


How to implement distributed message notification and push in PHP microservices
The following is an implementation method of distributed message notification and push based on PHP microservices, including detailed code examples.
Title: Distributed message notification and push implementation in PHP microservices
Introduction:
With the rise of microservice architecture, more and more applications adopt distributed Architecture to achieve system splitting and service decoupling. In distributed systems, message notification and push are very common requirements for asynchronous communication between different services. For PHP microservices, how to implement distributed message notification and push is an important and challenging task. This article will introduce a method to implement distributed message notification and push in PHP microservices, and provide corresponding code examples.
1. Using message queue
- Installing and configuring message queue
In PHP microservices, we can use some popular message queue systems, such as RabbitMQ, Kafka, etc. First, the corresponding message queue system needs to be installed and configured on the server. Taking RabbitMQ as an example, you can install it through the following command:
# 安装依赖 sudo apt-get install -y curl gnupg debian-archive-keyring apt-transport-https # 添加RabbitMQ官方GPG key curl https://packages.rabbitmq.com/rabbitmq-release-signing-key.asc | sudo apt-key add - # 添加RabbitMQ的APT源 echo "deb https://dl.bintray.com/rabbitmq/debian $(lsb_release -cs) main" | sudo tee /etc/apt/sources.list.d/bintray.rabbitmq.list # 更新APT源 sudo apt-get update # 安装RabbitMQ Server sudo apt-get install -y rabbitmq-server
- Create queues and switches
After the installation is complete, you can create queues and switches through the RabbitMQ management interface or command line tools . For example, you can create a queue named "notification" and an exchange named "exchange" using the following commands:
# 创建队列 sudo rabbitmqctl add_queue notification # 创建交换机 sudo rabbitmqctl add_exchange exchange
- Sending and receiving messages
In PHP code, Messages can be sent and received using RabbitMQ's client library. First, you need to install the corresponding RabbitMQ client library. It can be installed through Composer:
composer require php-amqplib/php-amqplib
Next, you can use the following code example to send a message:
<?php require_once __DIR__ . '/vendor/autoload.php'; $connection = new PhpAmqpLibConnectionAMQPStreamConnection('localhost', 5672, 'guest', 'guest'); $channel = $connection->channel(); $channel->exchange_declare('exchange', 'direct', false, false, false); $message = 'Hello, world!'; $channel->basic_publish(new PhpAmqpLibMessageAMQPMessage($message), 'exchange', 'notification'); $channel->close(); $connection->close();
Here is the example code to receive a message:
<?php require_once __DIR__ . '/vendor/autoload.php'; $connection = new PhpAmqpLibConnectionAMQPStreamConnection('localhost', 5672, 'guest', 'guest'); $channel = $connection->channel(); $channel->exchange_declare('exchange', 'direct', false, false, false); list($queue_name, ,) = $channel->queue_declare('', false, false, true, false); $channel->queue_bind($queue_name, 'exchange', 'notification'); $callback = function ($msg) { echo 'Received message: ' . $msg->body; }; $channel->basic_consume($queue_name, '', false, true, false, false, $callback); while ($channel->is_consuming()) { $channel->wait(); } $channel->close(); $connection->close();
2. Use push services
In addition to using message queues, we can also use some specialized push services to implement distributed message notification and push. The following is an example using cloud push service (taking Alibaba Cloud Push as an example):
- Register and configure push service
