


How to use Java to implement the Just-In-Time (JIT) inventory strategy of the warehouse management system
How to use Java to implement the Just-In-Time (JIT) inventory strategy of the warehouse management system
Abstract:
With the development and management of the global supply chain With the advancement of technology, Just-In-Time (JIT) inventory strategies are becoming more and more important in warehouse management. This article will introduce how to use Java language to implement the JIT inventory strategy of the warehouse management system and provide specific code examples.
- Introduction:
With the globalization of the economy and the intensification of business competition, warehouse management is crucial to the operational efficiency and cost control of enterprises. JIT inventory strategy aims to optimize warehouse management by minimizing inventory holding costs, reducing operating cycles, and improving logistics efficiency. This article will introduce how to use Java language to implement JIT inventory strategy to make the warehouse management system more efficient. - Principle of JIT inventory strategy:
The core idea of JIT inventory strategy is to obtain materials from suppliers in a timely manner when materials are needed, so as to reduce inventory occupation and operation cycle. Its main features include: - Production and procurement based on demand rather than forecasting;
- Reduce the residence time of materials in the warehouse;
- Establish a stable supply chain partnership .
- Steps to implement JIT inventory strategy using Java:
3.1 Demand planning and forecasting: Obtain material demand plans and forecasts from sales orders, customer needs, etc., and convert them into inventory strategies.
3.2 Supplier management: Establish a cooperative relationship with suppliers, and manage supplier-related information through Java code, including product type, quantity, price, etc.
3.3 Warehouse management: Use Java to write a warehouse management system, including the operations of material entry and exit, while monitoring inventory quantity and status in real time.
3.4 Material tracking: Realize material tracking and monitoring through Java code to maintain inventory accuracy and reliability.
3.5 Transportation management: Use Java to implement the transportation management module, arrange logistics and transportation based on sales orders and supplier information, and ensure the timely supply of materials.
- Java code example:
The following is a simple Java code example that demonstrates how to implement the material entry and exit functions:
public class Warehouse { private int stock; public int getStock() { return stock; } public void setStock(int stock) { this.stock = stock; } public synchronized void produce(int quantity) { while (stock != 0) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } stock += quantity; System.out.println("Produced: " + quantity); notifyAll(); } public synchronized void consume(int quantity) { while (stock < quantity) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } stock -= quantity; System.out.println("Consumed: " + quantity); notifyAll(); } }
In the above In the example, we created a warehouse class Warehouse and used the synchronized keyword to ensure thread safety. The produce method is used to produce materials, and the consume method is used to consume materials. Synchronization of production and consumption is achieved through the wait() and notifyAll() methods.
- Conclusion:
This article introduces how to use Java language to implement the Just-In-Time (JIT) inventory strategy of the warehouse management system, and gives specific code examples. By using JIT inventory strategy, companies can reduce inventory costs and improve logistics efficiency, thereby optimizing warehouse management and improving competitiveness. However, the implementation of the warehouse management system also requires customized development according to the specific circumstances of the enterprise to better meet the needs of the enterprise.
The above is the detailed content of How to use Java to implement the Just-In-Time (JIT) inventory strategy of the warehouse management system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
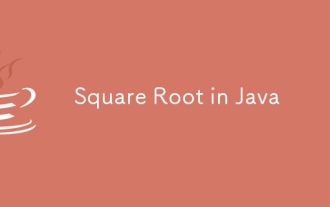
Guide to Square Root in Java. Here we discuss how Square Root works in Java with example and its code implementation respectively.
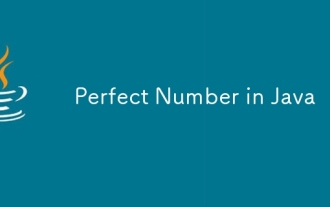
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
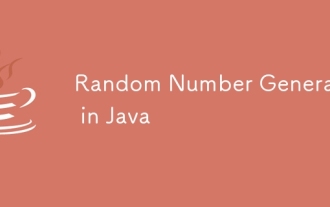
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
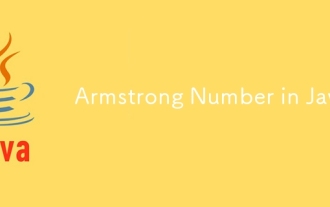
Guide to the Armstrong Number in Java. Here we discuss an introduction to Armstrong's number in java along with some of the code.
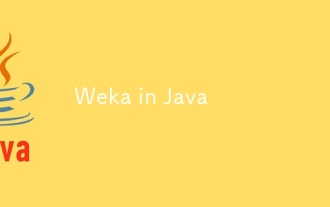
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
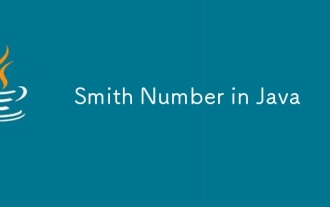
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
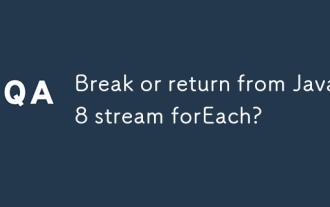
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
