How to use Go WaitGroup to handle concurrent tasks
How to use Go WaitGroup to handle concurrent tasks
In the Go language, we can handle concurrent tasks by using sync.WaitGroup
. sync.WaitGroup
can provide a concise and effective way to coordinate the execution of coroutines when handling concurrent tasks.
sync.WaitGroup
is a useful tool and is the preferred method for handling concurrent tasks when we don't know how many coroutines need to wait. It allows us to ensure that the main coroutine does not end execution until all tasks are completed.
Let's look at a specific example showing how to use sync.WaitGroup
to handle concurrent tasks.
First, we need to import the sync
package:
import ( "fmt" "sync" )
Next, let us create a sync.WaitGroup
object:
var wg sync.WaitGroup
Then, we can add the number of tasks to wait by calling the Add
method. In this example, we will add two tasks:
wg.Add(2)
Next, we can start two coroutines to perform the tasks. We can encapsulate the task in an anonymous function and pass it as a parameter to the go
keyword.
go func() { defer wg.Done() // 这里是第一个任务的代码逻辑 }() go func() { defer wg.Done() // 这里是第二个任务的代码逻辑 }()
In this example, we put defer wg.Done()
inside each coroutine after the go
keyword to ensure that after the task is completed Able to notify the WaitGroup
object.
Finally, we can execute the Wait
method to block the main coroutine until all tasks are completed. This method reduces the number of tasks in the WaitGroup object to zero.
wg.Wait()
package main import ( "fmt" "sync" ) func main() { var wg sync.WaitGroup wg.Add(2) go func() { defer wg.Done() fmt.Println("Task 1 executed") }() go func() { defer wg.Done() fmt.Println("Task 2 executed") }() wg.Wait() fmt.Println("All tasks completed") }
sync.WaitGroup, we can easily handle concurrent tasks and ensure that all tasks are completed before continuing the execution of the main coroutine. This is especially useful when you need to wait for all tasks to be completed in the main coroutine, such as crawlers, parallel computing and other scenarios.
sync.WaitGroup can help us handle concurrent tasks in the Go language. It provides a concise and effective way to coordinate the execution of the coroutine and ensure that all tasks are completed before continuing the execution of the main coroutine. I hope this article helps you understand concurrent task processing.
The above is the detailed content of How to use Go WaitGroup to handle concurrent tasks. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
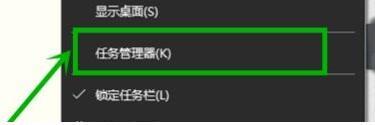
1. First, we right-click the blank space of the taskbar and select the [Task Manager] option, or right-click the start logo, and then select the [Task Manager] option. 2. In the opened Task Manager interface, we click the [Services] tab on the far right. 3. In the opened [Service] tab, click the [Open Service] option below. 4. In the [Services] window that opens, right-click the [InternetConnectionSharing(ICS)] service, and then select the [Properties] option. 5. In the properties window that opens, change [Open with] to [Disabled], click [Apply] and then click [OK]. 6. Click the start logo, then click the shutdown button, select [Restart], and complete the computer restart.
