


React Query Database Plugin: A way to achieve data archiving and recovery
React Query database plug-in: To implement data archiving and recovery methods, specific code examples are required
Introduction:
React Query is a powerful data management library. Can be used to manage remote data and local state in applications. It provides a set of easy-to-use APIs that can implement functions such as data acquisition, update, and caching. In addition to basic data management functions, React Query also supports plug-in extensions, allowing us to customize some functions according to specific needs. This article will introduce how to use the React Query database plug-in to implement data archiving and recovery, and provide specific code examples.
1. Introduction to React Query database plug-in
The React Query database plug-in is an extension library that allows us to store data in a local database to achieve data archiving and recovery functions. By using a database plug-in, we can store long-term data locally so that it can be quickly restored when the application reloads or an error occurs.
2. Install and configure the database plug-in
First, we need to use npm or yarn to install the React Query database plug-in. Open the terminal and execute the following command:
npm install react-query-db-plugin
or
yarn add react-query-db-plugin
After the installation is complete, we need to enable the database plug-in in the configuration of React Query. In the entry file of the application, such as index.js
or App.js
, add the following code:
import { QueryClient, QueryClientProvider } from 'react-query'; import { ReactQueryDBPlugin } from 'react-query-db-plugin'; const queryClient = new QueryClient(); // 创建数据库插件实例 const dbPlugin = new ReactQueryDBPlugin(); // 启用数据库插件 queryClient.use(dbPlugin); // 渲染应用程序 ReactDOM.render( <QueryClientProvider client={queryClient}> <App /> </QueryClientProvider>, document.getElementById('root') );
3. Data archiving and recovery methods
The database plug-in provides two main methods for archiving and restoring data: archiveData
and restoreData
. Below we will introduce these two methods in detail respectively.
- archiveData method
archiveData
method is used to archive data to a local database. We can selectively save data that needs long-term preservation to the database for future reloading or restoration.
import { useQueryClient } from 'react-query'; const ArchiveButton = () => { const queryClient = useQueryClient(); const handleArchiveData = () => { // 获取所有查询的数据 const data = queryClient.getQueryData(); // 将数据存储到数据库 queryClient.archiveData('myAppData', data); }; return ( <button onClick={handleArchiveData}>归档数据</button> ); };
In the above example, we first use the useQueryClient
hook function to obtain an instance of QueryClient, and then define an ArchiveButton
component. When the button is clicked , call the archiveData
method, and store all queried data in a database named 'myAppData'
.
- restoreData method
restoreData
method is used to restore previously archived data from the database. We can use this method when the app reloads or an error occurs to quickly restore previously saved data.
import { useQueryClient } from 'react-query'; const RestoreButton = () => { const queryClient = useQueryClient(); const handleRestoreData = async () => { // 从数据库中恢复数据 const data = await queryClient.restoreData('myAppData'); // 将数据设置为查询的数据 queryClient.setQueryData(data); }; return ( <button onClick={handleRestoreData}>恢复数据</button> ); };
In the above example, we also use the useQueryClient
hook function to obtain the instance of QueryClient, and then define a RestoreButton
component. When the button is clicked , call the restoreData
method, and set the data in 'myAppData'
database to the queried data.
4. Summary
The React Query database plug-in provides us with data archiving and recovery functions, allowing us to store data that needs to be saved for a long time locally and quickly when the application is reloaded or an error occurs. Data recovery. By installing and configuring the database plug-in, and using the archiveData
and restoreData
methods, we can easily archive and restore data. I hope this article can help you understand and use the React Query database plug-in!
Reference link:
- React Query official documentation: https://react-query.tanstack.com/
- React Query database plug-in GitHub repository: https: //github.com/react-query-db/react-query-db
The above is the detailed content of React Query Database Plugin: A way to achieve data archiving and recovery. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


