


React component design principles: How to design scalable and maintainable front-end components
React component design principles: How to design scalable and maintainable front-end components
Introduction:
In modern front-end development, use the React framework to build componentization The application has become a mainstream development method. A well-designed React component can increase code reusability, scalability, and maintainability. This article will introduce some design principles to help developers design better React components. At the same time, we will provide some specific code examples to help readers understand better.
1. Single Responsibility Principle
The single responsibility principle requires that each component is only responsible for one function. It helps improve the reusability and maintainability of components. When a component takes on too many responsibilities, it can become bloated and difficult to maintain.
For example, suppose we are building a user information display component. According to the single responsibility principle, we can decompose the component into the following two sub-components:
-
User avatar component:
function Avatar({ url }) { return <img src={url} alt="User Avatar" />; }
Copy after login User information component:
function UserInfo({ name, age }) { return ( <div> <h1 id="name">{name}</h1> <p>Age: {age}</p> </div> ); }
Copy after login
By splitting functions into different components, we can combine these sub-components more flexibly and achieve stronger reusability.
2. Stateless Function Component
Stateless function component is a simplified component form that only accepts input parameters and returns a React element. Because they don't care about component lifecycle or state management, they are easier to write, test, and maintain.
For example, we can use stateless function components to create a simple button component:
function Button({ text, onClick }) { return <button onClick={onClick}>{text}</button>; }
3. Component composition is better than inheritance
In React, component composition is more flexible than inheritance and scalable. By combining small and simple components to build large, complex components, we can better manage dependencies between components and make the entire application easier to understand and maintain.
For example, we can create a complete user card component by combining the above-mentioned "User Avatar Component" and "User Information Component":
function UserCard({ user }) { return ( <div> <Avatar url={user.avatarUrl} /> <UserInfo name={user.name} age={user.age} /> </div> ); }
4. Use component status appropriately
Component state is one of the core concepts of components, which allows us to render components based on changes in data. However, misuse of component state can lead to components that become complex, difficult to understand, and difficult to maintain. Therefore, we need to carefully consider which data should be used as state when designing components, and try to limit the scope of the state to a minimum.
A common anti-pattern is to store all data in the component's state, the so-called "Big Mac state". In order to avoid this situation, we can store the data in component state or component properties according to the needs of the data.
For example, consider a simple counter component, we only need to store the current count value:
function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); }
5. Reasonable use of life cycle methods
Life cycle methods can be used to manage components Create, update and destroy. However, after React 16.3, the life cycle method has been deprecated, and it is recommended to use Effect Hook instead. Effect Hook can help us manage side effect operations.
For example, we can use Effect Hook to start a timer after the component is mounted, and clear the timer when the component is unmounted:
function Timer() { useEffect(() => { const timer = setInterval(() => { console.log('Tick'); }, 1000); return () => { clearInterval(timer); }; }, []); return <div>Timer Component</div>; }
6. Good naming and documentation comments
Good naming and documentation comments are very important for the understandability and maintainability of components. We should give components, properties, and methods a descriptive name and provide necessary documentation comments for them.
For example, we can name and annotate our components using the following:
/** * Button组件 * @param {string} text - 按钮文本 * @param {function} onClick - 点击事件处理函数 */ function Button({ text, onClick }) { return <button onClick={onClick}>{text}</button>; }
Conclusion:
Designing scalable and maintainable React components is an important part of front-end development. By following the single responsibility principle, using stateless functional components, rational use of component composition and state management, appropriate use of life cycle methods, and good naming and documentation comments, we can design more flexible and maintainable React components.
Of course, in addition to the principles mentioned above, there are many other design principles that can help us build better React components. In practice, we should choose appropriate principles and practices based on the specific needs of the project and the agreement of the team. I hope this article can provide readers with some help and inspiration in React component design.
The above is the detailed content of React component design principles: How to design scalable and maintainable front-end components. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


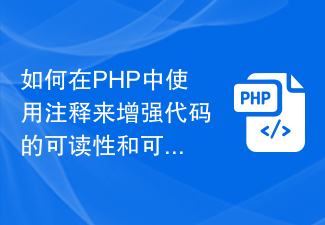
How to use comments in PHP to enhance code readability and maintainability Introduction: In the software development process, code readability and maintainability are very important. It can be said that comments are part of the code and can help developers better understand and maintain the code. Especially in large projects, a good comment style can make the code easier to understand and easier to debug and modify. This article will introduce how to use comments in PHP to enhance the readability and maintainability of code, and illustrate it through code examples. 1. Basic usage notes of comments

