


How to use PHP to develop a simple classified information publishing system
How to use PHP to develop a simple classified information publishing system
Foreword:
The classified information publishing system is a common website application that allows users to Publish various categories of information, such as second-hand items, rental information, recruitment information, etc. This article will introduce how to use the PHP programming language to develop a simple classified information publishing system and provide corresponding code examples.
- System requirements analysis
Before starting coding, we need to clarify the system requirements to ensure that the development process proceeds smoothly. Generally speaking, a simple classified information release system needs to include the following functional modules:
(1) User registration and login: Users should be able to register a new account and log in to the system using the account.
(2) Publishing and management of classified information: Users should be able to publish various classified information and manage the published information.
(3) Information display and search: The system should be able to display published information and provide a search function to facilitate users to quickly find information of interest.
- Database design
The classified information publishing system needs to use a database to store user information and published information. The following is a simple database design example:
(1) User table (user): Contains the user's basic information, such as user ID, user name, password, etc.
(2) Classification information table (category): Contains basic information of classification information, such as information ID, title, content, etc.
(3) User information association table (user_category): used to associate users with published classification information.
- Implementation of user registration and login functions
First, we need to create a user table and provide the corresponding API interface for user registration and login. The following is a simple sample code:
(1) Create user table:
CREATE TABLE user
(
id
int(11) NOT NULL AUTO_INCREMENT,
username
varchar(255) NOT NULL,
password
varchar(255) NOT NULL,
PRIMARY KEY (id
)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
(2) Implementation of registration function:
// Get user submissions Form data
$username = $_POST['username'];
$password = $_POST['password'];
// Insert the data submitted by the user into the user table
$sql = "INSERT INTO user (username, password) VALUES ('$username', '$password')";
// Execute SQL statement
// ...
// Jump to the login page after successful registration
header('Location: login.php');
?>
(3) Implementation of login function:
// Get form data submitted by the user
$username = $_POST['username'];
$password = $_POST['password'];
/ / Find matching username and password in the user table
$sql = "SELECT * FROM user WHERE username = '$username' AND password = '$password'";
// Execute SQL statement
/ / ...
// If a matching user is found, the login is successful
if ($user) {
// 保存用户登录状态 session_start(); $_SESSION['user_id'] = $user['id']; // 跳转到用户首页 header('Location: user_home.php');
} else {
// 登录失败 echo '用户名或密码错误';
}
?>
- Implementation of publishing and management functions of classified information
Next, we need to create a classified information table and provide corresponding API interfaces for users to publish and manage information. The following is a simple sample code:
(1) Create a classification information table:
CREATE TABLE category
(
id
int(11) NOT NULL AUTO_INCREMENT,
title
varchar(255) NOT NULL,
content
varchar(255) NOT NULL,
user_id
int(11) NOT NULL,
PRIMARY KEY (id
),
FOREIGN KEY (user_id
) REFERENCES user
(id
)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
(2) Implementation of publishing information function:
// Get users Submitted form data
$title = $_POST['title'];
$content = $_POST['content'];
$user_id = $_SESSION['user_id'];
// Insert the information published by the user into the category information table
$sql = "INSERT INTO category (title, content, user_id) VALUES ('$title', '$content', '$user_id')" ;
// Execute SQL statement
// ...
// Jump to the information list page after successful publishing
header('Location: category_list.php');
?>
(3) Implementation of the information management function:
// Get all the information posted by the user based on the user ID
$user_id = $ _SESSION['user_id'];
$sql = "SELECT * FROM category WHERE user_id = '$user_id'";
// Execute SQL statement
// ...
/ / Traverse the query results and display the information posted by the user
foreach ($categories as $category) {
// ...
}
?>
- Display and search of information Implementation of the function
Finally, we need to create an information list page to display the published information and provide a search function. The following is a simple sample code:
(1) Implementation of the information list display function:
// 查询所有已发布的信息
$sql = "SELECT * FROM category";
// 执行SQL语句
// ...
// 遍历查询结果,展示所有信息
foreach ($categories as $category) {
// ...
}
?>
(2)搜索功能的实现:
// 获取用户提交的搜索关键字
$keyword = $_POST['keyword'];
// 根据关键字查询匹配的信息
$sql = "SELECT * FROM category WHERE title LIKE '%$keyword%'";
// 执行SQL语句
// ...
// 遍历查询结果,展示匹配的信息
foreach ($categories as $category) {
// ...
}
?>
总结:
本文介绍了如何使用PHP编程语言来开发一个简单的分类信息发布系统。通过以上示例,我们可以看到,利用PHP开发一个分类信息发布系统并不复杂,只需正确设计数据库结构,实现相应的功能模块即可。当然,这只是一个简化的示例,实际情况中还需要考虑安全性、性能等方面的问题。希望本文能对您有所帮助,谢谢阅读!
The above is the detailed content of How to use PHP to develop a simple classified information publishing system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


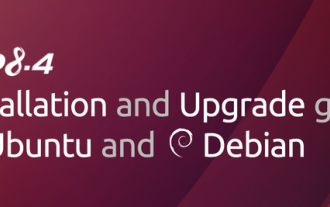
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
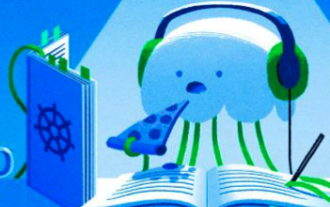
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
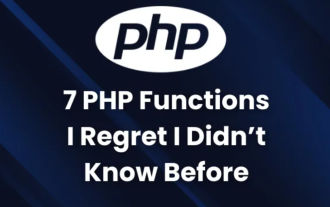
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
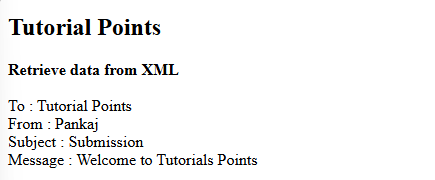
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
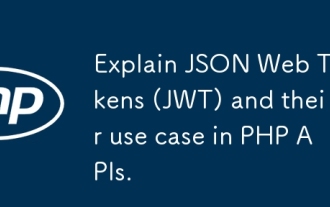
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
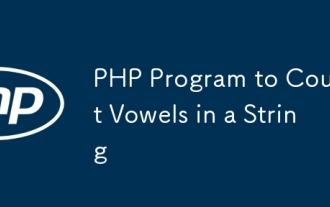
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
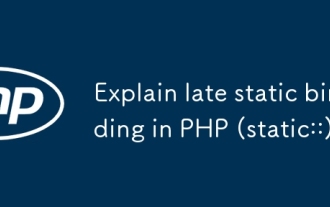
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
