


React code debugging guide: How to quickly locate and solve front-end bugs
React Code Debugging Guide: How to quickly locate and solve front-end bugs
Introduction:
When developing React applications, you often encounter various bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications.
1. Selection of debugging tools:
In React applications, there are many tools that can help us debug the code. The following are several commonly used debugging tools:
- Chrome Developer Tools: The developer tools that come with the Chrome browser are a powerful debugging tool that can inspect elements, view network requests, and view logs and other functions to debug React code.
- React Developer Tools: This is a Chrome plug-in that provides more intuitive and detailed React component level information, as well as functions to help observe and modify the state of React components.
- Redux DevTools: If your application uses Redux as a state management library, it is very helpful to use Redux DevTools to debug the Redux state flow. It can help you view and modify the status in the Redux store, as well as review historical status.
2. Locating React component exceptions:
- Use the Elements panel of Chrome developer tools to check the React component hierarchy and see if the rendering results are as expected. You can determine the specific problem by checking component Props and state, and troubleshooting components that may be faulty.
Sample code:
Suppose we have a TodoList component that displays a to-do list.
import React, { useState } from 'react'; function TodoList() { const [todos, setTodos] = useState([]); function addTodo() { setTodos([...todos, { id: Date.now(), text: 'New todo' }]); } return ( <div> <button onClick={addTodo}>Add Todo</button> {todos.map((todo) => ( <div key={todo.id}>{todo.text}</div> ))} </div> ); } export default TodoList;
Suppose an error is encountered when rendering the to-do list, and the corresponding rendering result cannot be displayed on the page. We can use the Elements panel of the Chrome developer tools to check whether there are rendering exceptions and see whether the status and Props are passed correctly.
- Use the Console panel of Chrome Developer Tools to view warning and error messages in React components. React usually provides useful warning and error messages in development mode to help us locate specific problems.
Sample code:
Modify the TodoList component above to intentionally cause an error when rendering the to-do list.
import React, { useState } from 'react'; function TodoList() { const [todos, setTodos] = useState([]); function addTodo() { setTodos([...todos, { id: Date.now(), text: 'New todo' }]); } // 引发错误:todos.map is not a function const renderedTodos = todos.map((todo) => <div key={todo.id}>{todo.text}</div>); return ( <div> <button onClick={addTodo}>Add Todo</button> {renderedTodos} </div> ); } export default TodoList;
After refreshing the page, check the Console panel of Chrome developer tools and you can see the error message: todos.map is not a function
. Through this error message, we can locate the location where the error occurred in the todos.map
line of code.
3. Use breakpoint debugging:
- In the Sources panel of Chrome developer tools, we can use the breakpoint debugging function to pause code execution on a certain line. At this time, we can view the value of the variable, call stack, execution context and other information to help us locate and solve the problem.
Sample code:
In the above TodoList component, we can set a breakpoint when clicking the button to add a to-do item.
import React, { useState } from 'react'; function TodoList() { const [todos, setTodos] = useState([]); function addTodo() { debugger; // 设置断点 setTodos([...todos, { id: Date.now(), text: 'New todo' }]); } return ( <div> <button onClick={addTodo}>Add Todo</button> </div> ); } export default TodoList;
Refresh the page and open the Sources panel of Chrome Developer Tools, then click the button. The code will pause execution at the debugger
line. At this time, we can view the code execution line by line and check whether the variable values are correct.
- In Redux development, you can use Redux DevTools to debug Redux state flow. Through Redux DevTools, we can view and modify the status in the Redux store, review historical status, and view the dispatch of Actions, etc.
Sample code:
If we have a Redux Store, it contains todos and filter states.
import { createStore } from 'redux'; const initialState = { todos: [], filter: 'all', }; // 定义reducer函数 function reducer(state = initialState, action) { switch (action.type) { case 'ADD_TODO': return { ...state, todos: [...state.todos, action.payload], }; case 'SET_FILTER': return { ...state, filter: action.payload, }; default: return state; } } // 创建store const store = createStore(reducer); export default store;
We can use Redux DevTools to view and modify todos and filter status, as well as the execution of dispatched Actions.
