


How to use PHP to develop permission management functions for employee attendance data?
How to use PHP to develop the permission management function of employee attendance data?
As an enterprise develops and expands in scale, the management of employee attendance data becomes more and more important. In order to protect the security and privacy of data, a reasonable permission management system becomes very necessary. This article will introduce how to use PHP to develop a permission management function for employee attendance data, and provide specific code examples.
- Design database structure
First, we need to design a database structure suitable for employee attendance data. We can create two tables: user table and permission table. The user table is used to store employee information, including usernames and passwords. The permission table is used to store employee permission information, such as whether they have permissions to view, edit, delete, etc.
The following is a simple database design example:
User table (users):
user_id(INT): User ID
username(VARCHAR): User name
password(VARCHAR): Password
Permissions table (permissions):
permission_id(INT): Permission ID
user_id(INT): User ID
view(INT): View permission (1 Represents authority, 0 represents no authority)
edit(INT): Edit authority (1 represents authority, 0 represents no authority)
delete(INT): Delete authority (1 represents authority, 0 represents no authority) )
- Create login function
Next, we need to create a login function so that employees can enter their username and password to log in to the system. During the login process, we need to verify that the username and password entered by the user are correct.
The following is a simple login function code example:
<?php // 获取用户输入的用户名和密码 $username = $_POST['username']; $password = $_POST['password']; // 根据用户名查询用户信息 $query = "SELECT * FROM users WHERE username = '$username'"; $result = mysqli_query($conn, $query); // 验证用户输入的密码是否正确 if ($result && mysqli_num_rows($result) > 0) { $user = mysqli_fetch_assoc($result); if ($password == $user['password']) { // 登录成功,可进行相应操作 // 将用户ID存入session供后续使用 $_SESSION['user_id'] = $user['user_id']; } else { echo "密码错误"; } } else { echo "用户名不存在"; }
- Administrator permissions setting
Normally, only administrators have the authority to set administrator permissions . So we need to create an administrator page for the administrator to set employee permissions.
The following is a simple administrator page example:
<?php // 验证当前用户是否为管理员,如果不是则跳转到其他页面 if ($_SESSION['user_id'] != $admin_user_id) { header('Location: index.php'); exit(); } // 获取员工列表 $query = "SELECT * FROM users"; $result = mysqli_query($conn, $query); // 显示员工列表以及设置权限 while ($user = mysqli_fetch_assoc($result)) { echo $user['username']; echo "<input type='checkbox' name='view_permission[]' value='".$user['user_id']."'/>查看权限"; echo "<input type='checkbox' name='edit_permission[]' value='".$user['user_id']."'/>编辑权限"; echo "<input type='checkbox' name='delete_permission[]' value='".$user['user_id']."'/>删除权限"; }
- Update permissions
After the administrator sets the employee's permissions, we need to save the permission information into the database.
The following is a simple code example for updating permissions:
<?php // 获取管理员设置的权限信息 $view_permission = $_POST['view_permission']; $edit_permission = $_POST['edit_permission']; $delete_permission = $_POST['delete_permission']; // 更新权限信息 foreach ($view_permission as $user_id) { $query = "INSERT INTO permissions (user_id, view) VALUES ('$user_id', 1) ON DUPLICATE KEY UPDATE view=1"; mysqli_query($conn, $query); } foreach ($edit_permission as $user_id) { $query = "INSERT INTO permissions (user_id, edit) VALUES ('$user_id', 1) ON DUPLICATE KEY UPDATE edit=1"; mysqli_query($conn, $query); } foreach ($delete_permission as $user_id) { $query = "INSERT INTO permissions (user_id, delete) VALUES ('$user_id', 1) ON DUPLICATE KEY UPDATE delete=1"; mysqli_query($conn, $query); }
Through the above steps, we have completed the permission management function of developing employee attendance data using PHP. When an employee logs into the system, it is judged whether he or she can perform corresponding operations based on the permissions he or she has. Administrators can set employee permissions on the administrator page and save them in the database.
Of course, the above code is just a simple example, and more security verification and optimization are required in actual development. I hope this article can be helpful to develop the permission management function of employee attendance data in PHP.