First, you need to register and create a push service on Alibaba Cloud. After successful registration, you can obtain some necessary identity authentication information, such as Access Key and Access Secret. Next, configure this information into the PHP microservice to facilitate subsequent push operations. - Install Push SDK
In order to facilitate the operation of push services, we can use Alibaba Cloud Push SDK. Use Composer to install the SDK:
composer require alibabacloud/sdk
- Send push messages
You can send push messages through the following code example:
<?php require_once __DIR__ . '/vendor/autoload.php'; use AlibabaCloudClientAlibabaCloud; use AlibabaCloudClientExceptionClientException; use AlibabaCloudClientExceptionServerException; use AlibabaCloudClientResultResult; AlibabaCloud::accessKeyClient('<your_access_key>', '<your_access_secret>') ->regionId('cn-hangzhou') ->asDefaultClient(); try { $result = AlibabaCloud::rpc() ->product('Push') ->version('2016-08-01') ->action('Push') ->method('POST') ->host('push.aliyuncs.com') ->options([ 'query' => [ 'RegionId' => 'cn-hangzhou', 'Target' => 'all', 'TargetValue' => 'all', 'Title' => 'Hello, world!', 'Body' => 'This is a push message.', ], ]) ->request(); // 处理返回结果 if ($result instanceof Result) { if ($result->isSuccess()) { // 成功处理 echo 'Push message sent successfully'; } else { // 失败处理 echo 'Failed to send push message: ' . $result->getErrorMessage(); } } else { // 请求异常处理 echo 'Failed to send push message.'; } } catch (ClientException $e) { // 客户端异常处理 echo 'Failed to send push message: ' . $e->getMessage(); } catch (ServerException $e) { // 服务器异常处理 echo 'Failed to send push message: ' . $e->getMessage(); }
Summary:
Passed Using message queues and push services, we can implement distributed message notification and push functions in PHP microservices. Whether you use message queue or push service, you need to install, configure and call the corresponding API accordingly. Being familiar with these basic concepts and operating methods can help us better implement message notification and push functions in distributed systems and improve application reliability and performance. Of course, based on actual needs, we can also choose other suitable solutions to implement distributed message notification and push.
The above is the detailed content of How to implement distributed message notification and push in PHP microservices. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
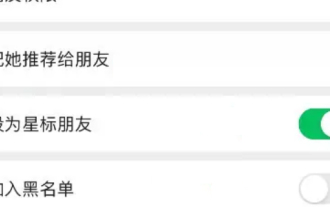
Business cards are a method that can be used to push friends in the software WeChat. Some users don’t know how to push friends’ business cards in WeChat. Just click on the friend’s personal page, select More to recommend them to friends and send them. This article is about WeChat push. The introduction of the friend’s business card method can tell you the specific content. The following is a detailed introduction, take a look! WeChat usage tutorial: How to push a friend’s business card on WeChat? Answer: Click on the friend’s personal page, select More to recommend them to friends and send them. Details: 1. Click on the friend you want to push a business card to. 2. Click the [More] option in the upper right corner. 3. Then click [Recommend TA to friends]. 4. Select the friend you want to send a business card to. 5. Click [Send].
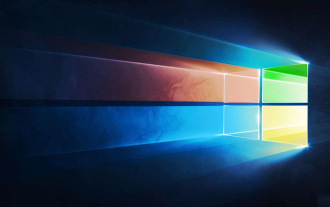
On January 9, 2020, Microsoft launched the latest and first new win10 system version in 2020. The version number of this update is win1019541. So friends who have been paying attention to Microsoft updates must be curious to know the specific content of this update. Well, according to the latest news obtained by the editor, this update is a multi-faceted improvement to the interface of the win10 system. When will the latest version of win10 19541 be pushed? Answer: The 19541 system push time is: January 9, 2020. This is the first Windows 10 preview update pushed by Microsoft after the Christmas holiday. Microsoft did not clarify whether this is the Windows 1020H1 version, so new features may be added.
