


Golang RabbitMQ: Architectural design for reliable messaging and system monitoring
Golang RabbitMQ: Architectural design to achieve reliable message delivery and system monitoring
Introduction:
In distributed systems, message delivery is a common problem. In order to ensure reliable delivery of messages, we need a reliable message queue system. In this article, we will use Golang and RabbitMQ to implement an architectural design for reliable messaging and system monitoring. We will discuss the basic concepts of message queues, how to use RabbitMQ and Golang for messaging, and how to monitor the entire system.
1. The basic concept of message queue
Message queue is a mechanism used to implement asynchronous communication in distributed systems. It consists of message producers and message consumers, which communicate through an intermediate message queue. Message queues can ensure reliable delivery of messages and can handle high-concurrency message processing.
Message queue has the following basic concepts:
- Message producer (Producer): Responsible for generating messages and sending them to the message queue.
- Message Queue (Queue): Responsible for storing messages and sending them to message consumers one by one.
- Message Consumer (Consumer): Responsible for obtaining messages from the message queue and processing them.
2. Using RabbitMQ and Golang for message delivery
RabbitMQ is an open source message queue system that supports multiple message protocols and provides an easy-to-use client library. The following are the steps for using RabbitMQ and Golang for messaging:
Step 1: Install RabbitMQ
First, you need to install RabbitMQ. For specific installation steps, you can refer to the official documentation (https://www.rabbitmq.com/) or search for related tutorials.
Step 2: Create a message producer
The following is a simple Golang code example for creating a message producer and sending messages to the RabbitMQ queue:
package main import ( "log" "github.com/streadway/amqp" ) func main() { conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { log.Fatalf("Failed to connect to RabbitMQ: %s", err) } defer conn.Close() ch, err := conn.Channel() if err != nil { log.Fatalf("Failed to open a channel: %s", err) } defer ch.Close() q, err := ch.QueueDeclare( "my_queue", // 队列名称 false, // 队列持久化 false, // 随服务器启动而创建 false, // 自动删除队列 false, // 不使用额外的属性 nil, // 额外属性 ) if err != nil { log.Fatalf("Failed to declare a queue: %s", err) } body := "Hello, RabbitMQ!" err = ch.Publish( "", // exchange q.Name, // routing key false, // mandatory false, // immediate amqp.Publishing{ ContentType: "text/plain", Body: []byte(body), }) if err != nil { log.Fatalf("Failed to publish a message: %s", err) } }
Step 3: Create a message consumer
The following is a simple Golang code example for creating a message consumer and getting messages from the RabbitMQ queue:
package main import ( "log" "os" "os/signal" "syscall" "time" "github.com/streadway/amqp" ) func main() { conn, err := amqp.Dial("amqp://guest:guest@localhost:5672/") if err != nil { log.Fatalf("Failed to connect to RabbitMQ: %s", err) } defer conn.Close() ch, err := conn.Channel() if err != nil { log.Fatalf("Failed to open a channel: %s", err) } defer ch.Close() q, err := ch.QueueDeclare( "my_queue", // 队列名称 false, // 队列持久化 false, // 随服务器启动而创建 false, // 自动删除队列 false, // 不使用额外的属性 nil, // 额外属性 ) if err != nil { log.Fatalf("Failed to declare a queue: %s", err) } msgs, err := ch.Consume( q.Name, // 队列名称 "", // 消费者标识符 true, // 自动回复消息确认 false, // 独占队列 false, // 不等待服务器响应 false, // 不使用额外的属性 nil, // 额外属性 ) if err != nil { log.Fatalf("Failed to register a consumer: %s", err) } // 处理消息 go func() { for d := range msgs { log.Printf("Received a message: %s", d.Body) } }() // 等待退出信号 sigs := make(chan os.Signal, 1) signal.Notify(sigs, syscall.SIGINT, syscall.SIGTERM) <-sigs log.Println("Exiting...") time.Sleep(1 * time.Second) }
3. Implementing reliable message delivery
RabbitMQ provides Message persistence mechanism ensures that even in the event of a failure or power outage, messages are saved and sent after recovery. The following is some sample code for achieving reliable messaging:
Message producer:
// 设置消息持久化 err = ch.Publish( "", q.Name, true, false, amqp.Publishing{ DeliveryMode: amqp.Persistent, ContentType: "text/plain", Body: []byte(body), })
Message consumer:
msg.Ack(false)
4. System monitoring
Provided by RabbitMQ It provides many tools and interfaces for monitoring and managing the running status of message queues. The following are some commonly used system monitoring methods:
- RabbitMQ management plug-in: monitor and manage RabbitMQ through the web interface. The RabbitMQ management plug-in can be enabled by running the
rabbitmq-plugins enable rabbitmq_management
command. - Prometheus and Grafana: Prometheus is an open source monitoring system and time series database, and Grafana is an open source data visualization tool. You can use Prometheus to collect RabbitMQ monitoring data, and use Grafana to display and analyze the data.
- RabbitMQ Exporter: It is a Prometheus Exporter, used to collect RabbitMQ monitoring data and expose it to Prometheus.
Conclusion:
This article introduces how to use Golang and RabbitMQ to achieve reliable messaging and system monitoring architecture design. We discussed the basic concepts of message queues, how to use RabbitMQ and Golang for messaging, and how to achieve reliable messaging and system monitoring. I hope this article is helpful to readers and can be used in practical applications.
