How to implement a simple blog version 2.0 using PHP
How to use PHP to implement a simple blog version 2.0
Overview:
In the Internet era, blogging has become a very popular way of expressing and recording life Tool of. PHP is a commonly used server-side scripting language when developing a blogging application. It can be used to handle user requests, generate dynamic pages, interact with databases, etc. This article will introduce how to use PHP to implement a simple blog version 2.0 and give specific code examples.
Step 1: Database design
First, we need to design a database to store blog-related information, including the title, content, creation time, etc. of the article. The following is a simple database design example:
CREATE TABLE `blog` ( `id` int(11) NOT NULL AUTO_INCREMENT, `title` varchar(255) NOT NULL, `content` text NOT NULL, `create_time` datetime NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
Step 2: Create a database connection
Use PHP's PDO extension to connect to the database and encapsulate it into a singleton class for convenience throughout the application Reuse. The following is an example of a simple database connection class:
class Database { private static $instance = null; private $conn; private function __construct() { $dbHost = 'localhost'; $dbName = 'blog_db'; $dbUser = 'root'; $dbPass = 'password'; $this->conn = new PDO("mysql:host=$dbHost;dbname=$dbName", $dbUser, $dbPass); } public static function getInstance() { if (!self::$instance) { self::$instance = new Database(); } return self::$instance; } public function getConnection() { return $this->conn; } }
Step 3: Implement the blog’s add, delete, modify, and check function
Next, we need to implement the blog’s add, delete, modify, and check function. Here we will use PHP object-oriented programming to encapsulate the blog operation class. The following is an example of a simple blog operation class:
class Blog { private $db; public function __construct() { $this->db = Database::getInstance()->getConnection(); } public function create($title, $content) { $stmt = $this->db->prepare("INSERT INTO blog (title, content, create_time) VALUES (:title, :content, NOW())"); $stmt->bindParam(':title', $title); $stmt->bindParam(':content', $content); $stmt->execute(); } public function update($id, $title, $content) { $stmt = $this->db->prepare("UPDATE blog SET title = :title, content = :content WHERE id = :id"); $stmt->bindParam(':id', $id); $stmt->bindParam(':title', $title); $stmt->bindParam(':content', $content); $stmt->execute(); } public function delete($id) { $stmt = $this->db->prepare("DELETE FROM blog WHERE id = :id"); $stmt->bindParam(':id', $id); $stmt->execute(); } public function getById($id) { $stmt = $this->db->prepare("SELECT * FROM blog WHERE id = :id"); $stmt->bindParam(':id', $id); $stmt->execute(); return $stmt->fetch(PDO::FETCH_ASSOC); } public function getAll() { $stmt = $this->db->prepare("SELECT * FROM blog ORDER BY create_time DESC"); $stmt->execute(); return $stmt->fetchAll(PDO::FETCH_ASSOC); } }
Step 4: Write the front-end page
Finally, we need to write the front-end page to display the content of the blog. Here we use HTML, CSS and Bootstrap to build the blog interface. The following is a simple example page:
<!DOCTYPE html> <html> <head> <title>Simple Blog 2.0</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css"> </head> <body> <div class="container"> <h1 id="My-Blog">My Blog</h1> <h2 id="Create-New-Post">Create New Post</h2> <form action="create.php" method="post"> <div class="form-group"> <label for="title">Title:</label> <input type="text" class="form-control" id="title" name="title"> </div> <div class="form-group"> <label for="content">Content:</label> <textarea class="form-control" id="content" name="content"></textarea> </div> <button type="submit" class="btn btn-primary">Submit</button> </form> <h2 id="All-Posts">All Posts</h2> <ul> <?php $blog = new Blog(); $posts = $blog->getAll(); foreach ($posts as $post) { echo "<li><a href='view.php?id=".$post['id']."'>".$post['title']."</a></li>"; } ?> </ul> </div> </body> </html>
Summary:
Through the above steps, we used PHP to implement a simple blog version 2.0, including database design, database connection, blog addition, deletion, modification and query functions, and front-end Page writing. Of course, this is just a simple example and you can extend and optimize it according to your actual needs. I hope this article can help you understand how to use PHP to develop blog applications.
The above is the detailed content of How to implement a simple blog version 2.0 using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


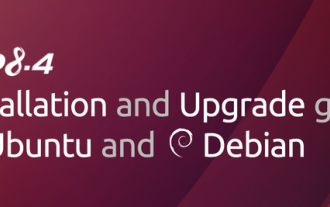
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
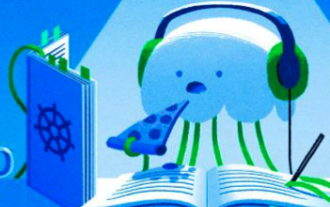
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
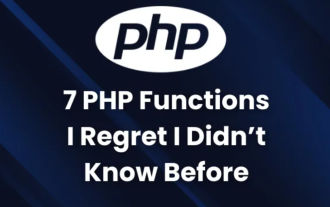
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
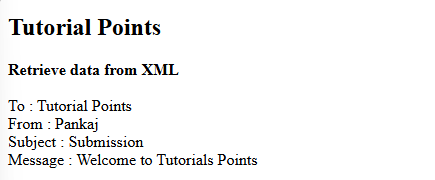
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
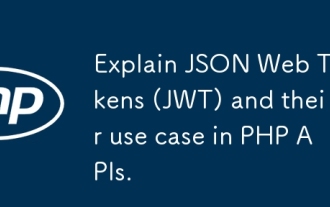
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
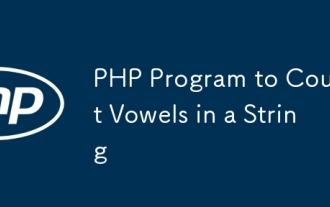
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
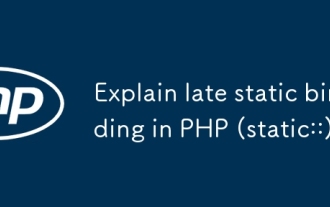
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
