


Use PHP and Vue to implement discounts for member points after payment
Using PHP and Vue to implement discounts and discounts for member points after payment
With the rapid development of e-commerce, more and more companies are beginning to pay attention to and utilize members Points to attract and retain customers. Offering discounts to members after payment is one common method. This article will introduce how to use PHP and Vue to implement discounts for member points after payment, and provide specific code examples.
1. Requirements Analysis
Before starting to write code, we first need to clarify the requirements. We will implement a simple e-commerce website where users can purchase goods through payment. After successful payment, members will enjoy corresponding discounts based on their points level.
The specific requirements are as follows:
- Users will receive a certain number of points after registering as a member.
- When users purchase goods, they can choose to use a certain number of points for discounts.
- The discount ratio is determined based on the user's points level. The higher the points level, the greater the discount.
- After successful payment, the member’s points will be reduced accordingly.
2. Technical Implementation
In order to achieve the above requirements, we can use PHP as the back-end language to process business logic, and use Vue as the front-end framework for data interaction and display. The following are the steps to implement:
- Create database
First we need to create a database containing two tables: user table and product table.
User table fields include: User ID (UserID), Username (Username), Password (Password), Points (Points), etc.
The product table fields include: product ID (ProductID), product name (ProductName), product price (Price), etc.
- PHP backend
(1) User registration
When a user registers, we need to initialize a certain number of points for the user.
<?php // 用户注册 function register($username, $password) { // 验证用户名和密码 // ... // 初始化用户积分 $points = 100; // 初始积分为100 // 插入用户表 $query = "INSERT INTO user (Username, Password, Points) VALUES ('$username', '$password', $points)"; // 执行插入操作 // ... } ?>
(2) Obtain user points level
According to user points, we can determine the user's points level and then determine the discount ratio.
<?php // 获取用户积分等级 function getPointsLevel($points) { // 根据积分等级规则,计算积分等级 if ($points >= 0 && $points < 100) { return 1; // 积分等级1 } else if ($points >= 100 && $points < 200) { return 2; // 积分等级2 } else if ($points >= 200 && $points < 300) { return 3; // 积分等级3 } else { return 4; // 积分等级4 } } ?>
(3) Processing payment
After the user pays successfully, we need to calculate the discount amount based on the number of points selected by the user and the product price, and update the user's points.
<?php // 处理支付 function handlePayment($userId, $productId, $pointsUsed) { // 查询用户积分 $query = "SELECT Points FROM user WHERE UserID = $userId"; // 执行查询操作 // ... // 获取用户积分 $points = 100; // 假设用户积分为100 // 查询商品价格 $query = "SELECT Price FROM product WHERE ProductID = $productId"; // 执行查询操作 // ... // 获取商品价格 $price = 100; // 假设商品价格为100 // 计算折扣金额 $discount = $pointsUsed * 0.1; // 假设折扣比例为0.1 // 更新用户积分 $newPoints = $points - $pointsUsed; $query = "UPDATE user SET Points = $newPoints WHERE UserID = $userId"; // 执行更新操作 // ... // 返回折扣金额 return $discount; } ?>
- Vue front-end
(1) User registration
On the user registration page, the user needs to fill in the user name and password, and submit the form by clicking the registration button.
<template> <div> <input v-model="username" placeholder="Username"> <input v-model="password" placeholder="Password"> <button @click="register">Register</button> </div> </template> <script> export default { data() { return { username: '', password: '' }; }, methods: { register() { // 调用后端注册接口 // ... } } } </script>
(2) Payment page
On the payment page, the user needs to select the number of points to use and submit the form by clicking the payment button.
<template> <div> <select v-model="pointsUsed"> <option v-for="point in points" :value="point">{{ point }}</option> </select> <button @click="handlePayment">Pay</button> </div> </template> <script> export default { data() { return { points: [0, 50, 100, 150, 200], // 假设可用积分 pointsUsed: 0 // 默认使用积分0 }; }, methods: { handlePayment() { // 调用后端支付接口 // ... } } } </script>
The above is a simple example of using PHP and Vue to implement discounts for member points after payment. In actual projects, we need to make appropriate modifications and expansions according to specific needs. Hope this article is helpful to you!
The above is the detailed content of Use PHP and Vue to implement discounts for member points after payment. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


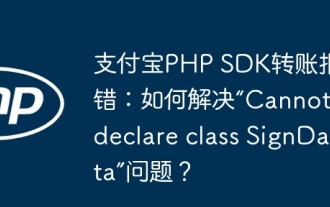
Alipay PHP...
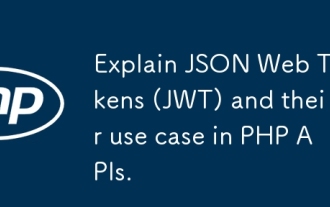
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
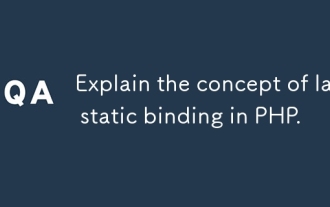
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
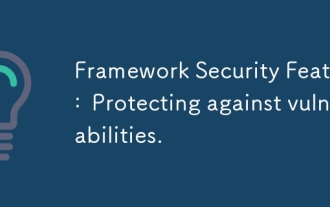
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
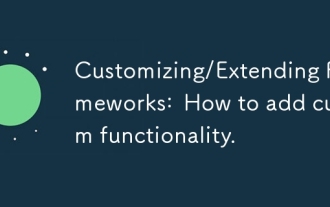
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
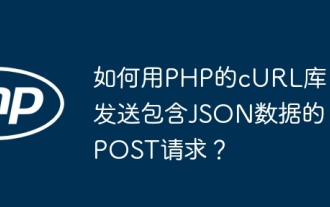
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
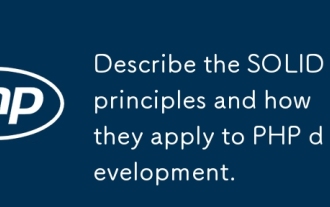
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
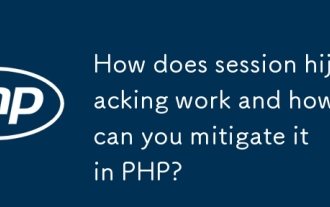
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