The above is the detailed content of Golang RabbitMQ: Architectural design for reliable messaging and system monitoring. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


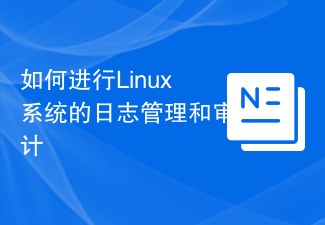
Overview of how to perform log management and auditing in Linux systems: In Linux systems, log management and auditing are very important. Through correct log management and auditing strategies, the operation of the system can be monitored in real time, problems can be discovered in a timely manner and corresponding measures can be taken. This article will introduce how to perform log management and auditing on Linux systems, and provide some specific code examples for reference. 1. Log management 1.1 Location and naming rules of log files In Linux systems, log files are usually located in the /var/log directory.
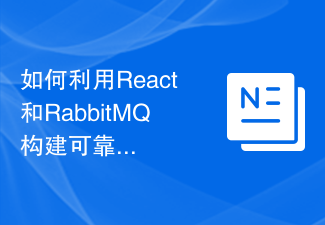
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
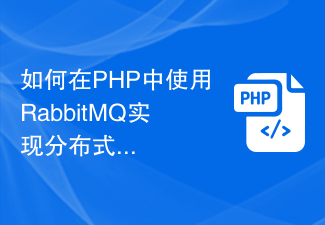
How to use RabbitMQ to implement distributed message processing in PHP Introduction: In large-scale application development, distributed systems have become a common requirement. Distributed message processing is a pattern that improves the efficiency and reliability of the system by distributing tasks to multiple processing nodes. RabbitMQ is an open source, reliable message queuing system that uses the AMQP protocol to implement message delivery and processing. In this article we will cover how to use RabbitMQ in PHP for distribution
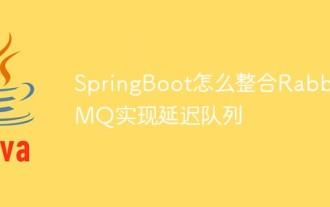
How to ensure that messages are not lost. The rabbitmq message delivery path producer->switch->queue->consumer is generally divided into three stages. 1. The producer ensures the reliability of message delivery. 2.MQ internal messages are not lost. 3. Consumer consumption is successful. What is message delivery reliability? Simply put, it means that messages are 100% sent to the message queue. We can turn on confirmCallback. After the producer delivers the message, mq will give the producer an ack. Based on the ack, the producer can confirm whether the message is sent to mq. Turn on confirmCallback and modify the configuration file #NONE: disable the release confirmation mode, which is the default value , CORRELATED:
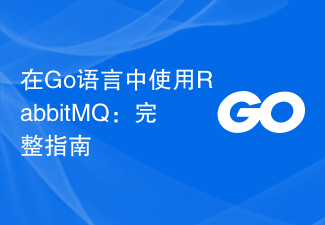
As modern applications increase in complexity, messaging has become a powerful tool. In this area, RabbitMQ has become a very popular message broker that can be used to deliver messages between different applications. In this article, we will explore how to use RabbitMQ in Go language. This guide will cover the following: Introduction to RabbitMQ RabbitMQ Installation RabbitMQ Basic Concepts Getting Started with RabbitMQ in Go RabbitMQ and Go
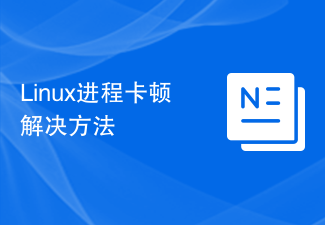
How to solve the process lag problem in Linux systems When we use the Linux operating system, we sometimes encounter process lags, which brings inconvenience to our work and use. Process lag may be caused by various reasons, such as insufficient resources, deadlock, IO blocking, etc. In this article, we will discuss some methods and techniques to solve the process stuck problem. First, we need to identify the cause of process lag. You can find the problem in the following ways: Use system monitoring tools: You can use tools like top,
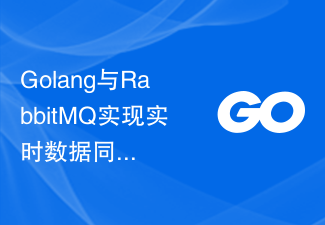
Introduction to the solution for real-time data synchronization between Golang and RabbitMQ: In today's era, with the popularity of the Internet and the explosive growth of data volume, real-time data synchronization has become more and more important. In order to solve the problems of asynchronous data transmission and data synchronization, many companies have begun to use message queues to achieve real-time synchronization of data. This article will introduce a real-time data synchronization solution based on Golang and RabbitMQ, and provide specific code examples. 1. What is RabbitMQ? Rabbi
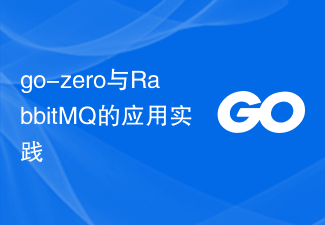
Now more and more companies are beginning to adopt the microservice architecture model, and in this architecture, message queues have become an important communication method, among which RabbitMQ is widely used. In the Go language, go-zero is a framework that has emerged in recent years. It provides many practical tools and methods to allow developers to use message queues more easily. Below we will introduce go-zero based on practical applications. And the usage and application practice of RabbitMQ. 1.RabbitMQ OverviewRabbit
