How to filter and search data in React Query?
How to filter and search data in React Query?
In the process of using React Query for data management, we often encounter the need to filter and search data. These features can help us find and display data under specific conditions more easily. This article explains how to use filtering and searching in React Query and provides specific code examples.
React Query is a library for data management in React applications. It provides some powerful functions to help us manage and cache data more conveniently. Among them, by using QueryKeys, you can define different query keys to operate on different data.
The key to implementing data filtering and searching in React Query is to use QueryKeys to dynamically create query keys. In this way, we can define different query keys to adapt to data filtering and searching under different conditions.
First, we need to define a query key that contains all data. For example, we can use "users" as the query key to get data for all users.
const queryClient = new QueryClient(); function App() { return ( <QueryClientProvider client={queryClient}> <UserList /> </QueryClientProvider> ); } function UserList() { const { data } = useQuery("users", fetchUsers); return ( <div> {data.map((user) => ( <UserCard key={user.id} user={user} /> ))} </div> ); }
In the above code, we use the useQuery
hook to obtain all user data and display it on the page.
Next, we need to define a query key required for the filter or search function. For example, we can use "filteredUsers" as a query key to get user data that meets a certain condition.
function UserFilter() { const [filter, setFilter] = useState(""); const { data } = useQuery( ["filteredUsers", filter], () => fetchFilteredUsers(filter), { enabled: Boolean(filter), } ); return ( <div> <input type="text" value={filter} onChange={(e) => setFilter(e.target.value)} /> {data && data.length > 0 ? ( <div> {data.map((user) => ( <UserCard key={user.id} user={user} /> ))} </div> ) : ( <div>No matching users</div> )} </div> ); }
In the above code, we use the useState
hook to define the state of a filter condition. Then, we use the useQuery
hook to obtain user data that meets the filter conditions and display it on the page. We use an array as the query key, where the first element is the name of the query key and the second element is the filter condition. When the filter criteria is empty, we disable the query to avoid unnecessary requests.
In practical applications, we can freely define filtering conditions according to specific needs and use different query keys according to different scenarios.
The above are the basic methods for data filtering and searching in React Query. Through the flexible use of query keys, we can easily implement data filtering and search functions. This flexibility makes React Query a powerful data management tool.
I hope this article can help you implement data filtering and search functions in React Query. If you have any questions or suggestions, please leave a message below to discuss with us.
The above is the detailed content of How to filter and search data in React Query?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


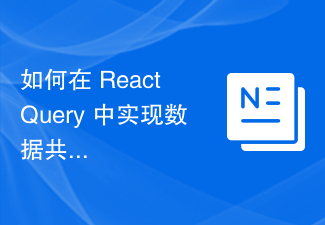
How to implement data sharing and permission management in ReactQuery? Advances in technology have made data management in front-end development more complex. In the traditional way, we may use state management tools such as Redux or Mobx to handle data sharing and permission management. However, after the emergence of ReactQuery, we can use it to deal with these problems more conveniently. In this article, we will explain how to implement data sharing and permissions in ReactQuery
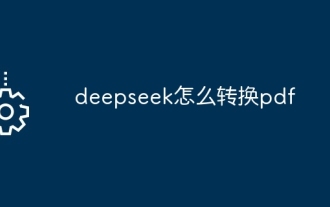
DeepSeek cannot convert files directly to PDF. Depending on the file type, you can use different methods: Common documents (Word, Excel, PowerPoint): Use Microsoft Office, LibreOffice and other software to export as PDF. Image: Save as PDF using image viewer or image processing software. Web pages: Use the browser's "Print into PDF" function or the dedicated web page to PDF tool. Uncommon formats: Find the right converter and convert it to PDF. It is crucial to choose the right tools and develop a plan based on the actual situation.
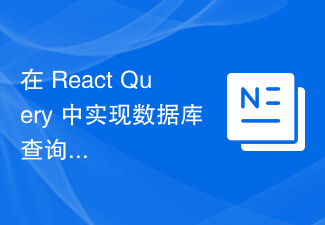
Implementing the error handling mechanism of database queries in ReactQuery ReactQuery is a library for managing and caching data, and it is becoming increasingly popular in the front-end field. In applications, we often need to interact with databases, and database queries may cause various errors. Therefore, implementing an effective error handling mechanism is crucial to ensure application stability and user experience. The first step is to install ReactQuery. Add it to the project using the following command: n
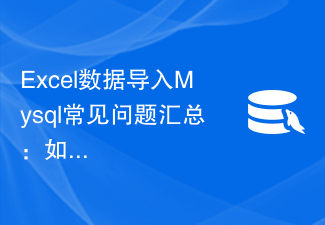
Summary of frequently asked questions about importing Excel data into Mysql: How to deal with duplicate data during the import process? In the process of data processing, we often encounter the need to import Excel data into the Mysql database. However, due to the huge amount of data, it is easy to duplicate data, which requires us to handle it accordingly during the import process. In this article, we discuss how to handle duplicate data during import and provide corresponding code examples. Before performing repeated data processing, you first need to ensure that there are unique
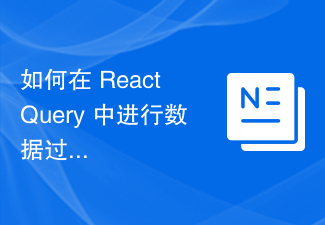
How to do data filtering and searching in ReactQuery? In the process of using ReactQuery for data management, we often encounter the need to filter and search data. These features can help us find and display data under specific conditions more easily. This article will introduce how to use filtering and search functions in ReactQuery and provide specific code examples. ReactQuery is a tool for querying data in React applications

Data Management with ReactQuery and Databases: A Best Practice Guide Introduction: In modern front-end development, managing data is a very important task. As users' demands for high performance and stability continue to increase, we need to consider how to better organize and manage application data. ReactQuery is a powerful and easy-to-use data management tool that provides a simple and flexible way to handle the retrieval, update and caching of data. This article will introduce how to use ReactQ
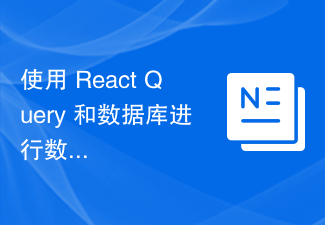
Introduction to data cache merging using ReactQuery and database: In modern front-end development, data management is a very important part. In order to improve performance and user experience, we usually need to cache the data returned by the server and merge it with local database data. ReactQuery is a very popular data caching library that provides a powerful API to handle data query, caching and updating. This article will introduce how to use ReactQuery and database
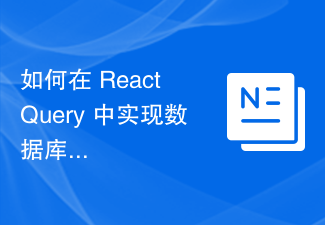
How to achieve separation of read and write in database in ReactQuery? In modern front-end development, the separation of reading and writing in the database is an important architectural design consideration. ReactQuery is a powerful state management library that can optimize the data acquisition and management process of front-end applications. This article will introduce how to use ReactQuery to achieve separation of read and write in the database, and provide specific code examples. The core concepts of ReactQuery are Query, Mutatio
