


How to implement distributed algorithms and model training in PHP microservices
How to implement distributed algorithm and model training in PHP microservices
Introduction:
With the rapid development of cloud computing and big data technology, data processing and model training are increasingly in demand. Distributed algorithms and model training are key to achieving efficiency, speed, and scalability. This article will introduce how to implement distributed algorithms and model training in PHP microservices, and provide some specific code examples.
1. What is distributed algorithm and model training
Distributed algorithm and model training are technologies that use multiple machines or server resources to perform data processing and model training simultaneously. By dividing large-scale tasks into multiple small tasks and assigning them to multiple nodes for calculation, the calculation speed and efficiency can be greatly improved.
2. PHP microservice framework
Before implementing distributed algorithms and model training, you first need to choose a suitable PHP microservice framework. Currently, the more popular PHP microservice frameworks include Swoole, Workerman, etc. These frameworks can provide high-performance, high-concurrency network communication and multi-process support, making them ideal for distributed algorithms and model training.
3. Implementation steps of distributed algorithm and model training
- Data segmentation: Divide large-scale data into multiple small tasks and distribute these data to different node for processing.
- Inter-node communication: Communication is required between nodes in order to coordinate the execution of tasks. TCP/IP protocol or other communication protocols can be used for data exchange between nodes.
- Distributed algorithm design: For complex algorithm tasks, it is necessary to design an appropriate distributed algorithm to ensure that the calculation results between nodes can be correctly merged.
- Model training: When performing model training in a distributed environment, the update information of the model parameters needs to be transferred between different nodes to ensure that all nodes can obtain the latest model parameters.
- Result merging: After each node completes the task, the results need to be merged to obtain the final calculation result.
4. Code Example
The following is a simple example that demonstrates how to implement distributed algorithms and model training in PHP microservices.
// master节点代码 $workerNum = 4; //节点数量 $pool = new Pool($workerNum, Worker::class); //创建进程池 $data = [1, 2, 3, 4, 5, 6, 7, 8]; //待处理的数据 $result = []; //存储计算结果 foreach ($data as $item) { $pool->submit(new Task($item)); //将任务提交到进程池 } $pool->shutdown(); // 关闭进程池 foreach ($pool as $worker) { $result[] = $worker->getResult(); //获取各个节点的计算结果 } //输出最终结果 echo "Final Result: "; print_r($result); // worker节点代码 class Worker extends Threaded { private $data; private $result; public function __construct($data) { $this->data = $data; } public function run() { //节点执行的具体计算任务 $this->result = $this->data * 2; } public function getResult() { return $this->result; } } // task节点代码 class Task extends Threaded { private $item; public function __construct($item) { $this->item = $item; } public function run() { //将任务分发到worker节点进行处理 $worker = new Worker($this->item); $worker->start(); $worker->join(); $this->worker = $worker; } public function getResult() { return $this->worker->getResult(); } }
In the above example, the master node divides the task into multiple small tasks and distributes and manages them through the process pool. The worker node performs calculations after receiving the task and returns the results to the task node. Finally, the master node merges and outputs the results.
Summary:
By using the PHP microservice framework, distributed algorithms and model training can be easily implemented. Reasonable division of tasks, design of distributed algorithms, and communication between nodes are the keys to realizing distributed algorithms and model training. I hope the sample code in this article will be helpful to readers in understanding and practicing distributed algorithms and model training.
The above is the detailed content of How to implement distributed algorithms and model training in PHP microservices. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


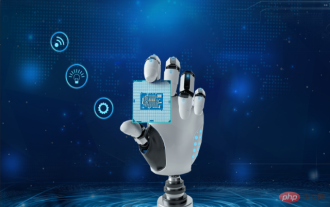
This article will introduce WeChat’s large-scale recommendation system training based on PyTorch. Unlike some other deep learning fields, the recommendation system still uses Tensorflow as the training framework, which is criticized by the majority of developers. Although there are some practices using PyTorch for recommendation training, the scale is small and there is no actual business verification, making it difficult to promote early adopters of business. In February 2022, the PyTorch team launched the official recommended library TorchRec. Our team began to try TorchRec in internal business in May and launched a series of cooperation with the TorchRec team. Over the course of several months of trialling, we found that TorchR
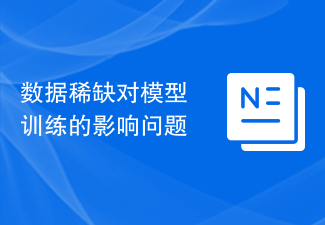
The impact of data scarcity on model training requires specific code examples. In the fields of machine learning and artificial intelligence, data is one of the core elements for training models. However, a problem we often face in reality is data scarcity. Data scarcity refers to the insufficient amount of training data or the lack of annotated data. In this case, it will have a certain impact on model training. The problem of data scarcity is mainly reflected in the following aspects: Overfitting: When the amount of training data is insufficient, the model is prone to overfitting. Overfitting refers to the model over-adapting to the training data.