The above is the detailed content of How to use PHP to develop permission management functions for employee attendance data?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


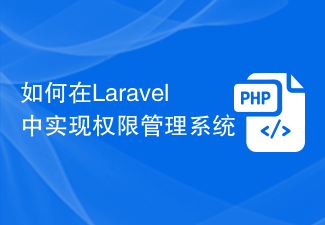
How to implement a permission management system in Laravel Introduction: With the continuous development of web applications, the permission management system has become one of the basic functions of many applications. Laravel, as a popular PHP framework, provides a wealth of tools and functions to implement permission management systems. This article will introduce how to implement a simple and powerful permission management system in Laravel and provide specific code examples. 1. Design ideas of the permission management system When designing the permission management system, the following key points need to be considered: roles and
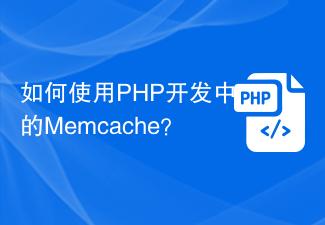
In web development, we often need to use caching technology to improve website performance and response speed. Memcache is a popular caching technology that can cache any data type and supports high concurrency and high availability. This article will introduce how to use Memcache in PHP development and provide specific code examples. 1. Install Memcache To use Memcache, we first need to install the Memcache extension on the server. In CentOS operating system, you can use the following command
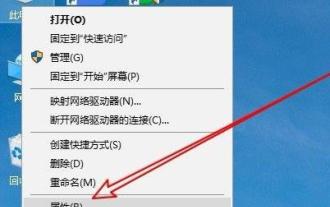
The problem that temporary folders cannot be installed without write permissions is a headache for many users. In fact, the operation is not very troublesome. You only need to enter your advanced menu to make changes. Let’s see how to solve the problem of no write permissions. The temporary folder cannot be installed without write permission: 1. First, right-click This Computer on the desktop, and then click "Properties". 2. Then click "Advanced System Settings" below. 3. Then click "Environment Variables" at the bottom of the window. 4. After that, you can open the environment variables window, click on the tmp file and select "Edit". 5. Then click "Browse Files" in the window that opens. 6. Set the new variable folder and click OK. 7. Finally wait until success.
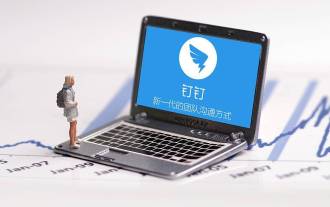
Everyone is familiar with the DingTalk platform. It is a very easy-to-use office software that provides convenience to users. It has a lot of rich functions to help companies better manage their employees. So does anyone know how to check employee attendance on DingTalk? Let’s take a look. Detailed steps to view employee attendance on DingTalk: 1. Open the DingTalk app and click "Attendance Punch" on the workbench. 2. Click [Statistics], click [Team Statistics], and click [Export Report] to see employee attendance. Software Features 1. The software has many convenient office functions and is a must-have artifact for your workplace; 2. The 2021 new version of DingTalk has added many new functions, providing a focused, efficient and secure instant messaging solution, allowing
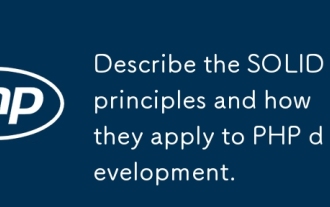
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
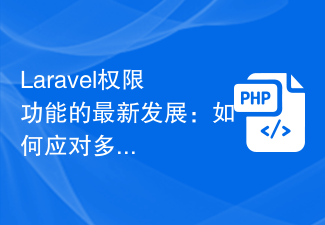
The latest development of Laravel's permissions function: How to deal with permission management in a multi-tenant environment, specific code examples are needed. In recent years, with the rise of cloud computing and software as a service (SaaS), permission management in a multi-tenant environment has become a key issue in software development. an important challenge. In this environment, multiple users or organizations share the same application, and each user or organization can only access its own data and functionality. In such a scenario, how to ensure that users can only access resources to which they have permission has become a problem that must be solved. L
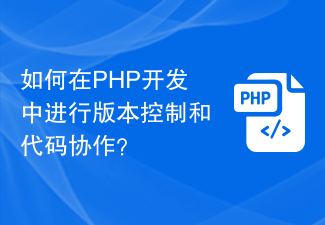
How to implement version control and code collaboration in PHP development? With the rapid development of the Internet and the software industry, version control and code collaboration in software development have become increasingly important. Whether you are an independent developer or a team developing, you need an effective version control system to manage code changes and collaborate. In PHP development, there are several commonly used version control systems to choose from, such as Git and SVN. This article will introduce how to use these tools for version control and code collaboration in PHP development. The first step is to choose the one that suits you
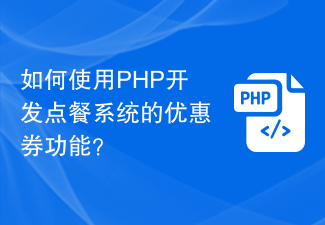
How to use PHP to develop the coupon function of the ordering system? With the rapid development of modern society, people's life pace is getting faster and faster, and more and more people choose to eat out. The emergence of the ordering system has greatly improved the efficiency and convenience of customers' ordering. As a marketing tool to attract customers, the coupon function is also widely used in various ordering systems. So how to use PHP to develop the coupon function of the ordering system? 1. Database design First, we need to design a database to store coupon-related data. It is recommended to create two tables: one
