
method to apply the configuration to the chart.
Of course, the above example is only a simple demonstration, and the actual chart display function may require more configuration and interaction. echarts provides a powerful API that can flexibly meet various needs. At the same time, PHP and Vue.js also provide more functions and features, which can be used in conjunction with the chart display function for more complex development.
To sum up, it is a common solution to realize the chart display function through the combination of PHP and Vue.js. By providing a data interface through PHP, sending HTTP requests through Vue.js and using echarts to render charts, you can quickly achieve cool chart display effects. Of course, in actual development, details such as data format processing, chart type selection, and implementation of interactive functions also need to be considered. I hope this article can provide some help to you in using PHP and Vue.js to implement chart display functions.
The above is the detailed content of How to use PHP and Vue to implement chart display function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
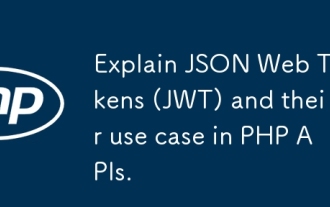
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
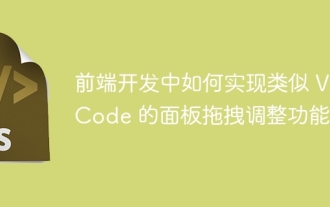
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
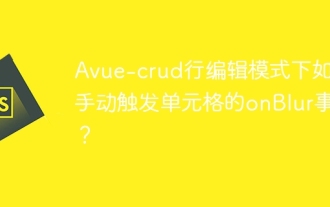
The onBlur event that implements Avue-crud row editing in the Avue component library manually triggers the Avue-crud component. It provides convenient in-line editing functions, but sometimes we need to...
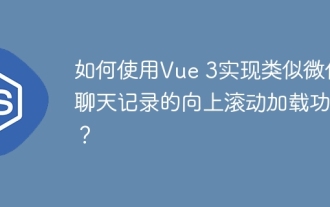
How to achieve upward scrolling loading similar to WeChat chat records? When developing applications similar to WeChat chat records, a common question is how to...
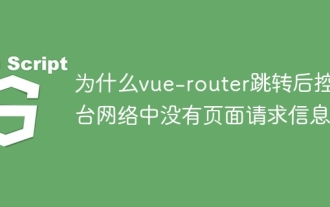
Why is there no page request information on the console network after vue-router jump? When using vue-router for page redirection, you may notice a...
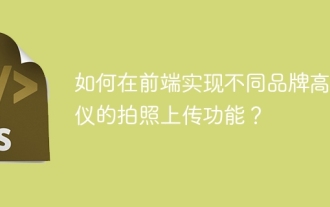
How to implement the photo upload function of different brands of high-photographers on the front end When developing front-end projects, you often encounter the need to integrate hardware equipment. for...
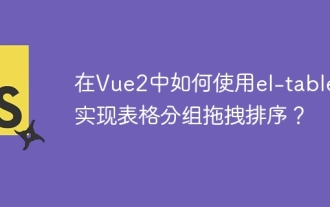
Implementing el-table table group drag and drop sorting in Vue2. Using el-table tables to implement group drag and drop sorting in Vue2 is a common requirement. Suppose we have a...
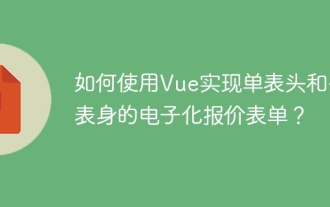
How to implement electronic quotation forms with single header and multi-body in Vue. In modern enterprise management, the electronic processing of quotation forms is to improve efficiency and...
