


How to use PHP to write an employee attendance data scrapping program?
How to use PHP to write an employee attendance data scrapping program?
In many companies, employee attendance data is very important. These data include employees’ work clock records, leave records, and overtime records. However, old attendance data can take up a lot of storage space, and over time, the data may become no longer needed. Therefore, writing an employee attendance data retirement program can help enterprises manage and clean up this data easily.
PHP is a powerful server-side scripting language that is very suitable for developing web applications. Below, we will introduce how to use PHP to write an employee attendance data scrapping program to help enterprises manage attendance data efficiently.
- Connect to the database
First, we need to connect to the database, because attendance data is usually stored in the database. You can use MySQL or other relational databases to store data. The following is a sample code to connect to the database:
<?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "database"; $conn = new mysqli($servername, $username, $password, $dbname); if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } echo "Connected successfully"; ?>
In this example, we use MySQL as the database. You will need to replace username
, password
, and database
with your own database credentials and database name.
- Query attendance data
Next, we need to write code to query attendance data. You can use SQL statements to query data in the database. Here is a sample code:
<?php $sql = "SELECT * FROM attendance WHERE date < '2022-01-01'"; $result = $conn->query($sql); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { // 处理数据 } } else { echo "No data found"; } $conn->close(); ?>
In this example, we use the attendance
table to store attendance data. We queried all data with a date earlier than January 1, 2022. You can modify the SQL statement according to the actual situation to query data that meets the conditions.
- Processing attendance data
Once the attendance data is queried, we can start processing the data. For example, you can export data to a file, backup it, or move it to other database tables. Here is a sample code:
<?php // 假设我们要将数据导出到CSV文件 $file = fopen("attendance.csv", "w"); fputcsv($file, array('ID', 'Date', 'Time')); while($row = $result->fetch_assoc()) { fputcsv($file, $row); } fclose($file); ?>
In this example, we export the attendance data to a CSV file named attendance.csv
. You can modify the file name and export format according to actual needs.
- Clean attendance data
Finally, we need to clean up the attendance data to free up storage space. You can use SQL statements to delete data that is no longer needed. Below is a sample code:
<?php $sql = "DELETE FROM attendance WHERE date < '2022-01-01'"; $result = $conn->query($sql); if ($conn->affected_rows > 0) { echo "Data deleted successfully"; } else { echo "No data found to delete"; } $conn->close(); ?>
In this example, we delete all attendance data with dates earlier than January 1, 2022. You can modify the SQL statement according to the actual situation to delete data that meets the conditions.
The above is an example of a basic employee attendance data scrapping program. You can further expand and optimize the program based on actual needs and business logic. Remember, writing efficient programs can help companies better manage and clean employee attendance data. Hope this article helps you!
The above is the detailed content of How to use PHP to write an employee attendance data scrapping program?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




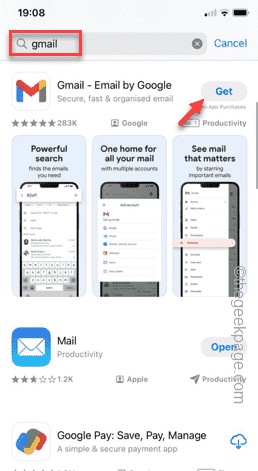
The default map on the iPhone is Maps, Apple's proprietary geolocation provider. Although the map is getting better, it doesn't work well outside the United States. It has nothing to offer compared to Google Maps. In this article, we discuss the feasible steps to use Google Maps to become the default map on your iPhone. How to Make Google Maps the Default Map in iPhone Setting Google Maps as the default map app on your phone is easier than you think. Follow the steps below – Prerequisite steps – You must have Gmail installed on your phone. Step 1 – Open the AppStore. Step 2 – Search for “Gmail”. Step 3 – Click next to Gmail app
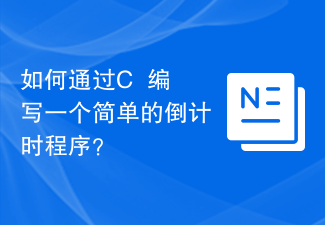
C++ is a widely used programming language that is very convenient and practical in writing countdown programs. Countdown program is a common application that can provide us with very precise time calculation and countdown functions. This article will introduce how to use C++ to write a simple countdown program. The key to implementing a countdown program is to use a timer to calculate the passage of time. In C++, we can use the functions in the time.h header file to implement the timer function. The following is the code for a simple countdown program
