


Powerful ORM of Django framework and lightweight database access of Flask
The powerful ORM of the Django framework and the lightweight database access of Flask
Introduction:
In web development, the database is an indispensable part. Database access and operations are critical to the performance and reliability of a web application. Django and Flask are two popular Python web frameworks that provide different ways of accessing databases. This article will introduce the powerful ORM (Object Relational Mapping) in the Django framework and the lightweight database access method in the Flask framework, and provide specific code examples.
- Powerful ORM of Django framework
Django is a full-featured web application framework, of which ORM is an important part of it. ORM is a programming technology that allows developers to operate the database in an object-oriented manner by mapping tables in the database to Python objects. Django's ORM provides rich functions and convenient syntax, which can perform complex query, insert, update and delete operations on the database.
The following is a simple Django ORM example, showing how to create a model class, insert data and query data through ORM:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
In the above code, we first define a User model class, which maps to the "user" table in the database. Then, we created two User objects and saved them to the database. Finally, through the User.objects.all() method, we obtain all User objects in the database and print their names and ages.
Django's ORM also provides a wealth of query methods, such as filter(), exclude() and annotate(), etc., which can filter data according to conditions, exclude specified data, and perform aggregation operations. In addition, ORM also supports advanced functions such as transaction management, data migration and model association, which is very suitable for complex database operation requirements.
- Flask's lightweight database access
Compared with Django, Flask is a more lightweight web framework that does not have a built-in ORM. However, Flask provides the extension package "Flask-SQLAlchemy", which is a Flask integrated version of SQLAlchemy and provides ORM support for Flask applications.
SQLAlchemy is a Python SQL toolkit that allows developers to use SQL language for database operations in Python by providing an efficient and flexible database access interface. Flask-SQLAlchemy integrates SQLAlchemy and provides convenient database access.
The following is a simple Flask-SQLAlchemy example that shows how to use ORM for database operations:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
|
In the above code, first we create a Flask application and configure the database connection . Then a User model class is defined, and the table and field types are specified by extending the syntax of Flask-SQLAlchemy. Next, we created two User objects and added them to the database through the db.session.add()
and db.session.commit()
methods, and finally User.query.all()
Obtains all User objects in the database and prints their names and ages.
In addition to basic database operations, Flask-SQLAlchemy also supports complex queries, transaction management, data migration, model association and other functions, which can meet most common database needs.
Summary:
The Django framework provides rich database access functions through its powerful ORM, which is suitable for complex database operations and large-scale projects. The Flask framework provides a lightweight database access method through Flask-SQLAlchemy, which is suitable for small projects or scenarios with low database access requirements. Based on actual needs, we can choose the appropriate framework and database access method, and use the functions and syntax it provides to develop efficient and reliable Web applications.
Reference:
- Django documentation. [Online]. Available: https://docs.djangoproject.com/
- Flask documentation. [Online]. Available: https://flask.palletsprojects.com/
- SQLAlchemy documentation. [Online]. Available: https://www.sqlalchemy.org/
- Flask-SQLAlchemy documentation. [Online] ]. Available: https://flask-sqlalchemy.palletsprojects.com/
The above is the detailed content of Powerful ORM of Django framework and lightweight database access of Flask. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


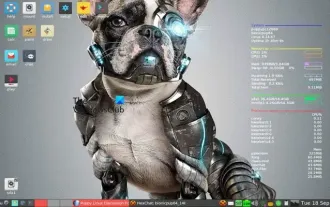