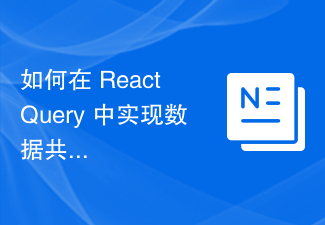
How to implement data sharing and permission management in ReactQuery? Advances in technology have made data management in front-end development more complex. In the traditional way, we may use state management tools such as Redux or Mobx to handle data sharing and permission management. However, after the emergence of ReactQuery, we can use it to deal with these problems more conveniently. In this article, we will explain how to implement data sharing and permissions in ReactQuery
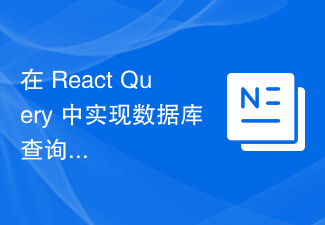
Implementing the error handling mechanism of database queries in ReactQuery ReactQuery is a library for managing and caching data, and it is becoming increasingly popular in the front-end field. In applications, we often need to interact with databases, and database queries may cause various errors. Therefore, implementing an effective error handling mechanism is crucial to ensure application stability and user experience. The first step is to install ReactQuery. Add it to the project using the following command: n
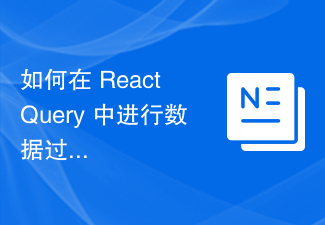
How to do data filtering and searching in ReactQuery? In the process of using ReactQuery for data management, we often encounter the need to filter and search data. These features can help us find and display data under specific conditions more easily. This article will introduce how to use filtering and search functions in ReactQuery and provide specific code examples. ReactQuery is a tool for querying data in React applications

Data Management with ReactQuery and Databases: A Best Practice Guide Introduction: In modern front-end development, managing data is a very important task. As users' demands for high performance and stability continue to increase, we need to consider how to better organize and manage application data. ReactQuery is a powerful and easy-to-use data management tool that provides a simple and flexible way to handle the retrieval, update and caching of data. This article will introduce how to use ReactQ
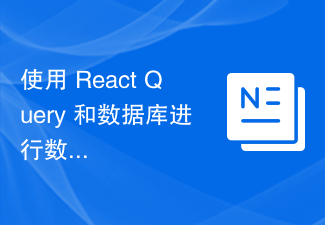
Introduction to data cache merging using ReactQuery and database: In modern front-end development, data management is a very important part. In order to improve performance and user experience, we usually need to cache the data returned by the server and merge it with local database data. ReactQuery is a very popular data caching library that provides a powerful API to handle data query, caching and updating. This article will introduce how to use ReactQuery and database
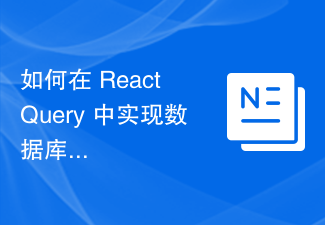
How to achieve separation of read and write in database in ReactQuery? In modern front-end development, the separation of reading and writing in the database is an important architectural design consideration. ReactQuery is a powerful state management library that can optimize the data acquisition and management process of front-end applications. This article will introduce how to use ReactQuery to achieve separation of read and write in the database, and provide specific code examples. The core concepts of ReactQuery are Query, Mutatio
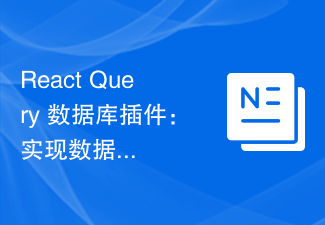
ReactQuery is a powerful data management library that provides many functions and features for working with data. When using ReactQuery for data management, we often encounter scenarios that require data deduplication and denoising. In order to solve these problems, we can use the ReactQuery database plug-in to achieve data deduplication and denoising functions in a specific way. In ReactQuery, you can use database plug-ins to easily process data
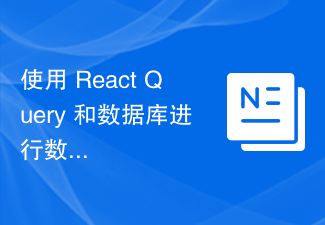
Title: Data Encryption and Decryption Using ReactQuery and Database Introduction: This article will introduce how to use ReactQuery and database for data encryption and decryption. We will use ReactQuery as the data management library and combine it with the database to perform data encryption and decryption operations. By combining these two technologies, we can securely store and transmit sensitive data, and perform encryption and decryption operations when needed to ensure data security. Text: 1. ReactQue
