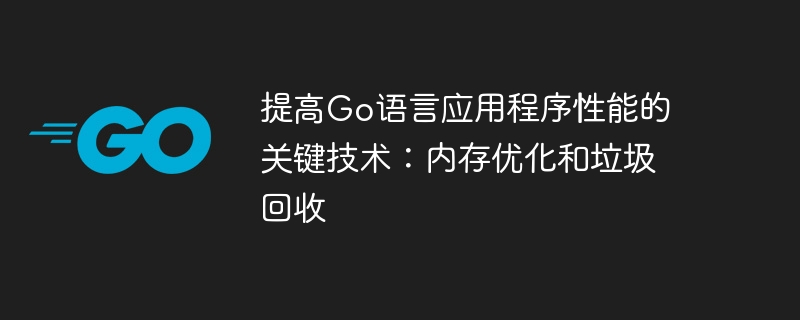
Key technologies to improve the performance of Go language applications: memory optimization and garbage collection
Abstract: With the popularity and widespread application of Go language, more and more Developers began to focus on how to improve the performance of Go applications. Among them, memory optimization and garbage collection are key technologies to improve the performance of Go applications. This article will introduce some memory optimization and garbage collection techniques, and give specific code examples to help readers better understand and apply these techniques.
1. Memory optimization technology
- Reduce memory allocation
The garbage collection mechanism of Go language provides us with the convenience of automatic memory management, but excessive memory allocation will cause Performance degrades. We can reduce memory allocation by using the following methods:
- Use fixed-size arrays or slices instead of dynamic-size data structures, such as using arrays instead of slices.
- Pre-allocate enough large slices to avoid dynamic expansion.
- Use sync.Pool to reuse temporary objects to avoid frequent application and release of memory.
- Avoid memory leaks
The garbage collection mechanism of the Go language can automatically recycle memory that is no longer used, but memory leaks may still occur. Common causes of memory leaks include:
- Circular references: When two or more objects refer to each other, if no other objects point to them, they will not be recycled by the garbage collector.
- Unclosed resources: such as open files, database connections, etc. If you forget to close them, memory leaks will occur. We should always promptly shut down resources that are no longer in use.
- Use pointers and reference types
In the Go language, using pointer types can reduce the number of memory copies and improve program performance. Especially in scenarios where a large amount of data is transferred and modified, using pointer types can reduce unnecessary memory overhead.
2. Garbage collection technology
- Use performance analysis tools
The Go language provides some performance analysis tools, such as pprof and trace. We can use these tools to identify and locate performance bottlenecks in our applications and optimize them accordingly. For example, we can use pprof to view memory allocation and garbage collection, and analyze the results to find code with high memory usage.
- Set appropriate garbage collection parameters
The garbage collector of the Go language has some parameters that can be adjusted, such as GOGC and GODEBUG. We can adjust these parameters according to application scenarios and needs to achieve better performance. For example, by adjusting the value of the GOGC parameter, the frequency of garbage collection can be reduced and the response speed of the application can be improved.
- Manually trigger garbage collection
In some scenarios, we need to manually trigger garbage collection to release unused memory in a timely manner. The runtime package of the Go language provides some functions, such as runtime.GC() and runtime.FreeOSMemory(), which can manually trigger garbage collection and operate the memory of the underlying operating system.
Specific code examples:
- Reduce memory allocation
package main
import (
"fmt"
"sync"
)
func main() {
slice := make([]int, 0, 100)
for i := 0; i < 100; i++ {
slice = append(slice, i)
}
// 使用sync.Pool重用临时对象
pool := &sync.Pool{
New: func() interface{} {
return make([]int, 0, 100)
},
}
for i := 0; i < 100; i++ {
tempSlice := pool.Get().([]int)
tempSlice = tempSlice[:0]
tempSlice = append(tempSlice, i)
fmt.Println(tempSlice)
pool.Put(tempSlice)
}
}
Copy after login
- Avoid memory leaks
package main
import (
"fmt"
"runtime"
"time"
)
func main() {
go func() {
for {
// 执行任务
time.Sleep(time.Second)
}
}()
// 等待程序退出
ch := make(chan struct{})
go func() {
for {
var memStat runtime.MemStats
runtime.ReadMemStats(&memStat)
fmt.Printf("HeapAlloc: %d
", memStat.HeapAlloc)
time.Sleep(time.Second)
}
}()
<-ch
}
Copy after login
- Set appropriate garbage collection parameters
package main
import (
"fmt"
"runtime"
"time"
)
func main() {
fmt.Println("GOGC:", runtime.GOGC)
// 设置GOGC的值为100
runtime.SetGCPercent(100)
fmt.Println("GOGC:", runtime.GOGC)
go func() {
var memStat runtime.MemStats
for {
runtime.ReadMemStats(&memStat)
fmt.Printf("HeapAlloc: %d
", memStat.HeapAlloc)
time.Sleep(time.Second)
}
}()
select {}
}
Copy after login
Conclusion:
By understanding and applying memory optimization and garbage collection technology for Go language applications, we can significantly improve the performance of the application. performance. This article introduces some key technologies of memory optimization and garbage collection, and gives specific code examples, which readers can refer to and apply according to actual needs. By using these technologies appropriately, we can make Go applications run faster and more stably.
The above is the detailed content of Key technologies to improve the performance of Go language applications: memory optimization and garbage collection. For more information, please follow other related articles on the PHP Chinese website!