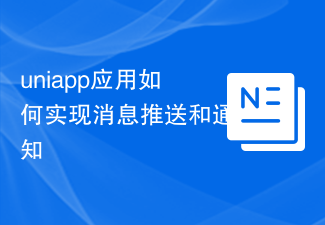
Uniapp is a cross-platform development framework based on Vue.js that can be used to develop applications that run on multiple platforms at the same time. When implementing message push and notification functions, Uniapp provides some corresponding plug-ins and APIs. The following will introduce how to use these plug-ins and APIs to implement message push and notification functions. 1. Message push To implement the message push function, we can use the uni-push plug-in provided by Uniapp. This plug-in is based on Tencent Cloud Push Service and can push messages on multiple platforms
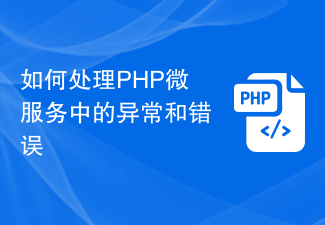
How to handle exceptions and errors in PHP microservices Introduction: With the popularity of microservice architecture, more and more developers choose to use PHP to implement microservices. However, due to the complexity of microservices, exception and error handling have become an essential topic. This article will introduce how to correctly handle exceptions and errors in PHP microservices and demonstrate it through specific code examples. 1. Exception handling In PHP microservices, exception handling is essential. Exceptions are unexpected situations encountered by the program during operation, such as database connection failure, A
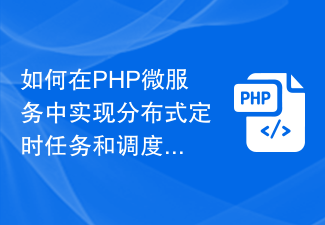
How to implement distributed scheduled tasks and scheduling in PHP microservices In modern microservice architecture, distributed scheduled tasks and scheduling are very important components. They can help developers easily manage, schedule and execute scheduled tasks in multiple microservices, improving system reliability and scalability. This article will introduce how to use PHP to implement distributed timing tasks and scheduling, and provide code examples for reference. Using a queue system In order to implement distributed scheduled tasks and scheduling, you first need to use a reliable queue system. Queuing systems can
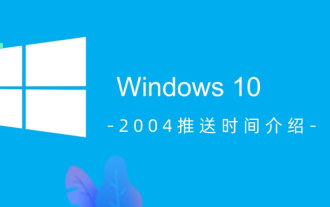
After Microsoft launched the new system win102004, many friends are paying attention to this new version of the system. So when is the push time for win102004? As far as the editor knows, it will be officially pushed on March 4, 2020. If we want to upgrade this new version of the system, we can also upgrade directly on the computer, or we can download and install the upgrade. Let’s take a look at what the editor said for details~ When is the win102004 push time? The win102004 version will be released to all Windows 10 users through the official channel on May 28. 1. Although this update does not add new features, users can still look forward to some good improvements in 20H1. 2. And Windows10
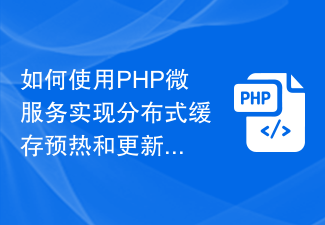
How to use PHP microservices to implement distributed cache warm-up and update Introduction: In modern web applications, caching is one of the important technical means to improve performance and reduce database load. The distributed cache can further improve the scalability and pressure resistance of the system. This article will introduce how to use PHP microservices to implement distributed cache warm-up and update, and provide some specific code examples. Requirements analysis: Our goal is to achieve two key functions through microservices: Cache warm-up: when the system starts, obtain data from the database and store it
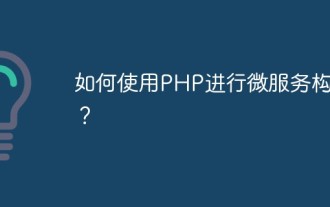
With the continuous development of the Internet and the continuous advancement of computer technology, microservice architecture has gradually become a hot topic in recent years. Different from the traditional monolithic application architecture, the microservice architecture decomposes a complex software application into multiple independent service units. Each service unit can be deployed, run and updated independently. The advantage of this architecture is that it improves the flexibility, scalability, and maintainability of the system. As an open source, Web-based programming language, PHP also plays a very important role in the microservice architecture.
