


How to implement a simple online order management system using PHP
How to use PHP to implement a simple online order management system
1. Introduction
The online order management system is a common e-commerce application , which can help merchants effectively manage the order process, speed up order processing, and improve customer satisfaction. This article will introduce how to use PHP to implement a simple online order management system, including the creation, modification, query and deletion of orders. This article assumes that readers already have some basic knowledge of PHP.
2. System requirements
The online order management system needs to meet the following basic requirements:
- Login function: Administrators can log in to the system through username and password.
- Order management function: Administrators can create, modify, query and delete orders.
- Order list function: Administrators can view the list of orders, filter and sort by conditions.
- Order details function: The administrator can view the detailed information of the order, including order number, customer information, product information, etc.
- Database support: The system needs to use the MySQL database to store order data.
3. Implementation steps
- Create database
First, we need to create a database to store order data. Use the following SQL statement to create a database named "orders" and create a data table named "order_info" to store order information.
CREATE DATABASE orders; USE orders; CREATE TABLE order_info ( id INT(11) AUTO_INCREMENT PRIMARY KEY, order_number VARCHAR(20) NOT NULL, customer_name VARCHAR(50) NOT NULL, total_price DECIMAL(10,2) NOT NULL, create_time TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
- Create a login page
Create a file named "index.php" in the root directory of the project and add the following code:
<?php session_start(); if(isset($_POST['username']) && isset($_POST['password'])){ if($_POST['username'] === 'admin' && $_POST['password'] === 'admin'){ $_SESSION['login'] = true; header('Location: order_list.php'); exit; } } ?> <!DOCTYPE html> <html> <head> <title>登录</title> <meta charset="UTF-8"> </head> <body> <form method="POST" action="index.php"> <input type="text" name="username" placeholder="用户名"> <input type="password" name="password" placeholder="密码"> <button type="submit">登录</button> </form> </body> </html>
This page contains a simple login form. When the username and password are correct, the login status will be saved in the session and jump to the order list page.
- Create order list page
Create a file named "order_list.php" in the root directory of the project and add the following code:
<?php session_start(); if(!isset($_SESSION['login']) || $_SESSION['login'] !== true){ header('Location: index.php'); exit; } ?> <!DOCTYPE html> <html> <head> <title>订单列表</title> <meta charset="UTF-8"> </head> <body> <h1 id="订单列表">订单列表</h1> <a href="create_order.php">创建新订单</a> <table> <tr> <th>订单号</th> <th>客户姓名</th> <th>订单金额</th> <th>创建时间</th> <th></th> </tr> <?php // 连接数据库 $conn = new mysqli('localhost', 'root', 'password', 'orders'); if ($conn->connect_error) { die('数据库连接失败:' . $conn->connect_error); } // 查询订单列表 $sql = 'SELECT * FROM order_info ORDER BY create_time DESC'; $result = $conn->query($sql); if ($result->num_rows > 0) { while($row = $result->fetch_assoc()) { echo '<tr>'; echo '<td>'.$row['order_number'].'</td>'; echo '<td>'.$row['customer_name'].'</td>'; echo '<td>'.$row['total_price'].'</td>'; echo '<td>'.$row['create_time'].'</td>'; echo '<td><a href="order_details.php?id='.$row['id'].'">详情</a></td>'; echo '</tr>'; } } else { echo '<tr><td colspan="5">暂无订单信息</td></tr>'; } $conn->close(); ?> </table> </body> </html>
This page first checks the login status. If the user is not logged in, it jumps to the login page. Next, it queries the order list from the database and displays it in an HTML table. Each row displays the order number, customer name, order amount, creation time, and a link to view the details of the order.
