How to write a simple subscription push function via PHP
How to write a simple subscription push function through PHP
In today's Internet era, the subscription push function has become an important part of many websites and applications. Through the subscription push function, users can get the information they are interested in in a timely manner without having to actively search for it themselves. In this article, we will learn how to write a simple subscription push function through PHP and provide specific code examples.
- Create database table
First, we need to create a database table to store the user's subscription information. We can call them subscriptions. The table needs to contain at least the following fields: id (auto-incrementing primary key), email (email address of the subscriber) and created_at (subscription creation time). The table can be created through the following SQL statement:
CREATE TABLE subscriptions (
id INT AUTO_INCREMENT PRIMARY KEY,
email VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
- Create a subscription interface
Next, we need to create a subscription interface that allows users to fill in their email to subscribe. In HTML, we can create a simple form:
- Handling subscription requests
When a user submits a subscription form, we need to process the request and store the subscription information into the database. A file called subscribe.php can be created to handle subscription requests. The code is as follows:
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$email = $_POST['email'];
// Verify that the email format is correct
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
// 连接数据库 $conn = new PDO('mysql:host=localhost;dbname=your_database', 'username', 'password'); // 插入订阅信息到subscriptions表中 $stmt = $conn->prepare('INSERT INTO subscriptions (email) VALUES (:email)'); $stmt->bindParam(':email', $email); $stmt->execute(); // 关闭数据库连接 $conn = null; echo '订阅成功!';
} else {
echo '请输入有效的电子邮件地址!';
}
}
?>
Please note that this is just a simple example. In actual projects, you need to perform more input validation and security checks based on your own needs.
- Push subscription message
When there is new information that needs to be pushed to subscribed users, we need to write a script to implement the function of sending push emails. You can create a file called send_push.php to implement this functionality. The code is as follows:
// Connect to the database
$conn = new PDO('mysql:host=localhost;dbname=your_database', 'username', 'password ');
//Query the email addresses of all subscribed users
$stmt = $conn->query('SELECT email FROM subscriptions');
$emails = $stmt-> ;fetchAll(PDO::FETCH_COLUMN);
//Close the database connection
$conn = null;
//Send push emails to all subscribed users
foreach ($emails as $email) {
$subject = 'New push message';
$message = 'Write the content of the message you want to push here';
// Use the mail() function Send email
mail($email, $subject, $message);
}
echo 'Push message successfully! ';
?>
Please note that this example uses PHP's mail() function to send emails. In actual use, you may need to use a more professional email sending library to handle the logic and problems of sending emails.
Through the above steps, we can write a simple subscription push function through PHP. When users subscribe, they will receive push messages in a timely manner. Of course, this is just a very basic implementation. In actual projects, you may need more functions, such as batch subscription management, unsubscription function, subscriber user authentication, etc. However, with the sample code above, you can have a good starting point to build more complex implementations. Hope this helps!
The above is the detailed content of How to write a simple subscription push function via PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
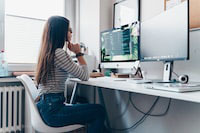
This article will explain in detail how PHP formats rows into CSV and writes file pointers. I think it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Format rows to CSV and write to file pointer Step 1: Open file pointer $file=fopen("path/to/file.csv","w"); Step 2: Convert rows to CSV string using fputcsv( ) function converts rows to CSV strings. The function accepts the following parameters: $file: file pointer $fields: CSV fields as an array $delimiter: field delimiter (optional) $enclosure: field quotes (
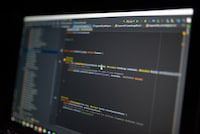
This article will explain in detail about changing the current umask in PHP. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Overview of PHP changing current umask umask is a php function used to set the default file permissions for newly created files and directories. It accepts one argument, which is an octal number representing the permission to block. For example, to prevent write permission on newly created files, you would use 002. Methods of changing umask There are two ways to change the current umask in PHP: Using the umask() function: The umask() function directly changes the current umask. Its syntax is: intumas
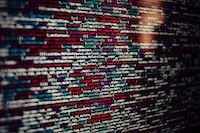
This article will explain in detail how to create a file with a unique file name in PHP. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Creating files with unique file names in PHP Introduction Creating files with unique file names in PHP is essential for organizing and managing your file system. Unique file names ensure that existing files are not overwritten and make it easier to find and retrieve specific files. This guide will cover several ways to generate unique filenames in PHP. Method 1: Use the uniqid() function The uniqid() function generates a unique string based on the current time and microseconds. This string can be used as the basis for the file name.
