


React API Call Guide: How to interact and transfer data with the backend API
React API Calling Guide: How to interact and transfer data with the backend API
Overview:
In modern web development, how to interact with the backend API Interaction and data transfer is a common requirement. React, as a popular front-end framework, provides some powerful tools and features to simplify this process. This article will introduce how to use React to call the backend API, including basic GET and POST requests, and provide specific code examples.
-
Install the required dependencies:
First, make sure you have Axios installed in your project, which is a popular HTTP client for sending and receiving HTTP requests. Use the following command to install Axios:npm install axios
Copy after login Send a GET request:
Sending a GET request is one of the most common ways to get data from the backend API. In React, you can send a GET request in the component'scomponentDidMount
lifecycle method. The following example shows how to use Axios to send a GET request and handle the response:import React, { Component } from 'react'; import axios from 'axios'; class MyComponent extends Component { componentDidMount() { axios.get('/api/endpoint') .then(response => { // 处理响应数据 console.log(response.data); }) .catch(error => { // 错误处理 console.error(error); }); } render() { return ( // 组件的渲染内容 ); } } export default MyComponent;
Copy after loginSend a POST request:
In addition to getting data, React can also use Axios to send a POST request to the backend API sends data. The following example shows how to use Axios to send a POST request and handle the response:import React, { Component } from 'react'; import axios from 'axios'; class MyComponent extends Component { handleSubmit = (event) => { event.preventDefault(); const data = { // 要发送的数据 }; axios.post('/api/endpoint', data) .then(response => { // 处理响应数据 console.log(response.data); }) .catch(error => { // 错误处理 console.error(error); }); } render() { return ( <form onSubmit={this.handleSubmit}> {/* 表单内容 */} <button type="submit">提交</button> </form> ); } } export default MyComponent;
Copy after login- Handling API responses:
Handling responses from the API may vary depending on the design of the backend API. Typically, the API's response data will be contained in thedata
attribute of the response. Depending on the data type returned by the backend API (JSON, plain text, etc.), the response data can be processed in an appropriate manner. - Error handling:
Error handling is an important aspect when interacting with the backend API. You can use thecatch
method to catch requests that fail and handle the error appropriately. This may include displaying error messages to the user or logging errors.
Summary:
By using the Axios library, React components can easily interact and transmit data with the back-end API. The above example provides a basic way to send GET and POST requests and process the response data. However, this is only the basis for API calls. According to specific project needs, other functions can be added, such as handling authentication, request interception, etc.
After all, interacting with the backend API is an important part of React development, and mastering the skills of API calls will help us build more powerful and flexible web applications.
The above is the detailed content of React API Call Guide: How to interact and transfer data with the backend API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


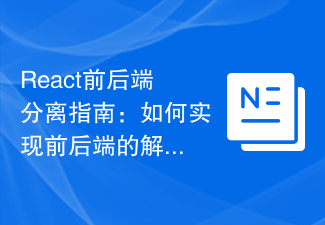
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
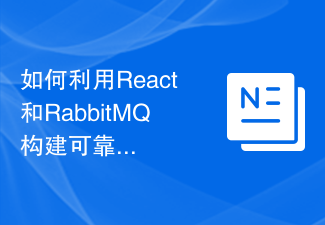
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
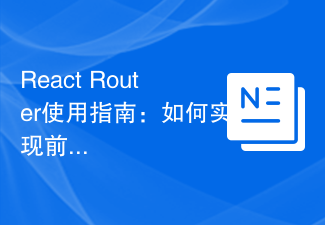
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
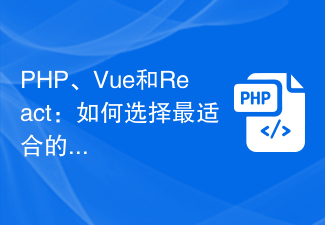
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
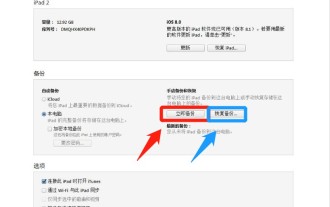
When many friends change their Apple phones, they want to import all the data in the old phone to the new phone. In theory, it is completely feasible, but in practice, it is impossible to "transfer all" the data. This issue's article List several ways to "transfer part of the data". 1. iTunes is a pre-installed software on Apple mobile phones. It can be used to migrate all data in old mobile phones, but it needs to be used in conjunction with a computer. The migration can be completed by installing iTunes on the computer, then connecting the phone and computer via a data cable, using iTunes to back up the apps and data in the phone, and finally restoring the backup to the new Apple phone. 2. iCloudiCloud is Apple’s exclusive “cloud space” tool. You can log in to your old phone first.
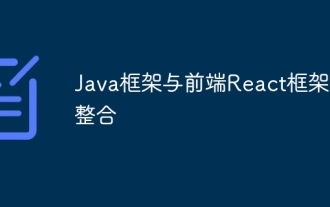
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.

Oracle is a world-renowned database management system provider, and its API (Application Programming Interface) is a powerful tool that helps developers easily interact and integrate with Oracle databases. In this article, we will delve into the Oracle API usage guide, show readers how to utilize data interface technology during the development process, and provide specific code examples. 1.Oracle
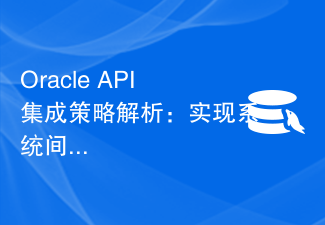
OracleAPI integration strategy analysis: To achieve seamless communication between systems, specific code examples are required. In today's digital era, internal enterprise systems need to communicate with each other and share data, and OracleAPI is one of the important tools to help achieve seamless communication between systems. This article will start with the basic concepts and principles of OracleAPI, explore API integration strategies, and finally give specific code examples to help readers better understand and apply OracleAPI. 1. Basic Oracle API