- Create order details page
Create a file named "order_details.php" in the root directory of the project and add the following code:
<?php session_start(); if(!isset($_SESSION['login']) || $_SESSION['login'] !== true){ header('Location: index.php'); exit; } if(!isset($_GET['id'])){ header('Location: order_list.php'); exit; } $id = $_GET['id']; // 连接数据库 $conn = new mysqli('localhost', 'root', 'password', 'orders'); if ($conn->connect_error) { die('数据库连接失败:' . $conn->connect_error); } // 查询订单详情 $sql = 'SELECT * FROM order_info WHERE id = '.$id; $result = $conn->query($sql); if ($result->num_rows == 0) { header('Location: order_list.php'); exit; } $row = $result->fetch_assoc(); ?> <!DOCTYPE html> <html> <head> <title>订单详情</title> <meta charset="UTF-8"> </head> <body> <h1 id="订单详情">订单详情</h1> <p><strong>订单号:</strong><?php echo $row['order_number'];?></p> <p><strong>客户姓名:</strong><?php echo $row['customer_name'];?></p> <p><strong>订单金额:</strong><?php echo $row['total_price'];?></p> <p><strong>创建时间:</strong><?php echo $row['create_time'];?></p> <a href="edit_order.php?id=<?php echo $row['id'];?>">编辑订单</a> <a href="delete_order.php?id=<?php echo $row['id'];?>" onclick="return confirm('确认删除该订单吗?')">删除订单</a> </body> </html>
This page first checks the login status and order id. If the user is not logged in or does not pass the order id, it will jump to the order list page. Next, it queries the order details from the database and displays them in an HTML page. The page also contains links to edit orders and delete orders.
- Create create order page
Create a file named "create_order.php" in the root directory of the project and add the following code:
<?php session_start(); if(!isset($_SESSION['login']) || $_SESSION['login'] !== true){ header('Location: index.php'); exit; } if(isset($_POST['submit'])){ // 获取订单信息 $order_number = $_POST['order_number']; $customer_name = $_POST['customer_name']; $total_price = $_POST['total_price']; // 连接数据库 $conn = new mysqli('localhost', 'root', 'password', 'orders'); if ($conn->connect_error) { die('数据库连接失败:' . $conn->connect_error); } // 创建订单 $sql = 'INSERT INTO order_info (order_number, customer_name, total_price) VALUES ("'.$order_number.'", "'.$customer_name.'", '.$total_price.')'; $result = $conn->query($sql); if($result === true){ header('Location: order_list.php'); exit; } else { die('创建订单失败:' . $conn->error); } $conn->close(); } ?> <!DOCTYPE html> <html> <head> <title>创建订单</title> <meta charset="UTF-8"> </head> <body> <h1 id="创建订单">创建订单</h1> <form method="POST" action="create_order.php"> <input type="text" name="order_number" placeholder="订单号"> <input type="text" name="customer_name" placeholder="客户姓名"> <input type="number" step="0.01" name="total_price" placeholder="订单金额"> <button type="submit" name="submit">创建订单</button> </form> </body> </html>
This page first checks the login status. If the user is not logged in, it jumps to the login page. It then saves the order information to the database when the order form is submitted.
- Create editing order page and delete order function
The implementation of editing order function is similar to creating order. Just add the following code to the "edit_order.php" page:
