


How to use PHP to implement a simple online photo album function
Use PHP to implement a simple online photo album function
With the popularity of digital cameras and smartphones, it has become very easy to take and store a large number of photos. To make it easier to browse and share these photos, we can create a simple online photo album feature. PHP is a widely used programming language suitable for developing dynamic websites. The following will introduce how to use PHP to implement a simple online photo album, and provide specific code examples.
Preparation
First, we need to install PHP on the web server. Apache, PHP, and MySQL can be installed using packages such as XAMPP, WAMP, or MAMP so they can be debugged in a local development environment. At the same time, a database is needed to store the album information, and MySQL or other databases can be used. In this article, we will use the MySQL database.
Create database and table
Create a new database in the MySQL database and name it "gallery". Then create a table named "photos" with the following columns: id, filename, title, and description.
PHP code implementation
First, create a file named "index.php" as the home page of the album. The homepage will display thumbnails of all the photos and provide links to each photo's page.
<!DOCTYPE html> <html> <head> <title>在线相册</title> <style> .thumbnail { width: 200px; height: 200px; object-fit: cover; } </style> </head> <body> <h1 id="我的相册">我的相册</h1> <?php $db = mysqli_connect("localhost", "username", "password", "gallery"); $query = "SELECT * FROM photos"; $result = mysqli_query($db, $query); while ($row = mysqli_fetch_assoc($result)) { $id = $row['id']; $filename = $row['filename']; $title = $row['title']; echo '<a href="photo.php?id=' . $id . '">'; echo '<img src="images/' . $filename . '" alt="' . $title . '" class="thumbnail">'; echo '</a>'; } mysqli_close($db); ?> </body> </html>
Then, create a file called "photo.php" that displays the details of a single photo.
<!DOCTYPE html> <html> <head> <title>照片详情</title> </head> <body> <?php $db = mysqli_connect("localhost", "username", "password", "gallery"); $id = $_GET['id']; $query = "SELECT * FROM photos WHERE id = $id"; $result = mysqli_query($db, $query); $row = mysqli_fetch_assoc($result); $filename = $row['filename']; $title = $row['title']; $description = $row['description']; echo '<h1 id="title">' . $title . '</h1>'; echo '<img src="images/' . $filename . '" alt="' . $title . '">'; echo '<p>' . $description . '</p>'; mysqli_close($db); ?> </body> </html>
Please replace "username" and "password" in the code with your database username and password, and make sure there are corresponding image files in the images folder.
Upload photos
In order to upload photos to the album, we can create a file named "upload.php". The file will provide a simple form where the user can select a photo file and enter a title and description.
<!DOCTYPE html> <html> <head> <title>上传照片</title> </head> <body> <h1 id="上传照片">上传照片</h1> <?php if ($_SERVER['REQUEST_METHOD'] === 'POST') { $db = mysqli_connect("localhost", "username", "password", "gallery"); $file = $_FILES['photo']; $filename = $file['name']; $title = $_POST['title']; $description = $_POST['description']; $target = 'images/' . $filename; move_uploaded_file($file['tmp_name'], $target); $query = "INSERT INTO photos (filename, title, description) VALUES ('$filename', '$title', '$description')"; mysqli_query($db, $query); echo '<p>照片已上传成功!</p>'; mysqli_close($db); } ?> <form action="upload.php" method="post" enctype="multipart/form-data"> <input type="file" name="photo" required> <input type="text" name="title" placeholder="标题" required> <textarea name="description" placeholder="描述" required></textarea> <button type="submit">上传</button> </form> </body> </html>
The above code implements a simple online photo album function. Users can add photos to the album through the photo upload form, and browse and view detailed information about each photo on the homepage.
Summary
By using PHP and MySQL, we can easily create a simple online photo album function. This makes browsing and sharing photos even more convenient. Hopefully the above code examples will help you get started building your own online photo album project.
