Unlock the secrets of Golang Facade mode to make coding easier
Unlock the secrets of Golang Facade mode and make coding easier
Introduction:
In software development, we often encounter multiple complex problems in a system subsystems, and we need to provide a simplified interface to the outside world to access these subsystems. At this time, the Facade mode can come in handy. The Facade pattern encapsulates complex subsystems by providing a unified interface, allowing clients to use these subsystems more easily.
This article will introduce how to apply the Facade pattern in Golang, explain its principles and applications through specific code examples, and help readers better understand and use this pattern.
Background:
Suppose we want to write a facade pattern for an order management system. The order management system has the following complex subsystems: inventory management, payment management and logistics management. In order to allow clients to conveniently operate these subsystems, we will use the Facade pattern for encapsulation.
Code implementation:
First, we need to define the interfaces of the three subsystems and implement specific functions in each subsystem. Taking inventory management as an example, the code is as follows:
type InventoryManager interface { CheckStock(productId int) bool ReduceStock(productId int, quantity int) bool } type InventoryManagerImpl struct { // 具体的库存管理实现 } func (i *InventoryManagerImpl) CheckStock(productId int) bool { // 检查库存是否足够的具体实现 } func (i *InventoryManagerImpl) ReduceStock(productId int, quantity int) bool { // 减少库存的具体实现 }
Next, we define a facade interface to encapsulate the specific calling methods of these three subsystems. The code is as follows:
type OrderFacade interface { CheckStock(productId int) bool PlaceOrder(productId int, quantity int) bool CancelOrder(orderId int) bool } type OrderFacadeImpl struct { inventoryManager InventoryManager paymentManager PaymentManager logisticsManager LogisticsManager } func (o *OrderFacadeImpl) CheckStock(productId int) bool { // 调用库存管理子系统的具体方法 return o.inventoryManager.CheckStock(productId) } func (o *OrderFacadeImpl) PlaceOrder(productId int, quantity int) bool { // 调用库存管理、支付管理和物流管理子系统的具体方法 if o.inventoryManager.CheckStock(productId) { if o.paymentManager.Pay(productId, quantity) { if o.logisticsManager.Ship(productId, quantity) { return true } } } return false } func (o *OrderFacadeImpl) CancelOrder(orderId int) bool { // 调用支付管理和物流管理子系统的具体方法 if o.paymentManager.Refund(orderId) { if o.logisticsManager.CancelShip(orderId) { return true } } return false }
Next, we need to implement specific subsystems, namely payment management and logistics management. The code is as follows:
type PaymentManager interface { Pay(productId int, quantity int) bool Refund(orderId int) bool } type PaymentManagerImpl struct { // 具体的支付管理实现 } func (p *PaymentManagerImpl) Pay(productId int, quantity int) bool { // 支付的具体实现 } func (p *PaymentManagerImpl) Refund(orderId int) bool { // 退款的具体实现 } type LogisticsManager interface { Ship(productId int, quantity int) bool CancelShip(orderId int) bool } type LogisticsManagerImpl struct { // 具体的物流管理实现 } func (l *LogisticsManagerImpl) Ship(productId int, quantity int) bool { // 发货的具体实现 } func (l *LogisticsManagerImpl) CancelShip(orderId int) bool { // 取消发货的具体实现 }
Finally, we can use these subsystems through the facade, simplifying the client code. The code is as follows:
func main() { orderFacade := &OrderFacadeImpl{ inventoryManager: &InventoryManagerImpl{}, paymentManager: &PaymentManagerImpl{}, logisticsManager: &LogisticsManagerImpl{}, } // 检查库存是否足够 if orderFacade.CheckStock(1001) { // 下订单 if orderFacade.PlaceOrder(1001, 1) { // 取消订单 if orderFacade.CancelOrder(10001) { fmt.Println("订单已取消") } else { fmt.Println("取消订单失败") } } else { fmt.Println("下订单失败") } } else { fmt.Println("库存不足") } }
Summary:
Through the above examples, we can see that through the Facade mode, we encapsulate complex subsystems and provide a unified interface for the client to use. The client can more easily call the functions of these subsystems. At the same time, through encapsulation and abstraction, we enable subsystems to evolve independently without affecting the client's calling code.
I hope that through the introduction of this article, readers can understand and master the usage and principles of Facade mode in Golang, so that they can use this mode more flexibly in actual development.
The above is the detailed content of Unlock the secrets of Golang Facade mode to make coding easier. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


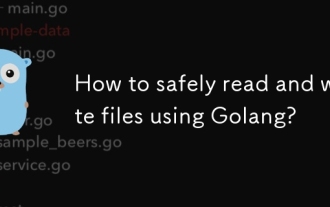
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
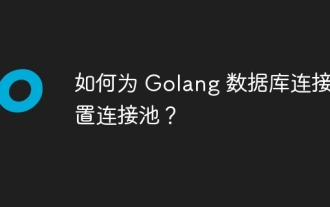
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
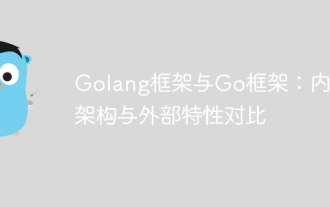
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
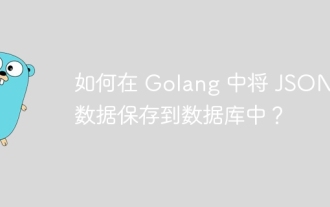
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
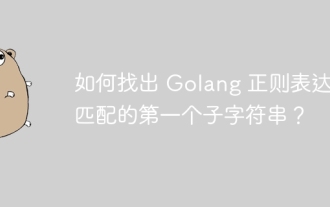
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
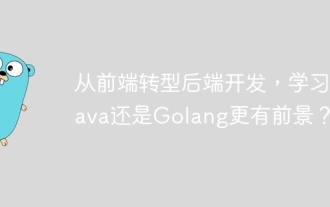
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
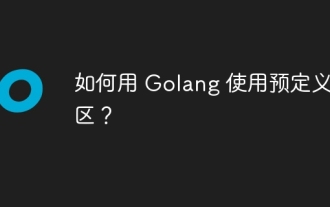
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
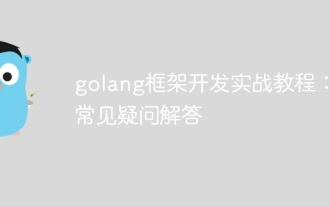
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
