


How to build real-time data processing applications using React and Apache Kafka
How to use React and Apache Kafka to build real-time data processing applications
Introduction:
With the rise of big data and real-time data processing, building real-time data processing applications It has become the pursuit of many developers. The combination of React, a popular front-end framework, and Apache Kafka, a high-performance distributed messaging system, can help us build real-time data processing applications. This article will introduce how to use React and Apache Kafka to build real-time data processing applications, and provide specific code examples.
1. Introduction to React Framework
React is a JavaScript library open sourced by Facebook, focusing on building user interfaces. React uses a component-based development method to divide the UI into independent and reusable structures, improving the maintainability and testability of the code. Based on the virtual DOM mechanism, React can efficiently update and render the user interface.
2. Introduction to Apache Kafka
Apache Kafka is a distributed, high-performance messaging system. Kafka is designed to handle large-scale data streams per second with high throughput, fault tolerance, and scalability. The core concept of Kafka is the publish-subscribe model, where producers publish messages to specific topics and consumers receive messages by subscribing to these topics.
3. Steps to build real-time data processing applications using React and Kafka
- Installing React and Kafka
First, we need to install the running environments of React and Kafka on the machine. React can be installed using npm, while Kafka requires downloading and configuring Zookeeper and Kafka server. -
Create React Project
Create a new React project using the React scaffolding tool create-react-app. Run the following command in the command line:npx create-react-app my-app cd my-app
Copy after login Install Kafka Library
Install Kafka-related libraries through npm for communicating with the Kafka server. Run the following command in the command line:npm install kafka-node
Copy after loginCreate Kafka producer
Create a kafkaProducer.js file in the React project for creating the Kafka producer and sending data to The specified topic. The following is a simple code example:const kafka = require('kafka-node'); const Producer = kafka.Producer; const client = new kafka.KafkaClient(); const producer = new Producer(client); producer.on('ready', () => { console.log('Kafka Producer is ready'); }); producer.on('error', (err) => { console.error('Kafka Producer Error:', err); }); const sendMessage = (topic, message) => { const payload = [ { topic: topic, messages: message } ]; producer.send(payload, (err, data) => { console.log('Kafka Producer sent:', data); }); }; module.exports = sendMessage;
Copy after loginCreate Kafka Consumer
Create a kafkaConsumer.js file in the React project for creating a Kafka consumer and consuming data from the specified topic Receive data. The following is a simple code example:const kafka = require('kafka-node'); const Consumer = kafka.Consumer; const client = new kafka.KafkaClient(); const consumer = new Consumer( client, [{ topic: 'my-topic' }], { autoCommit: false } ); consumer.on('message', (message) => { console.log('Kafka Consumer received:', message); }); consumer.on('error', (err) => { console.error('Kafka Consumer Error:', err); }); module.exports = consumer;
Copy after loginUsing Kafka in React components
Use the above Kafka producers and consumers in React components. The producer can be called in the component's lifecycle method to send data to the Kafka server, and the consumer can be used to obtain the data before rendering to the DOM. The following is a simple code example:import React, { Component } from 'react'; import sendMessage from './kafkaProducer'; import consumer from './kafkaConsumer'; class KafkaExample extends Component { componentDidMount() { // 发送数据到Kafka sendMessage('my-topic', 'Hello Kafka!'); // 获取Kafka数据 consumer.on('message', (message) => { console.log('Received Kafka message:', message); }); } render() { return ( <div> <h1>Kafka Example</h1> </div> ); } } export default KafkaExample;
Copy after loginIn the above code, the componentDidMount method will be automatically called after the component is rendered to the DOM. Here we send the first message and obtain the data through the consumer.
Run the React application
Finally, start the React application locally by running the following command:npm start
Copy after login
IV. Summary
This article Introduces how to use React and Apache Kafka to build real-time data processing applications. First, we briefly introduced the characteristics and functions of React and Kafka. We then provide specific steps to create a React project and create producers and consumers using Kafka related libraries. Finally, we show how to use these features in React components to achieve real-time data processing. Through these sample codes, readers can further understand and practice the combined application of React and Kafka, and build more powerful real-time data processing applications.
Reference materials:
- React official documentation: https://reactjs.org/
- Apache Kafka official documentation: https://kafka.apache.org /
The above is the detailed content of How to build real-time data processing applications using React and Apache Kafka. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


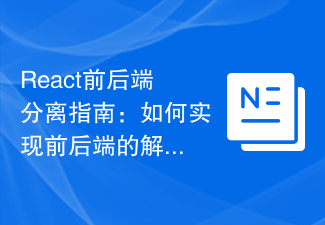
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
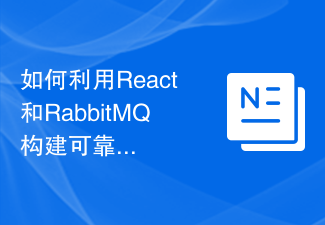
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
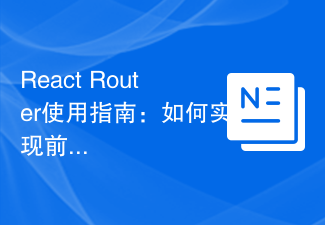
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
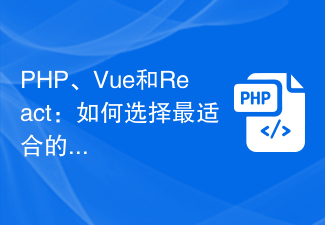
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
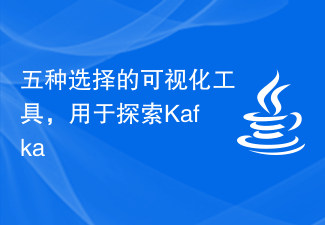
Five options for Kafka visualization tools ApacheKafka is a distributed stream processing platform capable of processing large amounts of real-time data. It is widely used to build real-time data pipelines, message queues, and event-driven applications. Kafka's visualization tools can help users monitor and manage Kafka clusters and better understand Kafka data flows. The following is an introduction to five popular Kafka visualization tools: ConfluentControlCenterConfluent
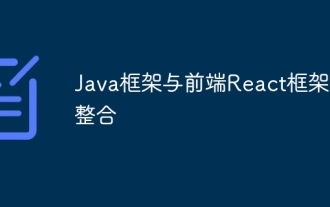
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.

How to choose the right Kafka visualization tool? Comparative analysis of five tools Introduction: Kafka is a high-performance, high-throughput distributed message queue system that is widely used in the field of big data. With the popularity of Kafka, more and more enterprises and developers need a visual tool to easily monitor and manage Kafka clusters. This article will introduce five commonly used Kafka visualization tools and compare their features and functions to help readers choose the tool that suits their needs. 1. KafkaManager

To install ApacheKafka on RockyLinux, you can follow the following steps: Update system: First, make sure your RockyLinux system is up to date, execute the following command to update the system package: sudoyumupdate Install Java: ApacheKafka depends on Java, so you need to install JavaDevelopmentKit (JDK) first ). OpenJDK can be installed through the following command: sudoyuminstalljava-1.8.0-openjdk-devel Download and decompress: Visit the ApacheKafka official website () to download the latest binary package. Choose a stable version