The above is the detailed content of How to use PHP to implement a simple online photo album function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


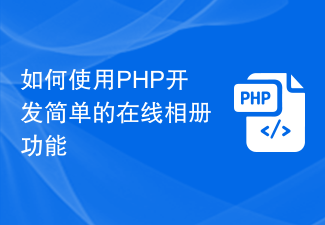
How to use PHP to develop a simple online photo album function Photo album is a common function for displaying pictures online. It can help us browse, share and download pictures conveniently. This article will introduce how to use PHP to develop a simple online photo album and provide specific code examples. 1. Set up a development environment First, we need to set up a development environment. You can choose to install an integrated development environment (IDE) such as XAMPP, WAMP or MAMP, which has built-in Apache server, MySQL database and PHP
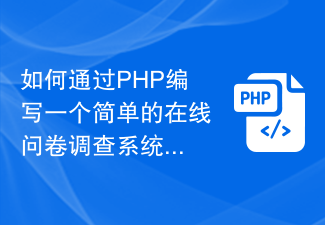
How to write a simple online questionnaire system through PHP. With the development and popularization of the Internet, more and more people are beginning to tend to conduct various surveys and questionnaires through the Internet. In order to meet this demand, we can write a simple online questionnaire system through PHP language. This article will introduce how to use PHP to implement a basic questionnaire system and provide specific code examples. Database design First, we need to design a database to store questionnaire-related data. We can use MySQL data
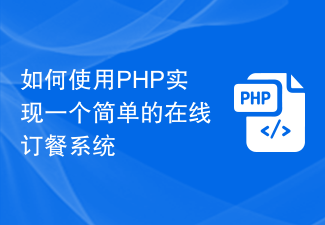
How to use PHP to implement a simple online ordering system. With the popularity of the Internet, the ordering industry is gradually developing online. In order to meet the needs of users, the development of online ordering systems has become very important. This article will introduce how to use PHP language to implement a simple online ordering system and provide specific code examples. Define the database structure First, we need to define the database structure to store order information. Create a data table named "orders" and define the following fields: order_id: order ID
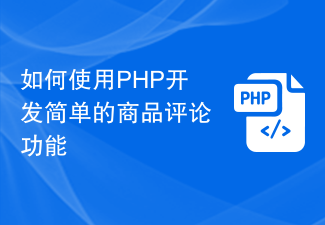
How to use PHP to develop a simple product review function. With the rise of e-commerce, the product review function has become an indispensable function to facilitate communication between users and consumers' evaluation of products. This article will introduce how to use PHP to develop a simple product review function, and attach specific code examples. Create a database First, we need to create a database to store product review information. Create a database called "product_comments" and within it a file called "comment
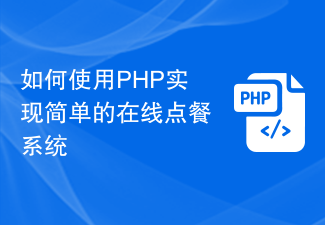
Today, we will implement a simple online ordering system using the PHP programming language. PHP is a popular server-side scripting language that is ideal for developing web-based applications. We'll show you how to use PHP to create a simple ordering system where users can select items on the website, add them to their shopping cart, and complete the transaction. Before starting, we need to create a new PHP project and install the necessary dependencies such as database and server environment. We will use MySQ
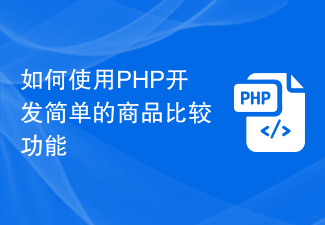
How to use PHP to develop a simple product comparison function requires specific code examples. With the development of e-commerce, users often encounter difficulties in selecting products when shopping, such as not knowing which brand of product to choose is better and which store has the best price. More affordable etc. In order to solve this problem, we can develop a simple product comparison function to help users easily compare the attributes of products and make choices. This article will introduce how to use PHP to implement this function and give specific code examples. First, we need to create a product
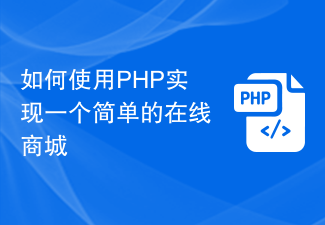
How to use PHP to implement a simple online mall With the development of the Internet, e-commerce has become a common way of shopping. Many people want to learn how to build their own online store. This article will introduce how to use PHP language to implement a simple online mall and give specific code examples. 1. Set up the environment First, we need to set up a PHP development environment locally. It is recommended to use XAMPP or WAMP tools to build an integrated development environment, which can avoid tedious configuration work. 2. Create database connection
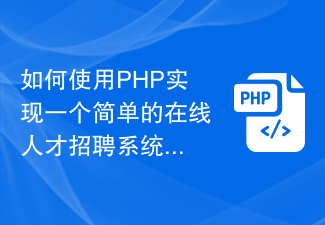
How to use PHP to implement a simple online talent recruitment system Talent recruitment is an important link in enterprise development. With the development of the Internet, more and more companies have begun to adopt online talent recruitment systems to simplify the recruitment process and improve efficiency. In this article, we will introduce how to use PHP language to implement a simple online talent recruitment system and provide specific code examples. 1. Requirements analysis Before implementing the online talent recruitment system, it is first necessary to clarify the system requirements. A simple talent recruitment system usually includes the following functions:
