


How to use PHP microservices to implement distributed task scheduling and distribution
How to use PHP microservices to implement distributed task scheduling and distribution
In recent years, with the rapid development of Internet technology, distributed systems have become an important factor in building high availability. One of the important methods for high-performance applications. In a distributed system, task scheduling and distribution are a key part of it. This article will introduce how to use PHP microservices to implement distributed task scheduling and distribution, and provide specific code examples.
1. What is microservice?
Microservices are an architectural style that splits complex applications into multiple small, independently deployed services, each of which can run and scale independently. Each microservice is a relatively independent process that can interact through lightweight communication mechanisms. The advantages of microservices are decoupling, scalability, fault tolerance, etc.
2. Requirements for distributed task scheduling and distribution
In distributed systems, task scheduling and distribution are the keys to achieving high availability and high concurrency of the system. Normally, we need to distribute tasks through a centralized scheduler and distribute tasks to different worker nodes for processing. In a distributed scenario, we hope that tasks can be evenly distributed to different nodes to improve the performance and stability of the overall system.
3. Use PHP microservices to implement distributed task scheduling and distribution
- Determine the task scheduler
We first need a task scheduler to receive tasks and distribute them to different worker nodes. You can use PHP frameworks to build task schedulers, such as Swoole, etc.
- Define task interface
We need to define a task interface for communication between the task scheduler and worker nodes. The task interface can be defined using the RESTful API or RPC framework provided by PHP.
Sample code:
//Task interface definition
interface TaskInterface {
public function execute($params);
}
- Implement task interface
Each worker node needs to implement the execute method in the task interface to execute specific task logic.
Sample code:
class Task implements TaskInterface {
public function execute($params) { // 执行具体的任务逻辑 }
}
- Start the task scheduler and worker nodes
We need to start the task scheduler and worker nodes on different servers respectively, and register and discover them. You can use the service registration center to realize automatic discovery and registration of nodes.
Sample code:
// Task scheduler
$scheduler = new Scheduler();
$scheduler->start();
/ / Worker node
$worker = new Worker();
$worker->start();
- Implement the task distribution logic of the task scheduler
The task scheduler distributes tasks to different working nodes through a certain scheduling algorithm. Scheduling can be performed based on factors such as task type and priority.
Sample code:
class Scheduler {
public function start() { // 监听任务队列 while (true) { $task = $this->receiveTask(); // 根据调度算法,将任务分发给不同的工作节点 $worker = $this->selectWorker(); $worker->executeTask($task); } } // 接收任务 private function receiveTask() { // 从任务队列中获取任务 } // 选择工作节点 private function selectWorker() { // 根据调度算法选择一个可用的工作节点 }
}
- Implement task execution logic of worker nodes
After the working node receives the task, it executes the execute method in the task interface. Task nodes can perform load balancing, fault tolerance, etc. as needed.
Sample code:
class Worker {
public function start() { // 监听任务队列 while (true) { $task = $this->receiveTask(); $this->executeTask($task); } } // 接收任务 private function receiveTask() { // 从任务队列中获取任务 } // 执行任务 public function executeTask($task) { $task->execute(); }
}
4. Summary
PHP is widely used in website development Scripting language, by using microservice architecture for distributed task scheduling and distribution, the scalability and performance of the system can be improved. This article introduces how to use PHP microservices to implement distributed task scheduling and distribution, and provides specific code examples. I hope it will be helpful to everyone.
The above is the detailed content of How to use PHP microservices to implement distributed task scheduling and distribution. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