Conclusion:
By using various debugging tools and techniques, we can quickly locate and solve front-end bugs. From checking the React component structure, viewing warning and error messages, to using breakpoint debugging and Redux DevTools, these methods can help us debug React code comprehensively and efficiently. Mastering these skills will significantly improve our efficiency and debugging capabilities in React development.
The above is the detailed content of React code debugging guide: How to quickly locate and solve front-end bugs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


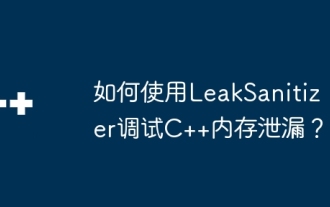
How to use LeakSanitizer to debug C++ memory leaks? Install LeakSanitizer. Enable LeakSanitizer via compile flag. Run the application and analyze the LeakSanitizer report. Identify memory allocation types and allocation locations. Fix memory leaks and ensure all dynamically allocated memory is released.
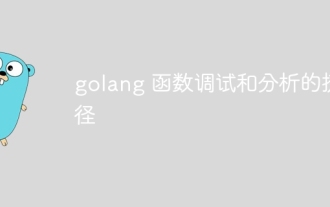
This article introduces shortcuts for Go function debugging and analysis, including: built-in debugger dlv, which is used to pause execution, check variables, and set breakpoints. Logging, use the log package to record messages and view them during debugging. The performance analysis tool pprof generates call graphs and analyzes performance, and uses gotoolpprof to analyze data. Practical case: Analyze memory leaks through pprof and generate a call graph to display the functions that cause leaks.
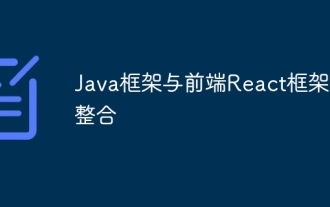
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
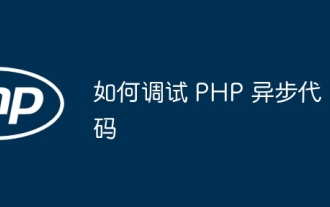
Tools for debugging PHP asynchronous code include: Psalm: a static analysis tool that can find potential errors. ParallelLint: A tool that inspects asynchronous code and provides recommendations. Xdebug: An extension for debugging PHP applications by enabling a session and stepping through the code. Other tips include using logging, assertions, running code locally, and writing unit tests.
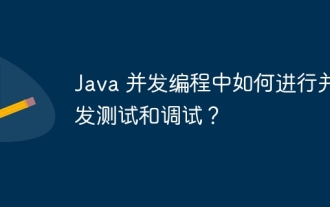
Concurrency testing and debugging Concurrency testing and debugging in Java concurrent programming are crucial and the following techniques are available: Concurrency testing: Unit testing: Isolate and test a single concurrent task. Integration testing: testing the interaction between multiple concurrent tasks. Load testing: Evaluate an application's performance and scalability under heavy load. Concurrency Debugging: Breakpoints: Pause thread execution and inspect variables or execute code. Logging: Record thread events and status. Stack trace: Identify the source of the exception. Visualization tools: Monitor thread activity and resource usage.
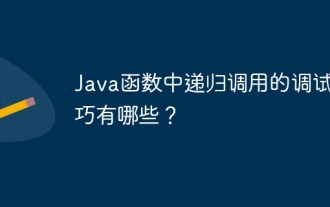
The following techniques are available for debugging recursive functions: Check the stack traceSet debug pointsCheck if the base case is implemented correctlyCount the number of recursive callsVisualize the recursive stack
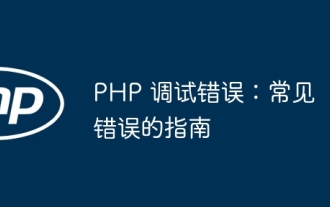
Common PHP debugging errors include: Syntax errors: Check the code syntax to make sure there are no errors. Undefined variable: Before using a variable, make sure it is initialized and assigned a value. Missing semicolons: Add semicolons to all code blocks. Function is undefined: Check that the function name is spelled correctly and make sure the correct file or PHP extension is loaded.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
