


How to implement concurrency control and current limiting functions in PHP microservices
How to implement concurrency control and current limiting functions in PHP microservices
Introduction:
With the rapid development of the Internet and the sharp increase in the number of users, for The requirements for server-side concurrency control and current limiting functions are also getting higher and higher. This article will introduce how to implement concurrency control and current limiting functions in PHP microservices, and provide specific code examples.
1. Requirements for concurrency control
In traditional single applications, the demand for concurrency control is not very high. However, in a microservice architecture, each service is deployed and run independently and may face a large number of concurrent requests. If left unchecked, it can result in high server load, long response times, or even crashes. Therefore, it is crucial to implement concurrency control functions in microservices.
2. Methods to achieve concurrency control
- Use semaphore (Semaphore)
Semaphore is a common concurrency control mechanism, which can Control the number of concurrent threads that access a resource at the same time. In PHP, semaphores can be implemented using the Semaphore extension.
First, we need to install the Semaphore extension. It can be installed through the following command line:
$ pecl install sem
After installing the extension, we can use the Semaphore class to implement concurrency control. The following is a simple code example:
// 初始化信号量,参数1表示资源的数量 $sem = new Semaphore(10); // 等待可用资源 $sem->acquire(); // 执行需要控制并发的代码 // 释放资源 $sem->release();
In the above example, we initialize a semaphore containing 10 resources. Every time you execute code that requires concurrency control, use the acquire method to wait for available resources, and use the release method to release the resources after execution.
- Using queues
Queue is a common concurrency control mechanism that can process concurrent requests in order. In PHP, you can use message queues to achieve concurrency control.
There are many message queue implementations in PHP, such as RabbitMQ, Redis, etc. Here we take Redis as an example to introduce how to use Redis to implement concurrency control.
First, we need to install the Redis extension and Predis library. It can be installed through the following command line:
$ pecl install redis $ composer require predis/predis
After installing the extension and library, we can use the Redis List type to implement the queue. The following is a sample code:
// 连接Redis $redis = new PredisClient(); // 将请求添加到队列 $redis->rpush('request_queue', time()); // 从队列中取出请求 $request = $redis->lpop('request_queue'); // 执行需要控制并发的代码 // 删除已处理的请求 $redis->lrem('request_queue', 0, $request);
In the above example, we add the request to the queue through the rpush method and remove the request from the queue through the lpop method for processing. After processing, delete the processed request through the lrem method.
3. The need for current limiting
Current limiting is a common server-side method of controlling concurrent requests, which can limit the number of requests per unit time. Using throttling in microservices can protect backend services from excessive request pressure.
4. Methods to implement current limiting
- Use the token bucket algorithm
The token bucket algorithm is one of the common current limiting algorithms. A fixed-capacity token bucket is maintained, and each request requires one token to be processed. When there are not enough tokens in the bucket, the request will be rejected.
The following is a sample code for the token bucket algorithm implemented using Redis:
// 连接Redis $redis = new PredisClient(); // 每秒生成10个令牌 $rate = 10; // 桶的容量为20个令牌 $capacity = 20; // 获取当前时间 $time = microtime(true); // 计算当前桶中的令牌数量 $tokens = $redis->get('tokens'); // 重新计算桶中的令牌数量 if ($tokens === null) { $tokens = $capacity; $redis->set('tokens', $tokens); $redis->set('last_time', $time); } else { $interval = $time - $redis->get('last_time'); $newTokens = $interval * $rate; $tokens = min($tokens + $newTokens, $capacity); $redis->set('tokens', $tokens); $redis->set('last_time', $time); } // 判断是否有足够的令牌 $allow = $redis->get('tokens') >= 1; if ($allow) { // 消耗一个令牌 $redis->decr('tokens'); // 执行请求处理代码 } else { // 拒绝请求 }
In the above example, we use Redis to store the number of tokens in the bucket and the last update time . Each time a request arrives, the number of new tokens is calculated by comparing the time interval between the current time and the last update time, and then the number of tokens is updated to Redis.
If there are enough tokens in the bucket, the request is allowed to pass and the number of tokens is reduced by 1; if there are not enough tokens in the bucket, the request is rejected.
Summary:
Implementing concurrency control and current limiting functions in PHP microservices is very important to ensure the stability and performance optimization of the service. This article introduces how to use Semaphore and queues to implement concurrency control, and how to use the token bucket algorithm to implement current limiting, and provides specific code examples. By using these functions rationally, concurrent requests can be better controlled and the stability and performance of the service can be improved.
The above is the detailed content of How to implement concurrency control and current limiting functions in PHP microservices. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


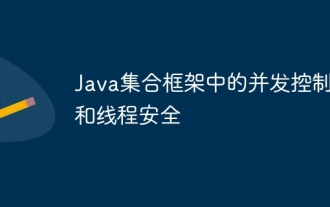
The Java collection framework manages concurrency through thread-safe collections and concurrency control mechanisms. Thread-safe collections (such as CopyOnWriteArrayList) guarantee data consistency, while non-thread-safe collections (such as ArrayList) require external synchronization. Java provides mechanisms such as locks, atomic operations, ConcurrentHashMap, and CopyOnWriteArrayList to control concurrency, thereby ensuring data integrity and consistency in a multi-threaded environment.
