How to use PHP to implement a simple data encryption function
How to use PHP to implement a simple data encryption function requires specific code examples
In the modern Internet era, data security has become an issue that we cannot ignore. In website development, to protect some sensitive data, we need to use encryption algorithms to prevent data leakage and tampering. PHP is a scripting language widely used in server-side development. It provides a wealth of encryption functions and algorithms. This article will introduce how to use PHP to implement a simple data encryption function and give specific code examples.
1. Use PHP built-in functions to implement encryption functions
PHP has built-in some commonly used encryption functions, such as md5 and sha1, which can be used to encrypt strings. Here is a sample code that demonstrates how to use the md5 function to encrypt a string:
<?php $str = 'Hello World'; $encrypted_str = md5($str); echo '加密后的字符串:' . $encrypted_str; ?>
This code will output the encrypted string: 5eb63bbbe01eeed093cb22bb8f5acdc3. The md5 function converts a string into a 32-bit hexadecimal number. This number is irreversible, that is, the original string cannot be restored from the encrypted string.
2. Use symmetric encryption algorithm to implement encryption function
In addition to using PHP's built-in encryption function, we can also use symmetric encryption algorithm to encrypt data. Symmetric encryption algorithms use the same key, called a secret key, to encrypt and decrypt data. The following is a sample code that demonstrates how to use a symmetric encryption algorithm to implement data encryption:
<?php $str = 'Hello World'; $key = 'mysecretkey'; $encrypted_str = base64_encode(openssl_encrypt($str, 'AES-128-CBC', $key, OPENSSL_RAW_DATA, '1234567890123456')); echo '加密后的字符串:' . $encrypted_str; $decrypted_str = openssl_decrypt(base64_decode($encrypted_str), 'AES-128-CBC', $key, OPENSSL_RAW_DATA, '1234567890123456'); echo '解密后的字符串:' . $decrypted_str; ?>
This code uses the openssl_encrypt and openssl_decrypt functions to implement encryption and decryption functions. Among them, 'AES-128-CBC' means using the AES algorithm for encryption, 'OPENSSL_RAW_DATA' means that both input and output are raw data (without base64 encoding), and '1234567890123456' means the initialization vector (IV) used. The encrypted string is encoded through the base64_encode function, and decoded through the base64_decode function during decryption.
3. Use asymmetric encryption algorithm to implement encryption function
Asymmetric encryption algorithm uses a pair of keys, namely public key and private key. The public key is used to encrypt data and the private key is used to decrypt data. The following is a sample code that demonstrates how to use an asymmetric encryption algorithm to implement data encryption:
<?php $str = 'Hello World'; // 生成密钥对 $config = array( "private_key_bits" => 1024, "private_key_type" => OPENSSL_KEYTYPE_RSA, ); $res = openssl_pkey_new($config); openssl_pkey_export($res, $private_key); $public_key = openssl_pkey_get_details($res); $public_key = $public_key["key"]; // 公钥加密 openssl_public_encrypt($str, $encrypted_str, $public_key); echo '加密后的字符串:' . base64_encode($encrypted_str); // 私钥解密 openssl_private_decrypt($encrypted_str, $decrypted_str, $private_key); echo '解密后的字符串:' . $decrypted_str; ?>
This code uses openssl_pkey_new, openssl_pkey_export and openssl_pkey_get_details functions to generate public and private keys. The openssl_public_encrypt function is used for encryption, and the openssl_private_decrypt function is used for decryption. The encrypted string is encoded through the base64_encode function, and decoded through the base64_decode function during decryption.
4. Summary
This article introduces how to use PHP to implement a simple data encryption function, including using PHP built-in functions, symmetric encryption algorithms and asymmetric encryption algorithms. By using these encryption algorithms, we can protect the security of sensitive data. In practical applications, appropriate encryption algorithms are selected according to specific needs and scenarios to protect data security.
The above is the detailed content of How to use PHP to implement a simple data encryption function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


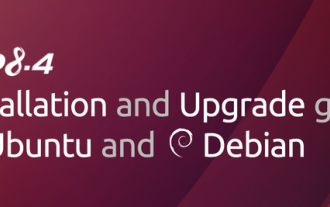
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
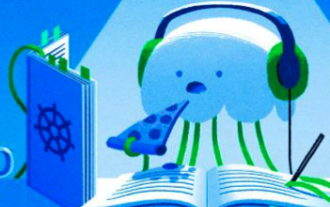
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
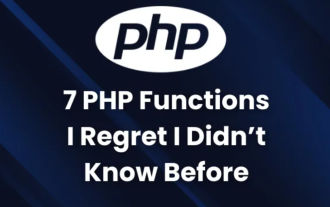
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
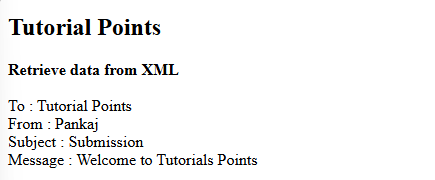
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
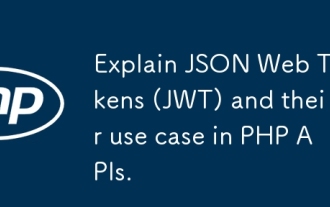
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
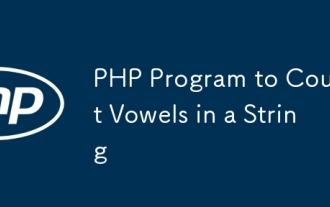
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
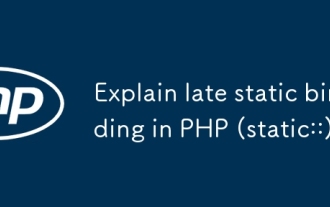
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