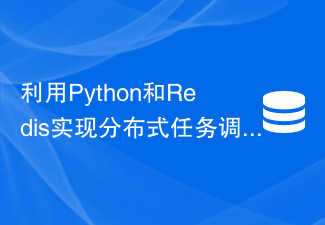
Using Python and Redis to implement distributed task scheduling: How to implement scheduled tasks Introduction: In distributed systems, task scheduling is an important task. For large-scale systems, in order to ensure high availability and high performance, task scheduling requires distributed processing. This article will introduce how to use Python and Redis to implement distributed task scheduling and specifically implement scheduled tasks. 1. What is RedisRedis is an open source in-memory data structure storage system that can also be used as a distributed cache and message broker.
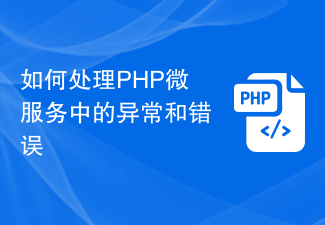
How to handle exceptions and errors in PHP microservices Introduction: With the popularity of microservice architecture, more and more developers choose to use PHP to implement microservices. However, due to the complexity of microservices, exception and error handling have become an essential topic. This article will introduce how to correctly handle exceptions and errors in PHP microservices and demonstrate it through specific code examples. 1. Exception handling In PHP microservices, exception handling is essential. Exceptions are unexpected situations encountered by the program during operation, such as database connection failure, A
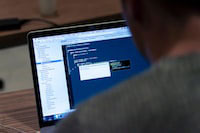
PyInstaller is an open source library that allows developers to compile Python code into platform-independent self-contained executables (.exe or .app). It does this by packaging Python code, dependencies, and supporting files together to create standalone applications that can run without installing a Python interpreter. The advantage of PyInstaller is that it removes dependency on the Python environment, allowing applications to be easily distributed and deployed to end users. It also provides a builder mode that allows users to customize the application's settings, icons, resource files, and environment variables. Install PyInstal using PyInstaller to package Python code
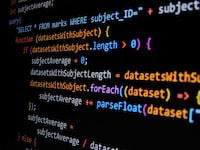
PyInstaller is a revolutionary tool that empowers Python applications beyond their original scripting form. By compiling Python code into standalone executable files, PyInstaller unlocks a new realm of code distribution, deployment, and maintenance. From a single script to a powerful application In the past, Python scripts only existed in a specific Python environment. Distributing such a script requires users to install Python and the necessary libraries, which is a time-consuming and cumbersome process. PyInstaller introduces the concept of packaging, combining Python code with all required dependencies into a single executable file. The Art of Code Packaging PyInstaller’s Work
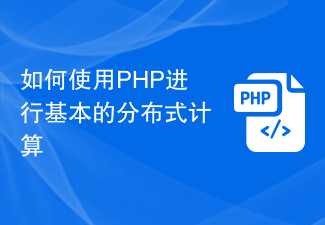
As the demand for data processing and analysis increases, distributed computing has gradually become an essential skill for many enterprises and data scientists. As a commonly used programming language, PHP can also be used for distributed computing. This article will introduce how to use PHP for basic distributed computing. What is distributed computing? Distributed computing refers to the process of splitting large computing tasks into small tasks that may be processed in parallel, and assigning these tasks to multiple computers for processing. Based on this method, the computer can complete a large number of computing tasks in the same time,

How to implement distributed task scheduling in Go language With the continuous development of the Internet, distributed systems are becoming more and more common when processing large-scale tasks. Distributed task scheduling is a way to evenly distribute tasks to multiple machines for execution, which can improve task processing efficiency and system scalability. This article will introduce how to implement distributed task scheduling in Go language and provide code examples. 1. Introduce third-party libraries We can use third-party libraries to simplify the implementation of distributed task scheduling. Commonly used ones are: etcd: a high
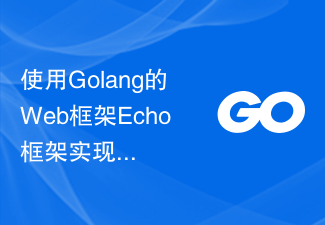
With the development of the Internet and the advancement of information technology, the era of big data has arrived, and fields such as data analysis and machine learning have also been widely used. In these fields, task scheduling is an inevitable problem. How to achieve efficient task scheduling is crucial to improving efficiency. In this article, we will introduce how to use Golang's web framework Echo framework to implement distributed task scheduling. 1. Introduction to the Echo framework Echo is a high-performance, scalable, lightweight GoWeb framework. It is based on HTTP
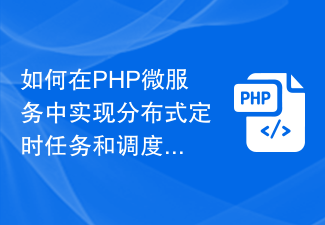
How to implement distributed scheduled tasks and scheduling in PHP microservices In modern microservice architecture, distributed scheduled tasks and scheduling are very important components. They can help developers easily manage, schedule and execute scheduled tasks in multiple microservices, improving system reliability and scalability. This article will introduce how to use PHP to implement distributed timing tasks and scheduling, and provide code examples for reference. Using a queue system In order to implement distributed scheduled tasks and scheduling, you first need to use a reliable queue system. Queuing systems can
