High concurrent database access: Go WaitGroup technology on Golang
High concurrent database access: Go WaitGroup technology on Golang
Introduction:
In today's Internet era, concurrent access to the database is a very important issue . As the number of users increases, the system needs to be able to handle requests from multiple users at the same time, and database access is often one of the efficiency bottlenecks. In order to solve this problem, this article will introduce the use of Go WaitGroup technology in Go language to achieve high concurrent database access. At the same time, specific code examples will be provided so that readers can better understand and apply this technology.
- Challenges of concurrent database access
In traditional single-threaded database access, each request is processed sequentially. When the number of system users increases, the waiting time for requests will also become longer, resulting in a decrease in system performance. In order to solve this problem, requests can be processed concurrently to improve the concurrency of the system. However, since database access is a resource competition issue, thread safety needs to be ensured, otherwise data errors or system crashes will result. - Go WaitGroup technology in Go language
Go language provides a convenient concurrency primitive Go WaitGroup, which can be used to control the execution of concurrent threads. WaitGroup maintains a counter internally to record the number of threads that need to wait. When a thread starts executing, the counter is incremented by 1; when a thread completes execution, the counter is decremented by 1. Only when the counter reaches 0 can all threads continue execution. This ensures that all concurrent requests are processed correctly. - Sample code for high concurrent database access
Now let us use an example to demonstrate how to use Go WaitGroup technology to achieve high concurrent database access in the Go language.
package main import ( "database/sql" "fmt" "sync" ) func main() { db, err := sql.Open("mysql", "username:password@tcp(127.0.0.1:3306)/mydb") if err != nil { fmt.Println("Failed to connect to the database:", err) return } defer db.Close() wg := sync.WaitGroup{} for i := 0; i < 100; i++ { wg.Add(1) go func(id int) { defer wg.Done() // 执行数据库查询或插入操作 // ... }(i) } wg.Wait() fmt.Println("All queries completed") }
In the above code, we use the sync.WaitGroup type wg to track all concurrent goroutines. Within the loop, we increment the counter by calling wg.Add(1) and perform database operations in a new goroutine using the go keyword. After each goroutine is executed, the counter value is decremented by calling wg.Done().
In this way, we can start multiple goroutines at the same time and wait for all goroutines to be executed before continuing. This achieves high concurrent database access.
Conclusion:
This article introduces the method of using Go WaitGroup technology to achieve high concurrent database access in Go language. We illustrate the challenges of concurrent database access and show specific Go language example code. By using Go WaitGroup technology, we can easily control the execution of concurrent threads, thereby improving the concurrency and performance of the system.
It is worth mentioning that the sample code in this article is for reference only and cannot be used directly in a production environment. In practical applications, other factors such as connection pools, retry mechanisms, error handling, etc. also need to be considered. We hope that readers can gain an in-depth understanding of Go WaitGroup technology through the introduction of this article and apply it to their actual projects to achieve more efficient database access.
The above is the detailed content of High concurrent database access: Go WaitGroup technology on Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


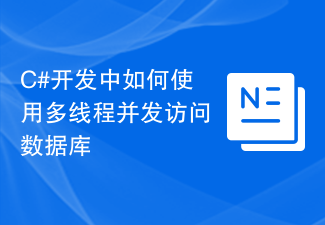
How to use multi-threads to access the database concurrently in C# development In C# development, multi-threads concurrent access to the database is a common requirement. Using multi-threading can improve the efficiency of database operations, but you also need to pay attention to issues such as thread safety and database connection management. This article will introduce how to use multi-threading to access the database concurrently in C# and provide specific code examples. Create a database connection Before using multi-threads to access the database concurrently, you first need to create a database connection. Normally, we use Sql provided by ADO.NET
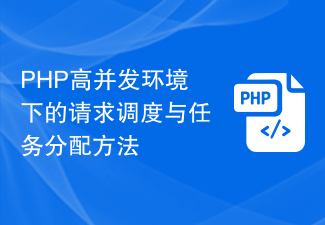
Request scheduling and task allocation methods in PHP high-concurrency environment With the rapid development of the Internet, PHP, as a widely used back-end development language, is facing more and more high-concurrency requests. In a high-concurrency environment, how to implement request scheduling and task allocation has become an important issue that needs to be solved during development. This article will introduce some request scheduling and task allocation methods in PHP high concurrency environment, and provide code examples. 1. Process management and task queue In PHP high concurrency environment, process management and task queue are commonly used implementation methods.