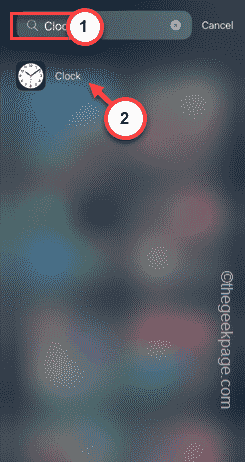
Is the clock app missing from your phone? The date and time will still appear on your iPhone's status bar. However, without the Clock app, you won’t be able to use world clock, stopwatch, alarm clock, and many other features. Therefore, fixing missing clock app should be at the top of your to-do list. These solutions can help you resolve this issue. Fix 1 – Place the Clock App If you mistakenly removed the Clock app from your home screen, you can put the Clock app back in its place. Step 1 – Unlock your iPhone and start swiping to the left until you reach the App Library page. Step 2 – Next, search for “clock” in the search box. Step 3 – When you see “Clock” below in the search results, press and hold it and
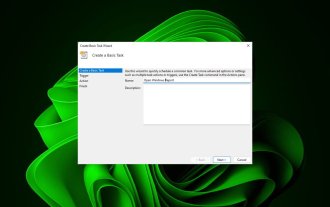
Do you frequently visit the same website at about the same time every day? This can lead to spending a lot of time with multiple browser tabs open and cluttering the browser while performing daily tasks. Well, how about opening it without having to launch the browser manually? It's very simple and doesn't require you to download any third-party apps, as shown below. How do I set up Task Scheduler to open a website? Press the key, type Task Scheduler in the search box, and then click Open. Windows On the right sidebar, click on the Create Basic Task option. In the Name field, enter the name of the website you want to open and click Next. Next, under Triggers, click Time Frequency and click Next. Select how long you want the event to repeat and click Next. Select enable
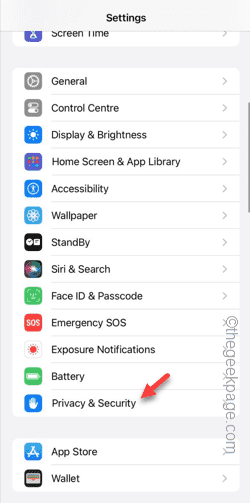
Are you getting "Unable to allow access to camera and microphone" when trying to use the app? Typically, you grant camera and microphone permissions to specific people on a need-to-provide basis. However, if you deny permission, the camera and microphone will not work and will display this error message instead. Solving this problem is very basic and you can do it in a minute or two. Fix 1 – Provide Camera, Microphone Permissions You can provide the necessary camera and microphone permissions directly in settings. Step 1 – Go to the Settings tab. Step 2 – Open the Privacy & Security panel. Step 3 – Turn on the “Camera” permission there. Step 4 – Inside, you will find a list of apps that have requested permission for your phone’s camera. Step 5 – Open the “Camera” of the specified app
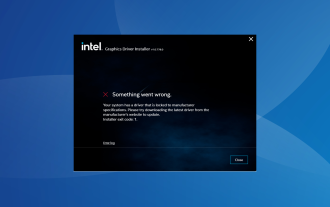
Graphics driver is one of the most important drivers on your PC, directly affecting performance and gaming experience. However, many people encounter the "Your system has drivers locked to manufacturer specifications" error when updating drivers through the installer. This issue occurs because the manufacturer restricts updates unless they are specifically pushed from their end. This ensures stability, but may be an issue for many people. So, let’s find out how to solve the problem now! How to fix your system with drivers locked to manufacturer specifications on Windows 11? Before we move on to slightly more complicated solutions, try these quick fixes: Make sure your computer and operating system meet the driver's system requirements. Boot your computer into safe mode, then
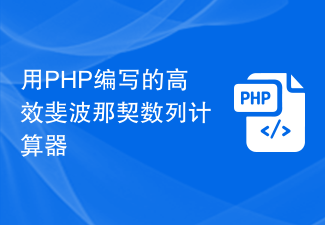
Efficient Fibonacci sequence calculator: PHP implementation of Fibonacci sequence is a very classic mathematical problem. The rule is that each number is equal to the sum of the previous two numbers, that is, F(n)=F(n -1)+F(n-2), where F(0)=0 and F(1)=1. When calculating the Fibonacci sequence, it can be implemented recursively, but performance problems will occur as the value increases. Therefore, this article will introduce how to write an efficient Fibonacci using PHP
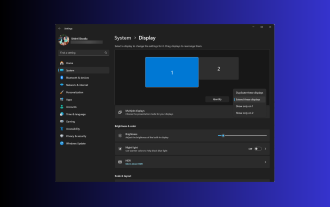
If you're using a multi-monitor setup and your apps keep opening on the wrong monitor in Windows 11, this guide can help! We'll discuss some practical ways to turn off multiple monitors on Windows so that your apps open on the primary monitor. Why do programs open on my second monitor? Previously used the program on a second monitor and closed it while the app was on it. Display settings in the operating system may be configured to open new programs on this screen. The secondary monitor has been reconnected or the monitor configuration has been changed. Some apps have glitches or bugs that may cause them to open on the wrong monitor, regardless of settings. How to make a program open Windo on a specific monitor