Overview of how to use Python to train models on images: In the field of computer vision, using deep learning models to classify images, target detection and other tasks has become a common method. As a widely used programming language, Python provides a wealth of libraries and tools, making it relatively easy to train models on images. This article will introduce how to use Python and its related libraries to train models on images, and provide corresponding code examples. Environment preparation: Before starting, you need to ensure that you have installed
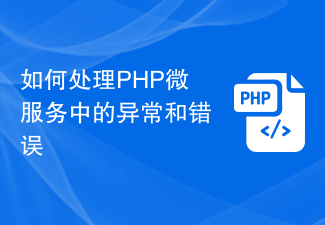
How to handle exceptions and errors in PHP microservices Introduction: With the popularity of microservice architecture, more and more developers choose to use PHP to implement microservices. However, due to the complexity of microservices, exception and error handling have become an essential topic. This article will introduce how to correctly handle exceptions and errors in PHP microservices and demonstrate it through specific code examples. 1. Exception handling In PHP microservices, exception handling is essential. Exceptions are unexpected situations encountered by the program during operation, such as database connection failure, A
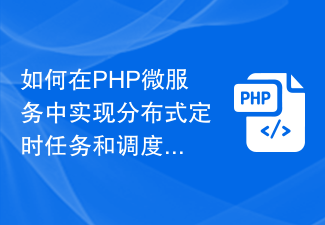
How to implement distributed scheduled tasks and scheduling in PHP microservices In modern microservice architecture, distributed scheduled tasks and scheduling are very important components. They can help developers easily manage, schedule and execute scheduled tasks in multiple microservices, improving system reliability and scalability. This article will introduce how to use PHP to implement distributed timing tasks and scheduling, and provide code examples for reference. Using a queue system In order to implement distributed scheduled tasks and scheduling, you first need to use a reliable queue system. Queuing systems can
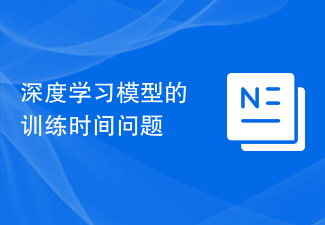
Introduction to the training time issue of deep learning models: With the development of deep learning, deep learning models have achieved remarkable results in various fields. However, the training time of deep learning models is a common problem. In the case of large-scale data sets and complex network structures, the training time of deep learning models increases significantly. This article will discuss the training time issue of deep learning models and give specific code examples. Parallel Computing Accelerates Training Time The training process of deep learning models usually requires a large amount of computing resources and time. In order to speed up training
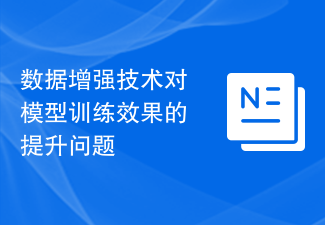
Specific code examples are needed to improve the model training effect of data augmentation technology. In recent years, deep learning has made huge breakthroughs in fields such as computer vision and natural language processing. However, in some scenarios, due to the small size of the data set, the model cannot The generalization ability and accuracy are difficult to reach satisfactory levels. At this time, data enhancement technology can play an important role by expanding the training data set and improving the generalization ability of the model. Data augmentation refers to a series of transformations and transformations on original data.
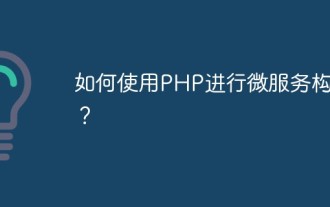
With the continuous development of the Internet and the continuous advancement of computer technology, microservice architecture has gradually become a hot topic in recent years. Different from the traditional monolithic application architecture, the microservice architecture decomposes a complex software application into multiple independent service units. Each service unit can be deployed, run and updated independently. The advantage of this architecture is that it improves the flexibility, scalability, and maintainability of the system. As an open source, Web-based programming language, PHP also plays a very important role in the microservice architecture.