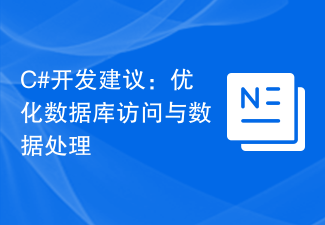
C# development suggestions: Optimize database access and data processing In modern software development, database access and data processing are an indispensable part. Especially in C# development, optimizing database access and data processing is the key to improving software performance and user experience. This article will discuss database access and data processing optimization in C# development, and provide developers with better guidance and suggestions. 1. Use appropriate database access technology. In C# development, common database access technologies include ADO.NET, EntityFr
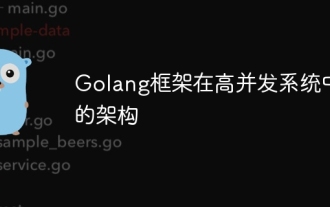
For high-concurrency systems, the Go framework provides architectural modes such as pipeline mode, Goroutine pool mode, and message queue mode. In practical cases, high-concurrency websites use Nginx proxy, Golang gateway, Goroutine pool and database to handle a large number of concurrent requests. The code example shows the implementation of a Goroutine pool for handling incoming requests. By choosing appropriate architectural patterns and implementations, the Go framework can build scalable and highly concurrent systems.
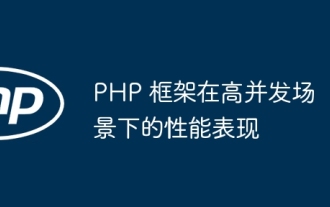
In high-concurrency scenarios, according to benchmark tests, the performance of the PHP framework is: Phalcon (RPS2200), Laravel (RPS1800), CodeIgniter (RPS2000), and Symfony (RPS1500). Actual cases show that the Phalcon framework achieved 3,000 orders per second during the Double Eleven event on the e-commerce website.

[Title] Highly concurrent TCP long connection processing techniques for Swoole development functions [Introduction] With the rapid development of the Internet, applications have increasingly higher demands for concurrent processing. As a high-performance network communication engine based on PHP, Swoole provides powerful asynchronous, multi-process, and coroutine capabilities, which greatly improves the concurrent processing capabilities of applications. This article will introduce how to use the Swoole development function to handle high-concurrency TCP long connection processing techniques, and provide detailed explanations with code examples. 【Text】1. Swo
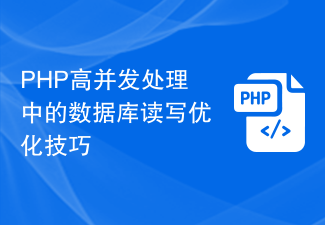
Database reading and writing optimization techniques in PHP high concurrency processing With the rapid development of the Internet, the growth of website visits has become higher and higher. In today's Internet applications, high concurrency processing has become a problem that cannot be ignored. In PHP development, database read and write operations are one of the performance bottlenecks. Therefore, in high-concurrency scenarios, it is very important to optimize database read and write operations. The following will introduce some database read and write optimization techniques in PHP high concurrency processing, and give corresponding code examples. Using connection pooling technology to connect to the database will
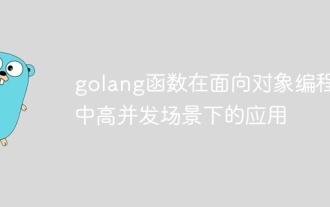
In high-concurrency scenarios of object-oriented programming, functions are widely used in the Go language: Functions as methods: Functions can be attached to structures to implement object-oriented programming, conveniently operating structure data and providing specific functions. Functions as concurrent execution bodies: Functions can be used as goroutine execution bodies to implement concurrent task execution and improve program efficiency. Function as callback: Functions can be passed as parameters to other functions and be called when specific events or operations occur, providing a flexible callback mechanism.
