


How to use Java to build an online examination system to screen test question difficulty
How to use Java to build an online examination system to screen test question difficulty
In the modern education system, online examinations have become a common academic assessment method. However, as the number of examination questions increases, screening of question difficulty becomes particularly important. To ensure the fairness and validity of the exam, the difficulty of the test questions should match the student's ability level. This article will introduce how to use Java to write and implement the test question difficulty screening algorithm in the online examination system, and provide code examples.
1. Demand Analysis
The following factors need to be considered when selecting the difficulty of test questions in the online examination system:
- Student ability level: The difficulty of the test questions should be consistent with the student’s ability level. Matching, relevant data can be obtained through student historical test scores, etc.
- Types of test questions: Different types of test questions (such as multiple-choice questions, fill-in-the-blank questions, quiz questions, etc.) have different difficulty criteria.
- Test question bank: The system should develop a question bank to store information about all test questions, including question content, answers, labels, etc.
- Test question difficulty assessment: The system should evaluate the difficulty of the test questions based on the student's ability level and question type in order to select questions with appropriate difficulty.
2. System Design
The test question difficulty screening module of the online examination system includes the following main components:
- Student ability level module: used to determine Ability level assesses its level of difficulty.
- Test question bank module: used to store and manage information about all test questions.
- Test question difficulty assessment module: used to evaluate the difficulty level of test questions based on students' ability levels and test question types.
- Test question screening module: Screen out appropriate test questions based on the difficulty level and type of the test questions.
3. Code Example
The following is a simple Java code example that shows how to use Java to build a test question difficulty screening algorithm for an online examination system:
class Question { String content; String answer; String type; double difficultyLevel; // ... 省略其他属性和方法 } class Student { String name; double abilityLevel; // ... 省略其他属性和方法 } class ExamSystem { List<Question> questionBank; public List<Question> filterQuestions(Student student) { List<Question> filteredQuestions = new ArrayList<>(); for (Question question : questionBank) { if (question.type.equals("选择题")) { double difficultyDifference = Math.abs(student.abilityLevel - question.difficultyLevel); if (difficultyDifference < 0.1) { filteredQuestions.add(question); } } else if (question.type.equals("填空题")) { // ... 根据不同类型的题目难度评估标准进行筛选 } } return filteredQuestions; } // ... 省略其他方法 } public class Main { public static void main(String[] args) { ExamSystem examSystem = new ExamSystem(); // 初始化试题库 examSystem.questionBank = new ArrayList<>(); Question question1 = new Question(); question1.content = "2 + 2 = ?"; question1.answer = "4"; question1.type = "选择题"; question1.difficultyLevel = 0.5; examSystem.questionBank.add(question1); Question question2 = new Question(); question2.content = "Java是一种编程语言。"; question2.answer = "是"; question2.type = "判断题"; question2.difficultyLevel = 0.3; examSystem.questionBank.add(question2); // 模拟学生信息 Student student = new Student(); student.name = "张三"; student.abilityLevel = 0.4; // 筛选试题 List<Question> filteredQuestions = examSystem.filterQuestions(student); // 输出筛选后的试题 for (Question question : filteredQuestions) { System.out.println(question.content); } } }
The above code example shows Describes how to design and implement the question difficulty filtering function of the online examination system. Based on students' ability levels and test question types, the system can screen out test questions that meet the requirements to ensure the fairness and validity of the exam. By repeatedly optimizing the difficulty assessment algorithm and test question screening strategy, the matching degree of test question difficulty and student ability level can be further improved.
Summary: This article introduces how to use Java to build the question difficulty filtering function of the online examination system, and provides code examples. The quality and effectiveness of online exams can be improved by rationally designing and implementing test question difficulty assessment algorithms, and performing appropriate screening processes based on students' ability levels and test question types.
The above is the detailed content of How to use Java to build an online examination system to screen test question difficulty. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


