How to use Golang Facade to implement concise interface calls
How to use Golang Facade to implement concise interface calling
Introduction:
In software development, interface calling is a very common requirement. When developing with Golang, we can use the Facade mode to simplify the complexity of interface calls and improve the readability and maintainability of the code. This article will introduce how to use Golang Facade to implement concise interface calls and provide specific code examples.
1. What is Facade mode?
Facade pattern is a structural design pattern that aims to provide a unified interface to simplify the use of a complex set of subsystems. The Facade pattern hides the complexity of the subsystem and provides a simple interface for the client, thereby reducing the coupling of the system.
2. Why use Facade mode?
When there are multiple complex subsystems in our system and we want to provide a simple interface to the outside world, we can consider using the Facade mode. By encapsulating the complexity of subsystems, the Facade pattern can reduce coupling within the system and provide a simple interface for clients to use, while also facilitating subsequent maintenance and expansion.
3. How to use Golang Facade to implement concise interface calls?
Below we use a scenario to illustrate how to use Golang Facade to implement concise interface calls.
Scenario:
Suppose there are three complex subsystems in our system: A, B and C. And we hope to provide a unified interface to the outside world to use these three subsystems.
First, we need to define a Facade structure to encapsulate the complexity of the subsystem and provide a simple interface for the client to use. The code is as follows:
// Facade 结构体 type Facade struct { subsystemA *SubsystemA subsystemB *SubsystemB subsystemC *SubsystemC } // 创建一个Facade实例 func NewFacade() *Facade { return &Facade{ subsystemA: NewSubsystemA(), subsystemB: NewSubsystemB(), subsystemC: NewSubsystemC(), } } // Facade接口方法 func (f *Facade) Operation1() { f.subsystemA.OperationA() f.subsystemB.OperationB() } func (f *Facade) Operation2() { f.subsystemB.OperationB() f.subsystemC.OperationC() }
Next, we need to define the structures of the three subsystems and implement their respective methods. The code is as follows:
// 子系统A type SubsystemA struct { // 属性 } // 创建子系统A实例 func NewSubsystemA() *SubsystemA { return &SubsystemA{} } // 子系统A方法 func (s *SubsystemA) OperationA() { fmt.Println("Subsystem A: Operation A") } // 子系统B type SubsystemB struct { // 属性 } // 创建子系统B实例 func NewSubsystemB() *SubsystemB { return &SubsystemB{} } // 子系统B方法 func (s *SubsystemB) OperationB() { fmt.Println("Subsystem B: Operation B") } // 子系统C type SubsystemC struct { // 属性 } // 创建子系统C实例 func NewSubsystemC() *SubsystemC { return &SubsystemC{} } // 子系统C方法 func (s *SubsystemC) OperationC() { fmt.Println("Subsystem C: Operation C") }
Finally, we can use these three subsystems by creating Facade instances and call the simple interface provided by Facade. The code is as follows:
func main() { facade := NewFacade() // 调用Facade接口方法 facade.Operation1() facade.Operation2() }
Run the above code, the output result is as follows:
Subsystem A: Operation A Subsystem B: Operation B Subsystem B: Operation B Subsystem C: Operation C
Through the above code example, we can see that using the Golang Facade mode can easily implement concise interface calls. As an intermediate layer, the Facade structure encapsulates the complexity of the subsystem and provides a simple interface for the client, making system calls more intuitive and convenient.
Conclusion:
This article introduces how to use the Golang Facade mode to implement concise interface calls and provides specific code examples. By using the Facade pattern, we can hide the complexity of the subsystem, improve the readability and maintainability of the code, and also facilitate the maintenance and expansion of the system. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of How to use Golang Facade to implement concise interface calls. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


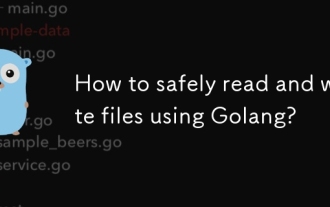
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
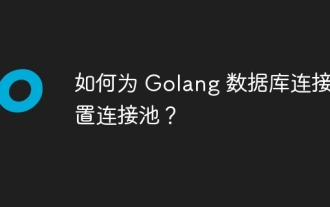
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
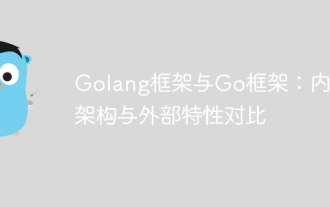
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
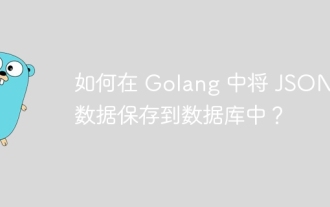
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
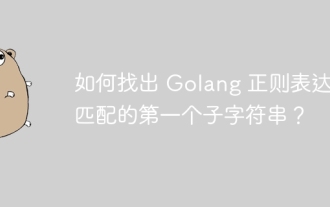
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
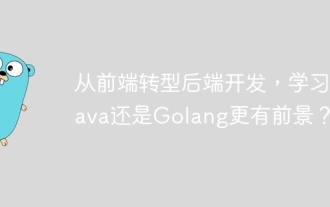
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
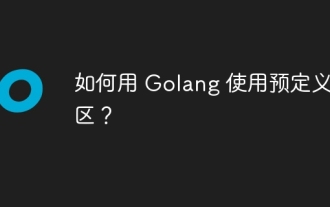
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
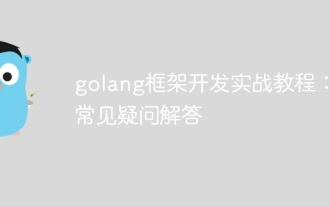
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
