


How to implement the automatic generation function of employee attendance through PHP and Vue
How to realize the automatic generation function of employee attendance through PHP and Vue
Introduction:
Employee attendance is a very important part of enterprise management. Traditional manual Recording attendance data is time-consuming, labor-intensive, and error-prone. With the help of two powerful development tools, PHP and Vue, we can easily realize the automatic generation function of employee attendance and improve the accuracy of attendance data and work efficiency. This article will introduce in detail how to implement the automatic generation function of employee attendance through PHP and Vue, and attach specific code examples.
1. Preparation
- Install PHP and Vue related development environment
- Create a database including employee table and attendance table
- In attendance The fields added to the table include: employee ID, attendance date, work time, and off work time
2. Back-end development (PHP)
- Create a PHP file and name it "attendance.php", this file is used to process front-end requests and interact with the database
- Write code in the "attendance.php" file to achieve the following functions:
a) Connect to the database
b) Receive the employee ID and attendance date passed by the front end
c) Query the employee's attendance record on that date
d) If there is a record, return the existing data; otherwise, automatically generate the attendance record and insert it into the database -
The following is a simplified version of the code example:
<?php // 连接数据库 $conn = new mysqli("localhost", "username", "password", "database"); // 检查连接 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } // 接收员工ID和考勤日期 $empId = $_POST["empId"]; $date = $_POST["date"]; // 查询考勤记录 $sql = "SELECT * FROM attendance WHERE emp_id = $empId AND date = $date"; $result = $conn->query($sql); // 如果有记录,则返回已有的数据 if ($result->num_rows > 0) { $row = $result->fetch_assoc(); echo json_encode($row); } else { // 自动生成考勤记录 $insertSql = "INSERT INTO attendance (emp_id, date, clock_in_time, clock_out_time) VALUES ($empId, $date, '09:00:00', '18:00:00')"; if ($conn->query($insertSql) === TRUE) { echo "考勤记录已生成"; } else { echo "生成考勤记录失败: " . $conn->error; } } $conn->close(); ?>
Copy after login
3. Front-end development (Vue)
- Create a Vue project , and use the axios library to send requests to the backend
- Write code in the Vue file to achieve the following functions:
a) Build the page, including the employee ID input box, attendance date selector and submit button
b) Listen to the form submission event and obtain the employee ID and attendance date entered by the user
c) Use the axios library to send a POST request to the back-end "attendance.php" file
d) Process the data returned by the background and update it The page displays The following is a simplified version of the code example:
<template> <div> <label for="empId">员工ID:</label> <input type="text" id="empId" v-model="empId"> <label for="date">考勤日期:</label> <input type="date" id="date" v-model="date"> <button @click="submit">提交</button> <p v-if="attendance">上班时间:{{ attendance.clock_in_time }},下班时间:{{ attendance.clock_out_time }}</p> </div> </template> <script> import axios from 'axios'; export default { data() { return { empId: '', date: '', attendance: null } }, methods: { submit() { axios.post('attendance.php', { empId: this.empId, date: this.date }) .then(response => { this.attendance = response.data; }) .catch(error => { console.log(error); }); } } } </script>
Copy after login
4. Run
- Run the Vue project in the terminal , and visit the corresponding URL
- Enter the employee ID and attendance date on the page, click the submit button
- The page will display the employee’s attendance record on that date. If there is no record, it will automatically The generation function will generate attendance records and display them on the page
Summary:
Through the combination of PHP and Vue, we have realized the automatic generation function of employee attendance. PHP is responsible for back-end processing and database interaction, and Vue is responsible for front-end page construction and communication with the back-end. This method can greatly improve the accuracy and work efficiency of employee attendance data, and reduce the errors and tediousness caused by manual recording. Of course, this is just a simplified version of the example, and actual projects need to be appropriately expanded and optimized according to needs. I hope this article will be helpful to readers who are learning and practicing PHP and Vue.
The above is the detailed content of How to implement the automatic generation function of employee attendance through PHP and Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


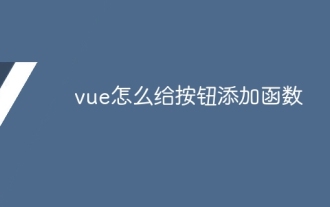
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.

The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
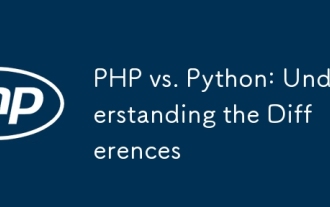
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
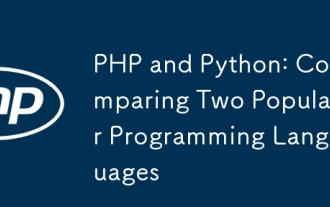
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
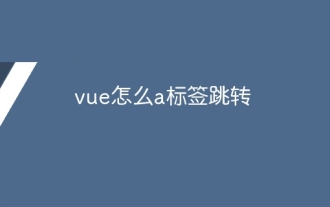
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
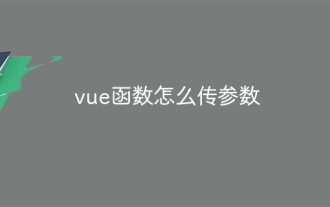
There are two main ways to pass parameters to Vue.js functions: pass data using slots or bind a function with bind, and provide parameters: pass parameters using slots: pass data in component templates, accessed within components and used as parameters of the function. Pass parameters using bind binding: bind function in Vue.js instance and provide function parameters.
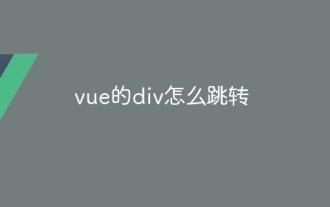
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.