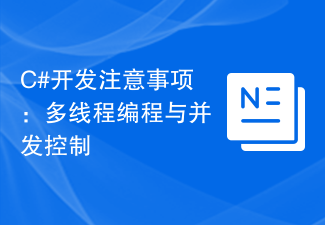
In C# development, multi-threaded programming and concurrency control are particularly important in the face of growing data and tasks. This article will introduce some matters that need to be paid attention to in C# development from two aspects: multi-threaded programming and concurrency control. 1. Multi-threaded programming Multi-threaded programming is a technology that uses multi-core resources of the CPU to improve program efficiency. In C# programs, multi-thread programming can be implemented using Thread class, ThreadPool class, Task class and Async/Await. But when doing multi-threaded programming
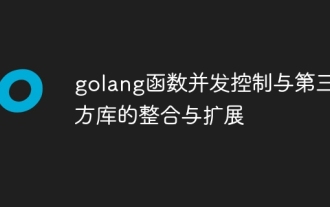
Concurrent programming is implemented in Go through Goroutine and concurrency control tools (such as WaitGroup, Mutex), and third-party libraries (such as sync.Pool, sync.semaphore, queue) can be used to extend its functions. These libraries optimize concurrent operations such as task management, resource access restrictions, and code efficiency improvements. An example of using the queue library to process tasks shows the application of third-party libraries in actual concurrency scenarios.
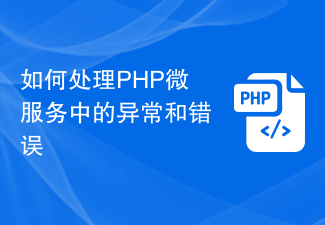
How to handle exceptions and errors in PHP microservices Introduction: With the popularity of microservice architecture, more and more developers choose to use PHP to implement microservices. However, due to the complexity of microservices, exception and error handling have become an essential topic. This article will introduce how to correctly handle exceptions and errors in PHP microservices and demonstrate it through specific code examples. 1. Exception handling In PHP microservices, exception handling is essential. Exceptions are unexpected situations encountered by the program during operation, such as database connection failure, A
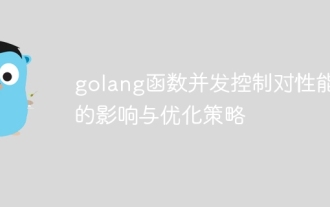
The impact of concurrency control on GoLang performance: Memory consumption: Goroutines consume additional memory, and a large number of goroutines may cause memory exhaustion. Scheduling overhead: Creating goroutines will generate scheduling overhead, and frequent creation and destruction of goroutines will affect performance. Lock competition: Lock synchronization is required when multiple goroutines access shared resources. Lock competition will lead to performance degradation and extended latency. Optimization strategy: Use goroutines correctly: only create goroutines when necessary. Limit the number of goroutines: use channel or sync.WaitGroup to manage concurrency. Avoid lock contention: use lock-free data structures or minimize lock holding times
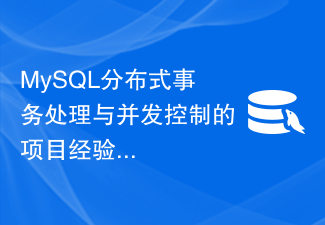
Analysis of MySQL Distributed Transaction Processing and Concurrency Control Project Experience In recent years, with the rapid development of the Internet and the increasing number of users, the requirements for databases have also increased. In large-scale distributed systems, MySQL, as one of the most commonly used relational database management systems, has always played an important role. However, as the data size increases and concurrent access increases, MySQL's performance and scalability face severe challenges. Especially in a distributed environment, how to handle transactions and control concurrency has become an urgent need to solve.
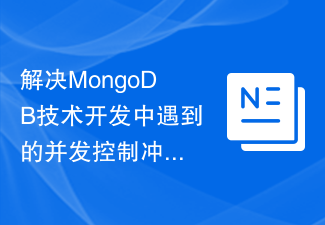
Research on methods to solve concurrency control conflicts encountered in MongoDB technology development Introduction: With the advent of the big data era, the demand for data storage and processing continues to increase. In this context, NoSQL database has become a database technology that has attracted much attention. As one of the representatives of NoSQL databases, MongoDB has been widely recognized and used for its high performance, scalability and flexible data model. However, MongoDB has some challenges in concurrency control, and how to solve these problems has become a research topic.
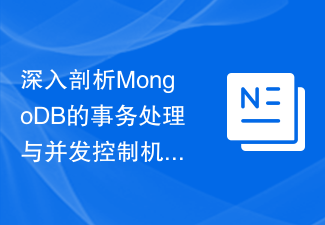
In-depth analysis of MongoDB's transaction processing and concurrency control mechanism Summary: MongoDB is a popular NoSQL database known for its high performance and scalability. However, MongoDB initially did not support transaction processing and concurrency control, which may cause data consistency and integrity issues in some cases. To address these issues, MongoDB introduced multi-document transactions and hybrid isolation levels in its latest version, providing developers with better concurrency control mechanisms. Introduction: Transaction Processing and
