


Implement efficient memory management and garbage collector tuning in Go language
Achieve efficient memory management and garbage collector tuning in Go language
Introduction:
Go language is known for its efficiency, simplicity and concurrency performance , one of the reasons is its efficient memory management and garbage collection mechanism. In this article, I will take you through an in-depth discussion of how to implement efficient memory management and optimize the garbage collector in the Go language, while providing detailed code examples.
- Avoid frequent memory allocation and release
In the Go language, frequent memory allocation and release will cause performance degradation, so we need to try to avoid this situation. A more common approach is to use object pooling, which means creating some objects in advance instead of creating new objects every time they are needed. The object pool can be implemented through sync.Pool. Here is a simple example:
package main import ( "fmt" "sync" ) type Object struct { // ... } var objectPool = sync.Pool{ New: func() interface{} { return &Object{} }, } func main() { obj := objectPool.Get().(*Object) // 使用obj进行自己的操作 objectPool.Put(obj) }
- Limit memory usage
In order to avoid excessive use of memory, we can allocate smaller sizes to goroutine The stack space allows more goroutines to run at the same time. In the Go language, the default stack size is 2KB, but it can be changed through runtime.GOMAXPROCS. The following is an example:
package main import ( "fmt" "runtime" "sync" ) func main() { runtime.GOMAXPROCS(1) // 设置只有一个逻辑处理器 var wg sync.WaitGroup for i := 0; i < 100000; i++ { wg.Add(1) go func() { defer wg.Done() // do something }() } wg.Wait() fmt.Println("All goroutines finished") }
- Garbage collector tuning
The garbage collector of the Go language uses a three-color mark removal algorithm, and can ensure the safety of the program in the case of concurrency. normal operation. By default, the Go language's garbage collector dynamically adjusts its scheduling strategy based on the program's memory usage. However, we can optimize the performance of the garbage collector through some means.
One way to optimize the performance of the garbage collector is by adjusting the value of the environment variable GOGC. The default value of GOGC is 100, which means that when the ratio of the memory occupied by the heap to the recycled memory is greater than 100, a garbage collection operation is triggered. We can increase or decrease the triggering frequency of the garbage collector by adjusting the value of GOGC.
Another way to optimize garbage collector performance is to manually trigger garbage collection operations. In the Go language, we can use runtime.GC() to manually trigger a garbage collection operation. The following is an example:
package main import ( "fmt" "runtime" "time" ) func main() { runtime.GOMAXPROCS(1) // 设置只有一个逻辑处理器 var m runtime.MemStats for i := 0; i < 1000000; i++ { time.Sleep(time.Millisecond * 10) // 模拟程序的运行 // do something runtime.ReadMemStats(&m) if m.HeapReleased > 1000000000 { // 当已释放的堆内存超过1GB时,手动触发垃圾回收 runtime.GC() } } fmt.Println("Program finished") }
Conclusion:
Through good memory management and garbage collector tuning, we can further improve the performance and stability of Go language applications. I hope that the code examples in this article can help you and inspire you to explore more optimization strategies in practice. Let us use the powerful memory management and garbage collection features of the Go language to create more efficient programs!
The above is the detailed content of Implement efficient memory management and garbage collector tuning in Go language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



C++ object layout and memory alignment optimize memory usage efficiency: Object layout: data members are stored in the order of declaration, optimizing space utilization. Memory alignment: Data is aligned in memory to improve access speed. The alignas keyword specifies custom alignment, such as a 64-byte aligned CacheLine structure, to improve cache line access efficiency.
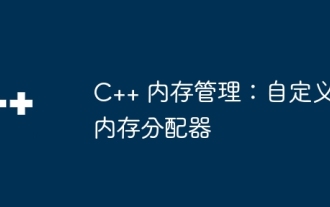
Custom memory allocators in C++ allow developers to adjust memory allocation behavior according to needs. Creating a custom allocator requires inheriting std::allocator and rewriting the allocate() and deallocate() functions. Practical examples include: improving performance, optimizing memory usage, and implementing specific behaviors. When using it, you need to pay attention to avoid freeing memory, manage memory alignment, and perform benchmark tests.
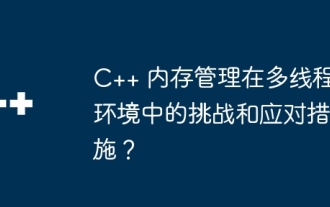
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.
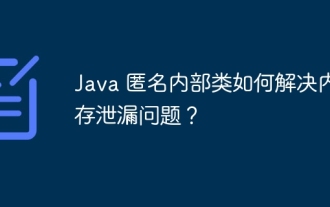
Anonymous inner classes can cause memory leaks. The problem is that they hold a reference to the outer class, preventing the outer class from being garbage collected. Solutions include: 1. Use weak references. When the external class is no longer held by a strong reference, the garbage collector will immediately recycle the weak reference object; 2. Use soft references. The garbage collector will recycle the weak reference object when it needs memory during garbage collection. Only then the soft reference object is recycled. In actual combat, such as in Android applications, the memory leak problem caused by anonymous inner classes can be solved by using weak references, so that the anonymous inner class can be recycled when the listener is not needed.
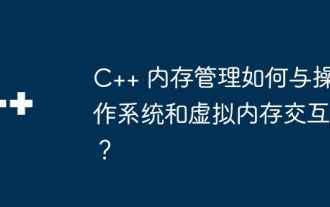
C++ memory management interacts with the operating system, manages physical memory and virtual memory through the operating system, and efficiently allocates and releases memory for programs. The operating system divides physical memory into pages and pulls in the pages requested by the application from virtual memory as needed. C++ uses the new and delete operators to allocate and release memory, requesting memory pages from the operating system and returning them respectively. When the operating system frees physical memory, it swaps less used memory pages into virtual memory.

The reference counting mechanism is used in C++ memory management to track object references and automatically release unused memory. This technology maintains a reference counter for each object, and the counter increases and decreases when references are added or removed. When the counter drops to 0, the object is released without manual management. However, circular references can cause memory leaks, and maintaining reference counters increases overhead.
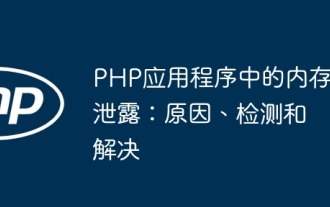
A PHP memory leak occurs when an application allocates memory and fails to release it, resulting in a reduction in the server's available memory and performance degradation. Causes include circular references, global variables, static variables, and expansion. Detection methods include Xdebug, Valgrind and PHPUnitMockObjects. The resolution steps are: identify the source of the leak, fix the leak, test and monitor. Practical examples illustrate memory leaks caused by circular references, and specific methods to solve the problem by breaking circular references through destructors.
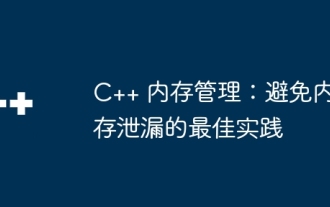
Memory leaks are a common mistake in C++ that can be avoided through best practices: use smart pointers to automatically manage memory and avoid dangling pointers. Follow the RAII principle to ensure resources are released when they are no longer needed. Write a custom destructor to explicitly release resources. Periodically call delete to release dynamically allocated memory. Use memory leak detection tools to identify potential problems.