Looking for the perfect Linux distribution to breathe new life into your old or low-end computer? If yes, then you have come to the right place. In this article, we'll explore some of our top picks for lightweight Linux distributions that are specifically tailored for older or less powerful hardware. Whether the motivation behind this is to revive an aging device or simply maximize performance on a budget, these lightweight options are sure to fit the bill. Why choose a lightweight Linux distribution? There are several advantages to choosing a lightweight Linux distribution, the first of which is getting the best performance on the least system resources, which makes them ideal for older hardware with limited processing power, RAM, and storage space. Beyond that, with heavier resource intensive
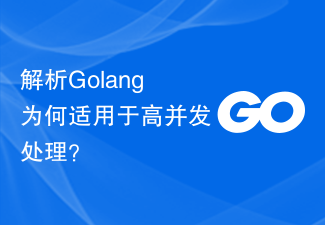
Golang (Go language) is a programming language developed by Google, aiming to provide an efficient, concise, concurrent and lightweight programming experience. It has built-in concurrency features and provides developers with powerful tools to perform well in high-concurrency situations. This article will delve into the reasons why Golang is suitable for high-concurrency processing and provide specific code examples to illustrate. Golang concurrency model Golang adopts a concurrency model based on goroutine and channel. goro
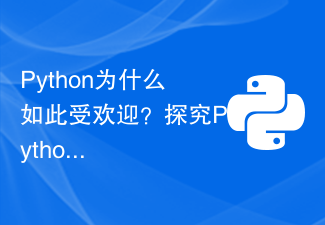
Why is Python so popular? To explore the advantages of Python in the field of programming, specific code examples are required. As a high-level programming language, Python has been loved and respected by programmers since its inception. The reason is not only because of its simplicity, readability and powerful functions, but also because it has shown unparalleled advantages in various fields. This article will explore the advantages of Python in the field of programming and explain why Python is so popular through specific code examples. First, Python
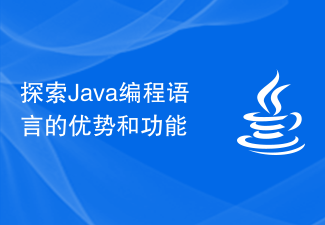
Java is a widely used computer programming language launched by SunMicrosystems in 1995. It is a high-level, object-oriented, portable programming language designed for developing cross-platform applications. Java programming language has many advantages that make it popular in the field of software development. First of all, Java is an object-oriented programming language. Object-oriented programming is a computer programming paradigm that encapsulates the data and operations in the program in objects.
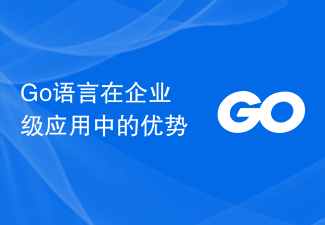
One of the reasons why thinking about using Go language for enterprise-level application development is gaining more and more attention is its excellent performance and concurrency features. This article will explore the advantages of Go language in enterprise-level application development and give specific code examples to illustrate these advantages. 1. Concurrent programming capabilities Go language achieves efficient concurrent programming through the goroutine mechanism, which gives it obvious advantages in handling concurrent tasks. Using goroutine, we can easily create concurrent tasks and execute them through channels
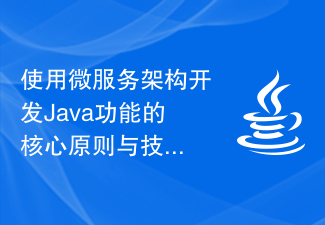
Core principles and techniques for developing Java functions using microservice architecture. With the rapid development of cloud computing and big data, traditional monolithic applications can no longer adapt to complex business needs. Microservices Architecture emerged as the times require and has become an emerging paradigm for building scalable, flexible and maintainable applications. In this article, we will explore the core principles and techniques of using microservices architecture to develop Java functions and give specific code examples. Single Responsibility Principle (Sin
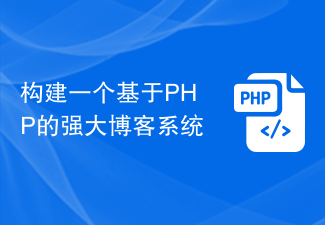
Build a powerful blog system based on PHP In the Internet era, personal blogs have become an important platform for many people to record and share their thoughts, experiences and knowledge. In order to meet the needs of different users, it is very necessary to build a powerful blog system. In this article, we will use PHP language to build a feature-rich blog system and provide code examples. Database design Before building a blog system, you first need to design the database structure. A typical blog system usually contains data tables such as users, articles, comments, etc. under
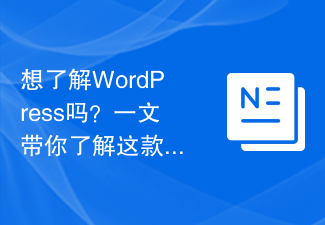
WordPress is one of the most popular website building and content management systems in the world today. Its flexibility and customizability make it the tool of choice for many website owners and developers. Whether it is a personal blog, corporate website, or e-commerce platform, WordPress can provide powerful functions and scalability. This article will discuss in depth the features, advantages and how to use WordPress to build your own website. First, let us understand the origin and development history of WordPress. Wo
