


Using RabbitMQ in Golang to implement performance optimization of distributed task queues
Golang is an open source programming language known for its efficient performance and concurrency. In distributed systems, task queues are a common task scheduling method. This article will introduce how to use RabbitMQ as a distributed task queue and provide some code examples for performance optimization.
1. Introduction to RabbitMQ
RabbitMQ is an open source message middleware based on AMQP protocol, which can implement reliable message delivery mechanism in distributed systems. Its main features include high concurrency, high reliability and flexible routing mechanism.
2. Basic concepts
- Producer: Producer, responsible for submitting tasks to RabbitMQ.
- Exchange: Exchange, responsible for distributing tasks to the corresponding queues.
- Queue: Task queue, which stores pending tasks.
- Consumer: Consumer, responsible for obtaining tasks from the queue and executing them.
3. Code example
The following is a simple code example using RabbitMQ to implement a distributed task queue:
package main import ( "fmt" "log" "github.com/streadway/amqp" ) func main() { // 连接到RabbitMQ服务器 conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { log.Fatalf("无法连接到RabbitMQ服务器:%s", err) } defer conn.Close() // 创建一个channel ch, err := conn.Channel() if err != nil { log.Fatalf("无法创建channel:%s", err) } defer ch.Close() // 声明一个队列 queue, err := ch.QueueDeclare( "task_queue", // 队列名称 true, // 是否持久化 false, // 是否自动删除 false, // 是否具有排他性 false, // 是否无等待 nil, // 额外参数 ) if err != nil { log.Fatalf("无法声明队列:%s", err) } // 发布任务 body := "Hello World!" err = ch.Publish( "", // 目标交换器 queue.Name, // 目标队列 false, // 是否为mandatory false, // 是否为immediate amqp.Publishing{ DeliveryMode: amqp.Persistent, // 消息持久化 ContentType: "text/plain", Body: []byte(body), }) if err != nil { log.Fatalf("无法发布任务:%s", err) } fmt.Println("任务已提交") }
4. Performance optimization suggestions
- Use connection pool: In order to improve performance and efficiency, you can use a connection pool to manage RabbitMQ connections. This reduces the overhead of establishing and disconnecting connections on each operation.
- Use multiple channels: Each channel has its own buffer and flow control mechanism. In a high-concurrency environment, using multiple channels can effectively improve throughput.
- Use batch submission and confirmation mechanism: In order to reduce network overhead, you can use batch submission and confirmation mechanism. Packaging multiple tasks into a batch of submissions can reduce the number of network IOs.
- Use the message prefetch mechanism: You can set the number of consumer prefetches and control the concurrency of tasks. This can improve throughput when task processing capabilities are strong.
In summary, using RabbitMQ as a distributed task queue can effectively improve the performance and reliability of the system. Through the rational use of connection pools, multiple channels, and optimized submission and confirmation mechanisms, the throughput of the system can be further improved. I hope these code examples and performance optimization suggestions will help you implement distributed task queues using RabbitMQ in Golang.
The above is the detailed content of Using RabbitMQ in Golang to implement performance optimization of distributed task queues. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


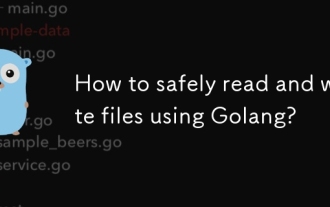
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
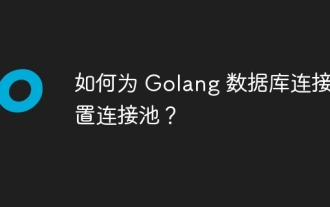
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
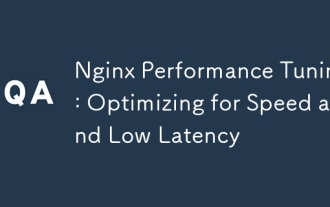
Nginx performance tuning can be achieved by adjusting the number of worker processes, connection pool size, enabling Gzip compression and HTTP/2 protocols, and using cache and load balancing. 1. Adjust the number of worker processes and connection pool size: worker_processesauto; events{worker_connections1024;}. 2. Enable Gzip compression and HTTP/2 protocol: http{gzipon;server{listen443sslhttp2;}}. 3. Use cache optimization: http{proxy_cache_path/path/to/cachelevels=1:2k
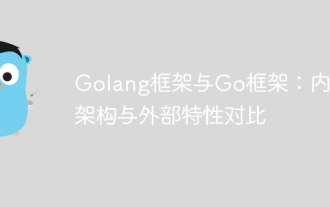
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
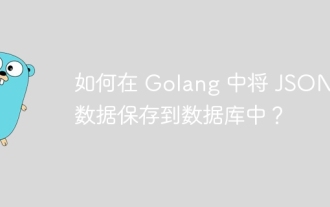
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
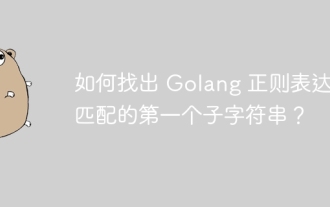
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
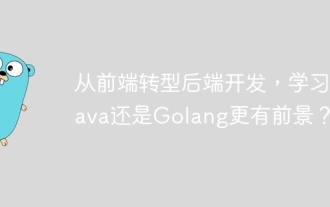
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
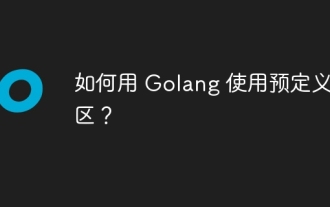
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