<?php session_start(); if(!isset($_SESSION['login']) || $_SESSION['login'] !== true){ header('Location: index.php'); exit; } if(!isset($_GET['id'])){ header('Location: order_list.php'); exit; } $id = $_GET['id']; // 连接数据库 $conn = new mysqli('localhost', 'root', 'password', 'orders'); if ($conn->connect_error) { die('数据库连接失败:' . $conn->connect_error); } // 查询订单详情 $sql = 'SELECT * FROM order_info WHERE id = '.$id; $result = $conn->query($sql); if ($result->num_rows == 0) { header('Location: order_list.php'); exit; } $row = $result->fetch_assoc(); if(isset($_POST['submit'])){ // 获取订单信息 $order_number = $_POST['order_number']; $customer_name = $_POST['customer_name']; $total_price = $_POST['total_price']; // 更新订单 $sql = 'UPDATE order_info SET order_number = "'.$order_number.'", customer_name = "'.$customer_name.'", total_price = '.$total_price.' WHERE id = '.$id; $result = $conn->query($sql); if($result === true){ header('Location: order_details.php?id='.$id); exit; } else { die('编辑订单失败:' . $conn->error); } $conn->close(); } ?> <!DOCTYPE html> <html> <head> <title>编辑订单</title> <meta charset="UTF-8"> </head> <body> <h1 id="编辑订单">编辑订单</h1> <form method="POST" action="edit_order.php?id=<?php echo $row['id'];?>"> <input type="text" name="order_number" placeholder="订单号" value="<?php echo $row['order_number'];?>"> <input type="text" name="customer_name" placeholder="客户姓名" value="<?php echo $row['customer_name'];?>"> <input type="number" step="0.01" name="total_price" placeholder="订单金额" value="<?php echo $row['total_price'];?>"> <button type="submit" name="submit">保存修改</button> </form> </body> </html>
The implementation of the delete order function is similar, just add the following code to the "delete_order.php" page:
<?php session_start(); if(!isset($_SESSION['login']) || $_SESSION['login'] !== true){ header('Location: index.php'); exit; } if(!isset($_GET['id'])){ header('Location: order_list.php'); exit; } $id = $_GET['id']; // 连接数据库 $conn = new mysqli('localhost', 'root', 'password', 'orders'); if ($conn->connect_error) { die('数据库连接失败:' . $conn->connect_error); } // 删除订单 $sql = 'DELETE FROM order_info WHERE id = '.$id; $result = $conn->query($sql); if($result === true){ header('Location: order_list.php'); exit; } else { die('删除订单失败:' . $conn->error); } $conn->close(); ?>
This page first checks the login status and order id. If the user is not logged in or If the order id is not passed, it will jump to the order list page. Then, it deletes the order with the specified id from the database and jumps to the order list page.
IV. Summary
This article introduces how to use PHP to implement a simple online order management system, including the creation, modification, query and deletion of orders. Through the sample code in this article, readers can learn how to use PHP and MySQL to implement a simple e-commerce application to provide merchants with a more efficient order management method. Of course, this is just a basic example, and more functions and security need to be considered in actual applications. I hope this article is helpful to readers, thank you for reading!
The above is the detailed content of How to implement a simple online order management system using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
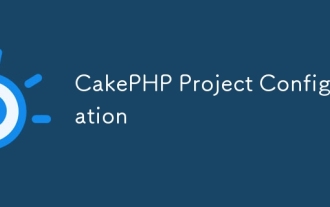
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
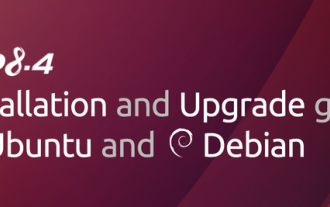
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
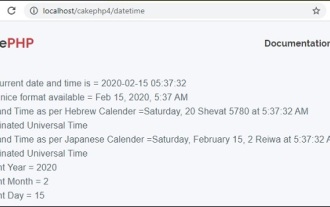
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
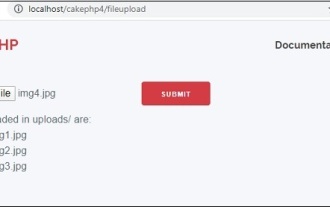
To work on file upload we are going to use the form helper. Here, is an example for file upload.
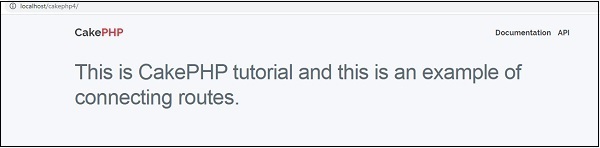
In this chapter, we are going to learn the following topics related to routing ?
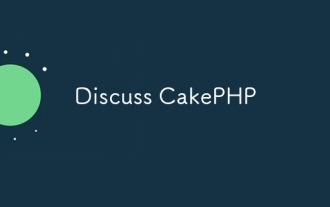
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
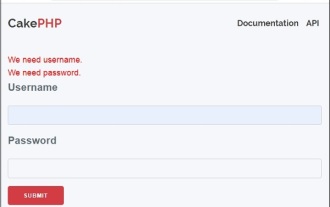
Validator can be created by adding the following two lines in the controller.
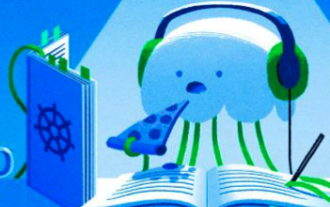
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