C++ code readability optimization: improving code understandability and maintainability Introduction: In software development, code readability is a very important factor. Readable code can make the code easier to understand, debug and maintain, and easier for teamwork and development. For high-level programming languages like C++, how to optimize the readability of the code is particularly important. This article will discuss some techniques to improve the readability of C++ code to help developers better understand and maintain the code. Use meaningful variable and function names: Give variables and functions
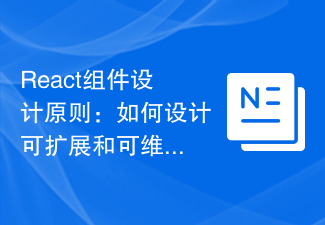
React component design principles: How to design scalable and maintainable front-end components Introduction: In modern front-end development, using the React framework to build componentized applications has become a mainstream development method. A well-designed React component can increase code reusability, scalability, and maintainability. This article will introduce some design principles to help developers design better React components. At the same time, we will provide some specific code examples to help readers understand better. 1. Single Responsibility Principle Single Responsibility Principle
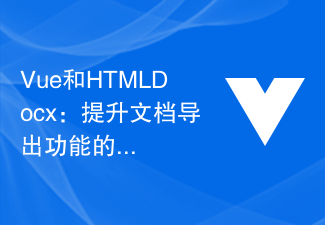
Vue and HTMLDocx: Improving the efficiency and scalability of the document export function Abstract: With the rapid development of information technology, the document export function is an essential part of many web applications. This article will introduce how to use Vue and HTMLDocx libraries to improve the efficiency and scalability of the document export function, and give code examples. Introduction: In today's digital era, we often need to implement document export functions in web applications. Whether exporting PDF documents, Word documents or other formats
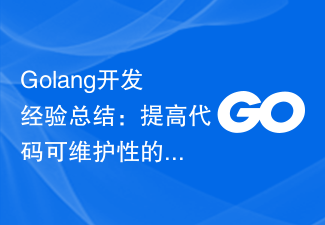
Golang is a fast, simple, and efficient programming language that is increasingly popular among developers. As a statically typed language, Golang has elegant syntax and powerful concurrency capabilities, allowing developers to easily build high-performance, scalable applications. However, as project size and code complexity increase, code maintainability becomes an important issue during the development process. In this article, I will share several tips to improve the maintainability of Golang code to help developers better manage and optimize their own

How PHP code specifications improve code scalability Introduction: When developing PHP applications, it is very important to write code that conforms to specifications. Following coding standards can improve code readability, maintainability, and scalability. This article will explore some practices of PHP code standards and show how to improve the scalability of the code through code examples. 1. Unified Naming Standards In the PHP development process, naming standards have an important impact on the readability and maintainability of the code. The following are some commonly used naming conventions: Class names should use camelCase naming
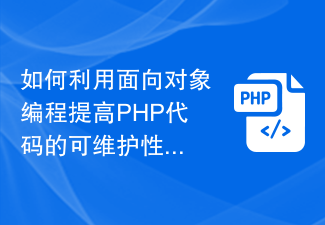
How to use object-oriented programming to improve the maintainability of PHP code Introduction: In the process of developing PHP projects, the maintainability of the code has always been the focus of developers. Maintainability refers to the readability, understandability and modifiability of the code after undergoing post-maintenance processes such as requirement changes, bug fixes and expanded functions. Object-oriented programming (OOP) is considered an effective method to improve code maintainability. This article will introduce how to use the
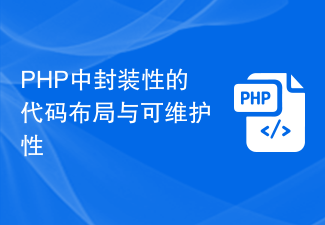
Encapsulated code layout and maintainability in PHP Encapsulation is an important concept in object-oriented programming. It can organize the code well, make the code modular and reusable, and improve the maintainability of the code. In PHP, encapsulated code layout and maintainability are one of the key issues that developers need to pay attention to. This article will explore how to improve the maintainability of PHP code through encapsulated code layout, and give specific code examples. Using namespaces for modularization In PHP, namespaces combine related classes and functions
