


How to implement asynchronous task queue using Celery, Redis and Django
How to implement asynchronous task queue using Celery, Redis and Django
Introduction:
In web development, we often need to handle some long-term tasks. Such as sending emails, generating reports, processing large amounts of data, etc. If these tasks are handled directly in the view function, the request response time will be too long and the user experience will be poor. In order to improve the performance and response speed of the system, we can use asynchronous task queues to handle these time-consuming tasks. Celery is a widely used asynchronous task queue framework for Python, and Redis is its default message middleware. This article will introduce how to use Celery, Redis and Django to implement asynchronous task queues, and provide specific code examples.
Step 1: Install Celery, Redis and Django
We must first install Celery, Redis and Django, and make relevant configurations in the Django configuration file.
- Install Celery: Run the following command in the command line to install Celery:
$ pip install Celery
- Install Redis: Celery uses Redis as the message middleware by default, we need Install Redis and make sure the Redis server is running.
- Configure Django: Add the following configuration to the Django configuration file (settings.py):
# 使用Redis作为消息中间件 CELERY_BROKER_URL = 'redis://localhost:6379/0' CELERY_RESULT_BACKEND = 'redis://localhost:6379/0'
Step 2: Create a Celery task
Next, we need to create a Celery tasks and define corresponding task functions. For example, let's create a task for sending emails.
- Create a file named tasks.py in Django’s project directory and add the following code:
from celery import shared_task from django.core.mail import send_mail @shared_task def send_email_task(subject, message, from_email, recipient_list): send_mail(subject, message, from_email, recipient_list)
- Call in Django’s view function Celery tasks:
from .tasks import send_email_task def send_email_view(request): # 获取邮件的相关参数 subject = 'Test Email' message = 'This is a test email.' from_email = 'sender@example.com' recipient_list = ['recipient@example.com'] # 调用Celery任务 send_email_task.delay(subject, message, from_email, recipient_list) return HttpResponse('Email sent!')
Step 3: Start Celery worker
Celery runs in a distributed architecture, with multiple workers responsible for processing tasks. We need to start Celery workers in the command line to process tasks in the task queue.
Run the following command to start the worker:
$ celery -A your_project_name worker -l info
Note, replace "your_project_name" with the name of your Django project.
Step 4: Run the Django server
Before starting the Celery worker, we need to run the Django server. Execute the following command in the root directory of the project:
$ python manage.py runserver
Now you can access the corresponding view function in the browser and observe Celery's log to view the execution of the task.
Summary:
By using Celery, Redis and Django, we can easily implement asynchronous task queues. By putting time-consuming tasks into the task queue, we can greatly improve the performance and response speed of the system and improve the user experience. At the same time, Celery’s distributed architecture allows us to flexibly expand the system’s processing capabilities. I hope this article will help you understand how to use Celery, Redis and Django to implement asynchronous task queues.
Reference link:
- Celery official documentation: https://docs.celeryproject.org/en/stable/index.html
- Django official documentation: https ://docs.djangoproject.com/
The above is the detailed content of How to implement asynchronous task queue using Celery, Redis and Django. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


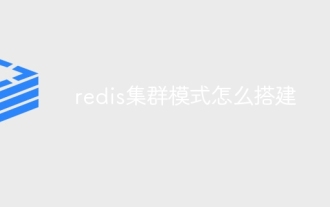
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make
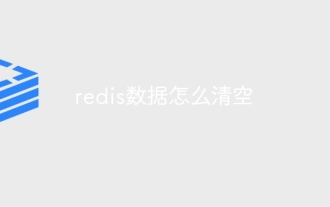
How to clear Redis data: Use the FLUSHALL command to clear all key values. Use the FLUSHDB command to clear the key value of the currently selected database. Use SELECT to switch databases, and then use FLUSHDB to clear multiple databases. Use the DEL command to delete a specific key. Use the redis-cli tool to clear the data.
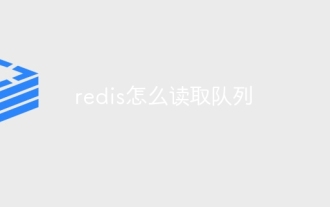
To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
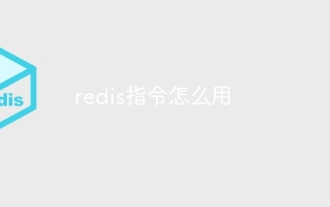
Using the Redis directive requires the following steps: Open the Redis client. Enter the command (verb key value). Provides the required parameters (varies from instruction to instruction). Press Enter to execute the command. Redis returns a response indicating the result of the operation (usually OK or -ERR).
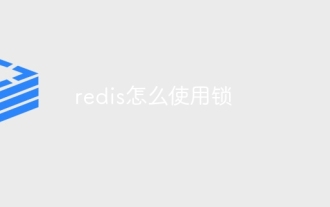
Using Redis to lock operations requires obtaining the lock through the SETNX command, and then using the EXPIRE command to set the expiration time. The specific steps are: (1) Use the SETNX command to try to set a key-value pair; (2) Use the EXPIRE command to set the expiration time for the lock; (3) Use the DEL command to delete the lock when the lock is no longer needed.
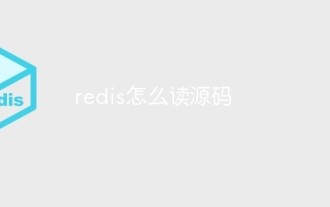
The best way to understand Redis source code is to go step by step: get familiar with the basics of Redis. Select a specific module or function as the starting point. Start with the entry point of the module or function and view the code line by line. View the code through the function call chain. Be familiar with the underlying data structures used by Redis. Identify the algorithm used by Redis.

Redis data loss causes include memory failures, power outages, human errors, and hardware failures. The solutions are: 1. Store data to disk with RDB or AOF persistence; 2. Copy to multiple servers for high availability; 3. HA with Redis Sentinel or Redis Cluster; 4. Create snapshots to back up data; 5. Implement best practices such as persistence, replication, snapshots, monitoring, and security measures.
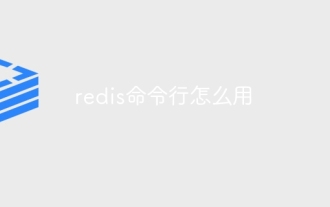
Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
