


How to use PHP to develop employee attendance data query tool?
How to use PHP to develop employee attendance data query tool?
Abstract: This article will introduce how to use PHP to develop a simple employee attendance data query tool. We will store employee attendance data through the MySQL database, and use PHP to write the query page and database connection code.
Keywords: PHP, employee attendance data, query tools, MySQL, database connection
1. Preparation work
- First, we need to install it in the local environment PHP and MySQL, make sure they are running properly.
- Create a MySQL database to store employee attendance data. You can use the following SQL statement to create a simple table to store data:
CREATE TABLE attendance ( id INT PRIMARY KEY AUTO_INCREMENT, emp_id INT NOT NULL, date DATE NOT NULL, time_in TIME NOT NULL, time_out TIME, status ENUM('Present', 'Absent') NOT NULL );
2. Write the database connection code
- In the root directory of the project, create a table named "dbconn.php" file. This file will be used for database connections and referenced in other files. In "dbconn.php", write the following code:
<?php $servername = "localhost"; $username = "your_username"; $password = "your_password"; $dbname = "your_database_name"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } ?>
Please replace "your_username", "your_password" and "your_database_name" with your MySQL connection credentials and database name.
3. Write the query page code
- Create a file named "index.php" in the project root directory as the employee attendance data query page. Write the following code:
<?php include('dbconn.php'); $query = "SELECT * FROM attendance"; $result = $conn->query($query); ?> <!DOCTYPE html> <html> <head> <title>员工考勤数据查询工具</title> </head> <body> <h1 id="员工考勤数据查询工具">员工考勤数据查询工具</h1> <table> <tr> <th>ID</th> <th>员工ID</th> <th>日期</th> <th>签到时间</th> <th>签退时间</th> <th>状态</th> </tr> <?php if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { echo "<tr>"; echo "<td>" . $row['id'] . "</td>"; echo "<td>" . $row['emp_id'] . "</td>"; echo "<td>" . $row['date'] . "</td>"; echo "<td>" . $row['time_in'] . "</td>"; echo "<td>" . $row['time_out'] . "</td>"; echo "<td>" . $row['status'] . "</td>"; echo "</tr>"; } } else { echo "没有可用的数据"; } ?> </table> </body> </html>
4. Run the query tool
- Save the above code and place the project in the root folder of your Web server.
- Enter your project URL in a web browser, such as "localhost/your_project_folder/index.php".
- You will see a simple employee attendance data query page, which displays the data retrieved from the database by the SELECT query.
Conclusion:
By following the steps in this article, you can develop a simple employee attendance data query tool using PHP. By modifying the database connection code and query page code, you can adapt it to any data set and need. I hope this article can help you quickly build an employee attendance data query tool.
The above is the detailed content of How to use PHP to develop employee attendance data query tool?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


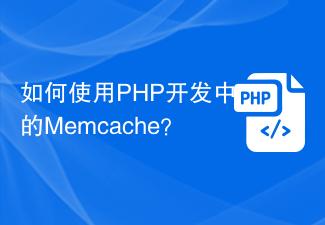
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
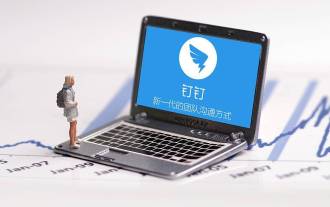
Everyone is familiar with the DingTalk platform. It is a very easy-to-use office software that provides convenience to users. It has a lot of rich functions to help companies better manage their employees. So does anyone know how to check employee attendance on DingTalk? Let’s take a look. Detailed steps to view employee attendance on DingTalk: 1. Open the DingTalk app and click "Attendance Punch" on the workbench. 2. Click [Statistics], click [Team Statistics], and click [Export Report] to see employee attendance. Software Features 1. The software has many convenient office functions and is a must-have artifact for your workplace; 2. The 2021 new version of DingTalk has added many new functions, providing a focused, efficient and secure instant messaging solution, allowing
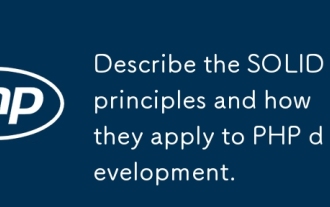
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
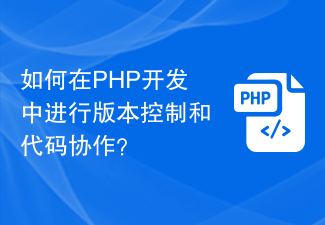
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
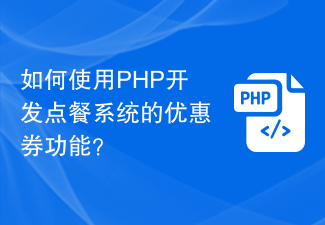
How to use PHP to develop the coupon function of the ordering system? With the rapid development of modern society, people's life pace is getting faster and faster, and more and more people choose to eat out. The emergence of the ordering system has greatly improved the efficiency and convenience of customers' ordering. As a marketing tool to attract customers, the coupon function is also widely used in various ordering systems. So how to use PHP to develop the coupon function of the ordering system? 1. Database design First, we need to design a database to store coupon-related data. It is recommended to create two tables: one
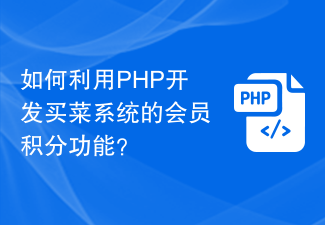
How to use PHP to develop the member points function of the grocery shopping system? With the rise of e-commerce, more and more people choose to purchase daily necessities online, including grocery shopping. The grocery shopping system has become the first choice for many people, and one of its important features is the membership points system. The membership points system can attract users and increase their loyalty, while also providing users with an additional shopping experience. In this article, we will discuss how to use PHP to develop the membership points function of the grocery shopping system. First, we need to create a membership table to store users
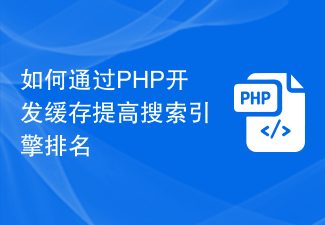
How to improve search engine rankings through PHP cache development Introduction: In today's digital era, the search engine ranking of a website is crucial to the website's traffic and exposure. In order to improve the ranking of the website, an important strategy is to reduce the loading time of the website through caching. In this article, we'll explore how to improve search engine rankings by developing caching with PHP and provide concrete code examples. 1. The concept of caching Caching is a technology that stores data in temporary storage so that it can be quickly retrieved and reused. for net
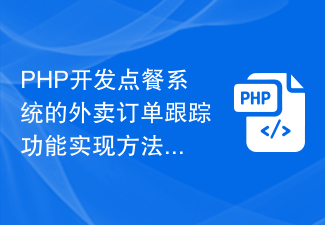
With the booming takeout business, major restaurants and takeout platforms are competing to launch ordering systems. The takeout order tracking function has become a feature that both customers and restaurants are paying close attention to. So, how do we implement the takeout order tracking function in the ordering system developed in PHP? 1. Front-end page design First, we need to design a front-end page so that users can easily check the order status. The following points need to be noted in the design of the front-end page: the interface is simple and clear, and users can quickly find the entrance to the order tracking function. In the process of order tracking