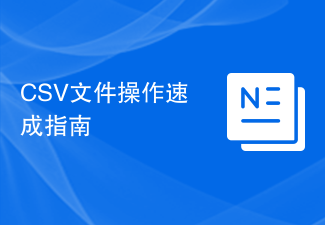
Quickly learn how to open and process CSV format files. With the continuous development of data analysis and processing, CSV format has become one of the widely used file formats. A CSV file is a simple and easy-to-read text file with different data fields separated by commas. Whether in academic research, business analysis or data processing, we often encounter situations where we need to open and process CSV files. The following guide will show you how to quickly learn to open and process CSV format files. Step 1: Understand the CSV file format First,
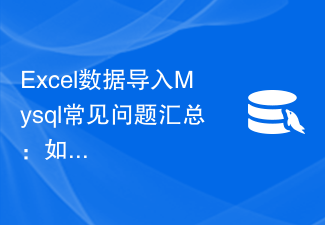
Summary of frequently asked questions about importing Excel data into Mysql: How to deal with error log problems encountered when importing data? Importing Excel data into a MySQL database is a common task. However, during this process, we often encounter various errors and problems. One of them is the error log issue. When we try to import data, the system may generate an error log listing the specific information about the error that occurred. So, how should we deal with the error log when we encounter this situation? First, we need to know how
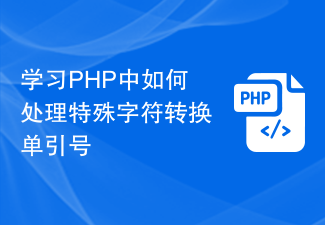
In the process of PHP development, dealing with special characters is a common problem, especially in string processing, special characters are often escaped. Among them, converting special characters into single quotes is a relatively common requirement, because in PHP, single quotes are a common way to wrap strings. In this article, we will explain how to handle special character conversion single quotes in PHP and provide specific code examples. In PHP, special characters include but are not limited to single quotes ('), double quotes ("), backslash (), etc. In strings
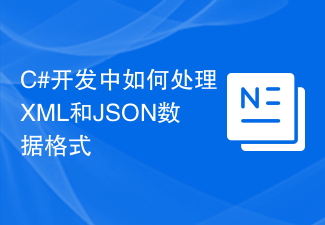
How to handle XML and JSON data formats in C# development requires specific code examples. In modern software development, XML and JSON are two widely used data formats. XML (Extensible Markup Language) is a markup language used to store and transmit data, while JSON (JavaScript Object Notation) is a lightweight data exchange format. In C# development, we often need to process and operate XML and JSON data. This article will focus on how to use C# to process these two data formats, and attach
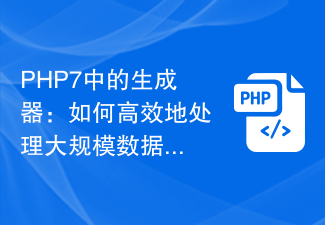
Generators in PHP7: How to handle large-scale data efficiently and save memory? Overview: PHP7 introduces generators as a powerful tool in terms of large-scale data processing and memory saving. Generators are a special type of function in the PHP language. Unlike ordinary functions, generators can pause execution and return intermediate results instead of returning all results at once. This makes the generator ideal for processing large batches of data, reducing memory usage and improving processing efficiency. This article will introduce students
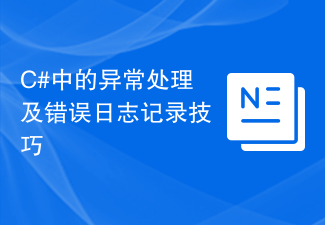
Exception handling and error logging skills in C# Introduction: In the software development process, exception handling and error logging are very important links. For C# developers, mastering exception handling skills and error logging methods can help us better track and debug code, and improve the stability and maintainability of the program. This article will introduce commonly used exception handling techniques in C# and provide specific code examples to help readers better understand and apply exception handling and error logging. 1. Basic concepts of exception handling Exceptions refer to the
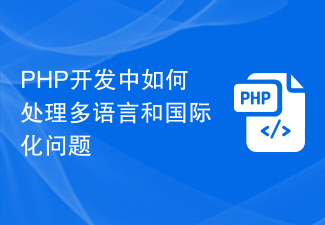
How to deal with multi-language and internationalization issues in PHP development requires specific code examples. With the development of the Internet, people's demand for multi-language and internationalization is getting higher and higher. In PHP development, how to effectively handle multi-language and internationalization issues has become an important task that developers need to solve. Handling of character encoding In PHP development, we must first ensure that character encoding is handled correctly. In multi-language environments, using UTF-8 encoding is the most common choice. You can add the following code to the head of the PHP file: header('C
