


How to use PHP to develop the data shard storage function of the accounting system - Provides a development guide for the shard storage function
How to use PHP to develop the data shard storage function of the accounting system
With the rapid development of the Internet, the amount of data has increased exponentially. In order to cope with the storage and query requirements of large amounts of data, data shard storage has become a common solution. In applications such as the development of accounting systems that require efficient storage and query of large amounts of data, the data sharding storage function can greatly improve the performance and scalability of the system.
This article will focus on how to use PHP to develop the data shard storage function of the accounting system, and provide specific code examples to help readers better understand and practice.
1. What is the data sharding storage function?
Data shard storage is a storage method that stores large-scale data slices on multiple physical or logical nodes. By splitting data into smaller chunks, data can be stored on multiple nodes, improving storage and query efficiency. At the same time, data shard storage also has good scalability. When the amount of data increases, just adding new nodes can complete the expansion.
2. Considerations for designing sharded storage solutions for accounting systems
- Data segmentation rules: When designing data segmentation rules, it needs to be based on actual business needs and data characteristics. Make reasonable divisions. Common segmentation rules include segmentation by time, segmentation by user, etc. In the accounting system, it can be segmented based on the date of the bill.
- Number of nodes: Determine the number of nodes based on the size of the data volume and the estimated data growth rate. The greater the number of nodes, the higher the efficiency of data storage and query, but it will also increase the complexity and maintenance cost of the system.
- Data replication strategy: In order to ensure data reliability and high availability, you need to consider how to perform data backup and replication. Common strategies include master-slave replication, multi-copy storage, etc.
3. Sample code for using PHP to implement the data sharding storage function
The following is a sample code for using PHP to implement the data sharding storage function for the accounting system:
<?php class ShardingStorage { private $servers = array( array('host' => 'server1', 'port' => '3306'), array('host' => 'server2', 'port' => '3306'), array('host' => 'server3', 'port' => '3306') ); private function getServer($key) { $num = count($this->servers); $index = crc32($key) % $num; return $this->servers[$index]; } public function saveData($key, $value) { $server = $this->getServer($key); // 连接服务器并保存数据 $conn = new mysqli($server['host'], 'username', 'password', 'database', $server['port']); $sql = "INSERT INTO table (key, value) VALUES ('$key', '$value')"; $conn->query($sql); $conn->close(); } public function getData($key) { $server = $this->getServer($key); // 连接服务器并查询数据 $conn = new mysqli($server['host'], 'username', 'password', 'database', $server['port']); $sql = "SELECT value FROM table WHERE key='$key'"; $result = $conn->query($sql); $data = $result->fetch_assoc()['value']; $conn->close(); return $data; } } $shardingStorage = new ShardingStorage(); $shardingStorage->saveData('20211201', '100.00'); $data = $shardingStorage->getData('20211201'); echo $data; // 输出:100.00 ?>
The above example code simply demonstrates how to use PHP to implement the data sharding storage function. By defining a ShardingStorage
class, select the corresponding server according to the key value of the data, and then save and query the data according to requirements.
It should be noted that in actual projects, functions such as error handling, connection pool management, and data replication strategies need to be added to further improve the system.
4. Summary
The data shard storage function is a technology commonly used in developing large data applications such as accounting systems. This article introduces how to use PHP to develop the data shard storage function of the accounting system and provides specific code examples. By properly designing data segmentation rules, increasing the number of nodes and implementing data replication strategies, the performance and scalability of the system can be improved.
I hope this article can help readers better understand and apply data sharding storage technology, and provide an effective solution for the development of accounting systems or other large data applications.
The above is the detailed content of How to use PHP to develop the data shard storage function of the accounting system - Provides a development guide for the shard storage function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


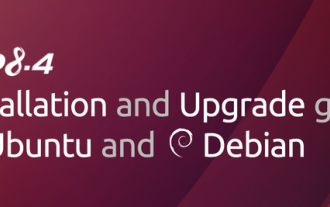
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
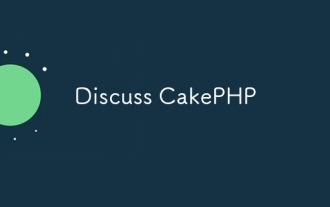
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
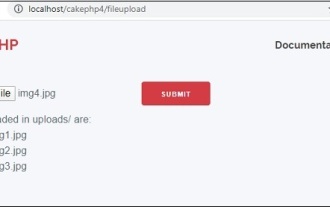
To work on file upload we are going to use the form helper. Here, is an example for file upload.
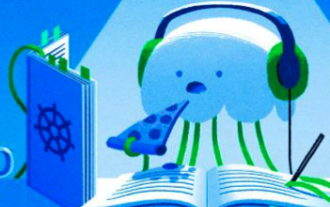
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
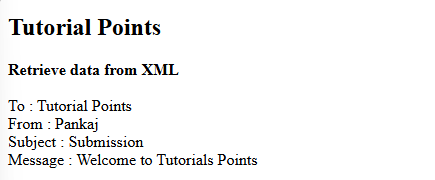
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
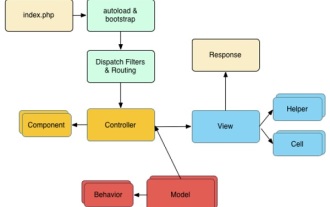
CakePHP is an open source MVC framework. It makes developing, deploying and maintaining applications much easier. CakePHP has a number of libraries to reduce the overload of most common tasks.
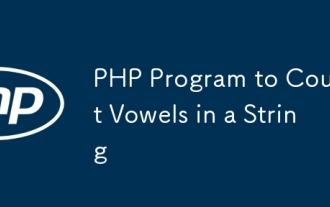
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
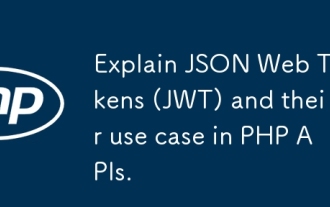
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
