How to implement data copy function using PHP and Vue
How to use PHP and Vue to implement data replication function
In modern web application development, data replication is a common requirement. For example, when the user needs to copy data from one table to another table, or needs to copy the content of one article to another article. This article will introduce how to use PHP and Vue to implement such data copy function, and provide specific code examples.
- Preparation
Before you start, make sure you have set up the development environment for PHP and Vue. If you haven't already, please install PHP and Vue first and make sure they work properly. - Create database tables
First, we need to create two tables in the database to store original data and copied data respectively. The following is a simple example:
CREATE TABLE original_data ( id INT PRIMARY KEY AUTO_INCREMENT, content TEXT ); CREATE TABLE copied_data ( id INT PRIMARY KEY AUTO_INCREMENT, content TEXT );
- Create a backend API
Next, we need to create a backend API to handle the logic of data replication. In PHP, we can use PDO extension to connect to the database and execute SQL queries. The following is a simple example:
<?php header('Content-Type: application/json'); $pdo = new PDO('mysql:host=localhost;dbname=your_database_name;charset=utf8', 'your_username', 'your_password'); if ($_SERVER['REQUEST_METHOD'] === 'POST') { $content = $_POST['content']; $insertSql = "INSERT INTO copied_data (content) VALUES (:content)"; $statement = $pdo->prepare($insertSql); $statement->bindParam(':content', $content); $statement->execute(); $result = ['success' => true]; } else { $result = ['success' => false, 'message' => 'Invalid request method']; } echo json_encode($result); ?>
- Creating the front-end interface
Now we can start creating the front-end interface. In Vue, we can use the axios library to send HTTP requests. The following is a simple example:
<template> <div> <textarea v-model="originalData"></textarea> <button @click="copyData">复制</button> </div> </template> <script> import axios from 'axios'; export default { data() { return { originalData: '', }; }, methods: { copyData() { axios.post('/api/copy_data.php', { content: this.originalData }) .then(response => { if (response.data.success) { alert('复制成功!'); } else { alert('复制失败,请重试。'); } }) .catch(error => { console.error(error); alert('服务器错误,请稍后再试。'); }); }, }, }; </script>
In the above code, we use Vue's two-way binding to process what the user enters in the text box. When the user clicks the copy button, we send a POST request to the backend API and use the user input as a parameter of the request. Based on the results returned by the backend, we will pop up a prompt box to inform the user whether the copy is successful.
- Connect the front and back ends
Finally, we need to connect the front and back ends. In the Vue entry file, add the following code:
import Vue from 'vue'; import App from './App.vue'; Vue.config.productionTip = false; new Vue({ render: h => h(App), }).$mount('#app');
Now, we have completed all the steps to implement the data copy function using PHP and Vue. When you run the application, you will be able to enter content in the text box and copy it to another form by clicking the copy button. Is not it simple? Hurry up and give it a try!
To sum up, this article introduces how to use PHP and Vue to implement the data copy function, and provides specific code examples. Hope this article helps you!
The above is the detailed content of How to implement data copy function using PHP and Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
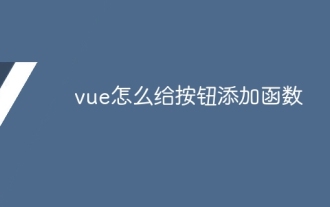
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.
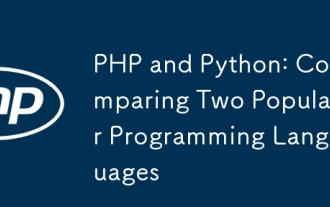
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.

The future of PHP will be achieved by adapting to new technology trends and introducing innovative features: 1) Adapting to cloud computing, containerization and microservice architectures, supporting Docker and Kubernetes; 2) introducing JIT compilers and enumeration types to improve performance and data processing efficiency; 3) Continuously optimize performance and promote best practices.
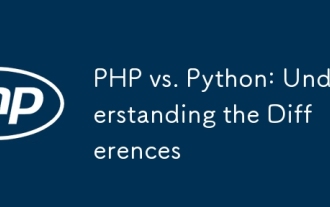
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
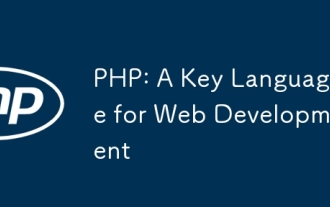
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
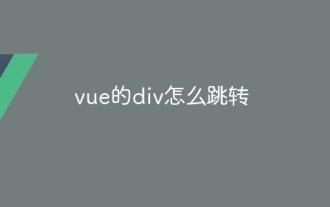
There are two ways to jump div elements in Vue: use Vue Router and add router-link component. Add the @click event listener and call this.$router.push() method to jump.
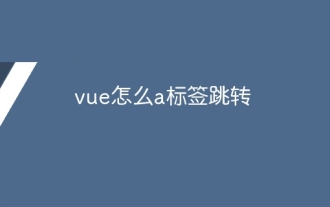
The methods to implement the jump of a tag in Vue include: using the a tag in the HTML template to specify the href attribute. Use the router-link component of Vue routing. Use this.$router.push() method in JavaScript. Parameters can be passed through the query parameter and routes are configured in the router options for dynamic jumps.