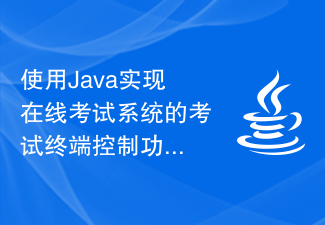
Java implements the examination terminal control function of the online examination system 1. Introduction The online examination system plays an important role in modern education. It can provide a convenient examination environment and an efficient scoring system. The examination terminal control function is an indispensable part of the online examination system. It can control the student's examination process and ensure the fairness and security of the examination. This article will use Java language as the basis to introduce how to implement the examination terminal control function of the online examination system and give specific code examples. 2. Requirements for examination terminal control functions
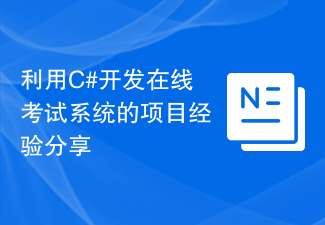
Sharing project experience using C# to develop an online examination system Introduction: With the continuous development of Internet technology, online education has become an increasingly popular way of learning. Online examination systems are widely used in many educational institutions and enterprises because they can provide flexible, efficient, and automated examination management and assessment functions. This article will share my experience and lessons learned in the project of developing an online examination system using C#. System Requirements Analysis Before developing an online examination system, the functions and limitations of the system need to be clarified. First, it is necessary to clarify the user type and permissions.
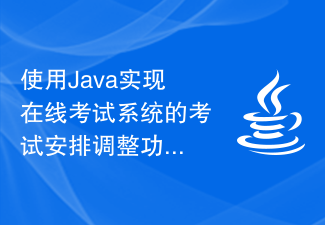
Java implementation of the examination arrangement adjustment function of the online examination system Introduction: With the development of Internet technology, more and more schools and training institutions choose to use online examination systems for examinations and assessments. Examination schedule adjustment is an important function in the online examination system, which can help administrators flexibly adjust examination time and examination-related information according to the actual situation. This article will introduce in detail how to use Java programming to implement the examination schedule adjustment function of the online examination system, and give specific code examples. Database design exam arrangement adjustment function needs

Overview of how to use Go language and Redis to implement an online examination system: The online examination system is an application that implements online examinations. By using Go language and Redis database, we can build an efficient, scalable and reliable online examination system. This article will introduce how to use Go language and Redis to design and implement a basic online examination system, and provide specific code examples. Requirements for the exam system: Before starting to implement it, we need to clarify the basic requirements for the exam system. Below is a simple requirement column
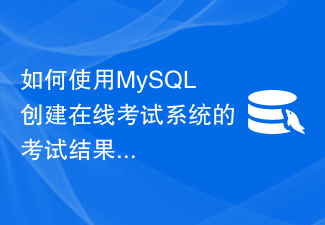
How to use MySQL to create the examination results query table structure of the online examination system? Online examination systems are an increasingly popular educational tool that can conveniently provide students with examination opportunities and provide fast and accurate feedback on examination results. The test result query function is one of the important components of the online test system. Users can query their test scores and rankings by entering relevant information. This article will introduce how to use MySQL to create the examination results query table structure of the online examination system, and provide specific code examples. In MyS
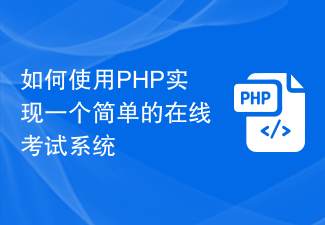
How to use PHP to implement a simple online examination system In modern education, more and more schools and training institutions use online examination systems to assess and evaluate students. A simple online examination system can provide convenient examination management, performance statistics, student feedback and other functions. This article will introduce how to use PHP to implement a simple online examination system and provide specific code examples. Database design First, we need to design a database to store exam-related data. The examination system needs to store the following important data tables:
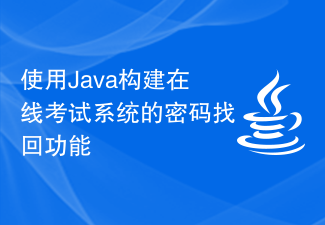
Java is a powerful programming language that is widely used in various fields. In the development of online examination systems, the password retrieval function is very important for users, which can help users quickly recover their login passwords. This article will introduce how to use Java to build the password retrieval function of the online examination system and give specific code examples. 1. Requirements Analysis for Password Retrieval Function The password retrieval function of the online examination system needs to meet the following basic requirements: Users can retrieve their password through the email or mobile phone number provided during registration. System requirements
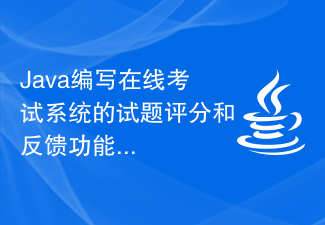
Question scoring and feedback functions of Java-written online examination systems With the development of the Internet, more and more educational institutions and companies tend to use online examinations to evaluate the abilities and knowledge levels of students or employees. In order to make the online examination system more complete and practical, question scoring and feedback functions are essential. This article will introduce how to use Java to write the question scoring and feedback functions of the online examination system, and provide some specific code examples. 1. Principles and methods of test question scoring Test question scoring is one of the core functions of the online examination system.