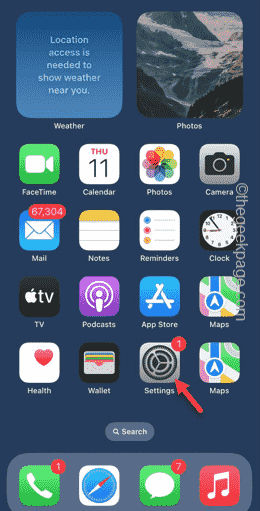
The country or region of your Apple ID cannot be changed. In many cases, you cannot change the region of your Apple ID. We have discussed all these conditions and their respective solutions so that you can change AppleID on iPhone. Fix 1 – Cancel all active subscriptions Apple does not allow you to perform region modification operations if you already have active subscriptions. Typically, these apps have different subscription plans, tiers, and fees (currencies) in different regions. Step 1 – You have to go to Settings. Step 2 – You will find your Apple ID at the top of the Settings page. Step 3 – Click once to open it. Step 4 – On the next page, open the Subscriptions menu. Step 5 – You can view it in
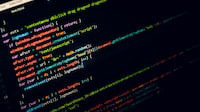
This article will explain in detail about PHP calculating the MD5 hash of files. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP calculates the MD5 hash of a file MD5 (MessageDigest5) is a one-way encryption algorithm that converts messages of arbitrary length into a fixed-length 128-bit hash value. It is widely used to ensure file integrity, verify data authenticity and create digital signatures. Calculating the MD5 hash of a file in PHP PHP provides multiple methods to calculate the MD5 hash of a file: Use the md5_file() function. The md5_file() function directly calculates the MD5 hash value of the file and returns a 32-character
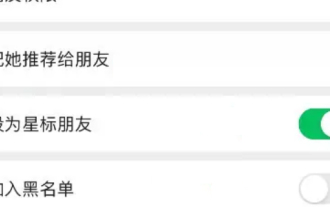
Business cards are a method that can be used to push friends in the software WeChat. Some users don’t know how to push friends’ business cards in WeChat. Just click on the friend’s personal page, select More to recommend them to friends and send them. This article is about WeChat push. The introduction of the friend’s business card method can tell you the specific content. The following is a detailed introduction, take a look! WeChat usage tutorial: How to push a friend’s business card on WeChat? Answer: Click on the friend’s personal page, select More to recommend them to friends and send them. Details: 1. Click on the friend you want to push a business card to. 2. Click the [More] option in the upper right corner. 3. Then click [Recommend TA to friends]. 4. Select the friend you want to send a business card to. 5. Click [Send].
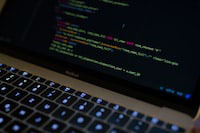
This article will explain in detail how PHP returns an array after key value flipping. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP Key Value Flip Array Key value flip is an operation on an array that swaps the keys and values in the array to generate a new array with the original key as the value and the original value as the key. Implementation method In PHP, you can perform key-value flipping of an array through the following methods: array_flip() function: The array_flip() function is specially used for key-value flipping operations. It receives an array as argument and returns a new array with the keys and values swapped. $original_array=[
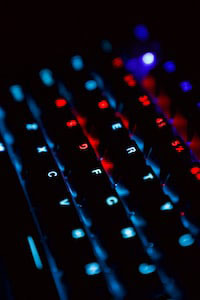
This article will explain in detail how PHP truncates files to a given length. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. Introduction to PHP file truncation The file_put_contents() function in PHP can be used to truncate files to a specified length. Truncation means removing part of the end of a file, thereby shortening the file length. Syntax file_put_contents($filename,$data,SEEK_SET,$offset);$filename: the file path to be truncated. $data: Empty string to be written to the file. SEEK_SET: designated as the beginning of the file
