


Using Java to develop order tracking and logistics network optimization functions of warehouse management systems
Java is a very powerful and widely used programming language in the field of software development. This article will introduce how to use Java to develop a warehouse management system and add order tracking and logistics network optimization functions. At the same time, some specific code examples will also be provided to help readers understand better.
A warehouse management system is a software system used to track and manage items and orders in a warehouse. It can help warehouse managers effectively manage and handle various operations, including order management, inventory management, logistics management, etc. Order tracking and logistics network optimization are very important functions in warehouse management systems. They can help companies improve order processing speed and accuracy, reduce logistics costs, and improve customer satisfaction.
The following is a simple Java code example of a warehouse management system:
public class Warehouse { private List<Order> orders; public Warehouse() { this.orders = new ArrayList<>(); } public void addOrder(Order order) { this.orders.add(order); } public void removeOrder(Order order) { this.orders.remove(order); } public List<Order> getOrders() { return this.orders; } } public class Order { private String orderId; private String customerName; private String address; public Order(String orderId, String customerName, String address) { this.orderId = orderId; this.customerName = customerName; this.address = address; } // Getters and setters // Other methods } public class Main { public static void main(String[] args) { Warehouse warehouse = new Warehouse(); Order order1 = new Order("1001", "张三", "北京市"); Order order2 = new Order("1002", "李四", "上海市"); warehouse.addOrder(order1); warehouse.addOrder(order2); List<Order> orders = warehouse.getOrders(); for(Order order : orders) { System.out.println("订单号:" + order.getOrderId()); System.out.println("顾客姓名:" + order.getCustomerName()); System.out.println("地址:" + order.getAddress()); System.out.println("---------------------"); } } }
In the above code, we define three classes: Warehouse
, Order
and Main
. The Warehouse
class is used to represent a warehouse, which contains a list of stored orders. The Order
class represents an order, which contains information such as the order number, customer name and address. The Main
class is the entry point of the program and is used to demonstrate how to use the Warehouse
class.
In the Main
class, we create a warehouse object, then create two order objects and add them to the warehouse. Finally, we obtain the order list in the warehouse through the getOrders()
method, and traverse to print out the details of each order.
Order tracking and logistics network optimization are very important functions in warehouse management systems. Order tracking can provide real-time status and location information of orders to customers by tracking logistics information, allowing customers to understand the progress of orders in real time. Logistics network optimization reduces logistics costs and transportation time by optimizing the transportation path of products. Both functions are very important for enterprises to improve operational efficiency and improve customer satisfaction.
In actual development, we can implement the order tracking function by calling the third-party logistics company's API. For example, you can use Alibaba's logistics platform interface to query the logistics track information of an order by passing the order number and courier company code. Logistics network optimization can be achieved by using some algorithms and technologies, such as using Dijkstra's algorithm to find the shortest path, selecting the optimal transportation path, etc.
The above is a simple Java code example of a warehouse management system, and introduces the functions of order tracking and logistics network optimization. I hope it will be helpful to readers, and also encourage readers to further explore and develop in practice.
The above is the detailed content of Using Java to develop order tracking and logistics network optimization functions of warehouse management systems. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
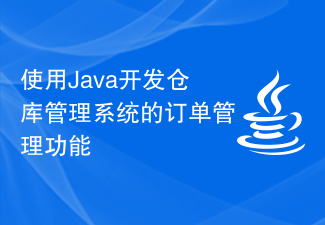
Order management function of Java warehouse management system Order management is one of the important functions of the warehouse management system. Through order management, you can purchase, view, modify and delete products in the warehouse. In this article, we will introduce how to use Java to develop the order management function of the warehouse management system and provide specific code examples. System Requirements Analysis Before developing the order management function, system requirements analysis needs to be performed first. According to actual needs, the order management function should include the following basic functions: Add order: add products to the order
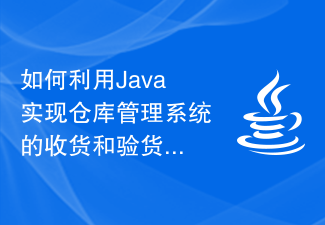
How to use Java to implement the receiving and inspection functions of the warehouse management system. With the development of e-commerce, the importance of the warehouse management system cannot be ignored. In the warehouse management system, receiving and inspecting goods are crucial links. This article will introduce how to use Java to implement the receiving and inspection functions in the warehouse management system, and provide specific code examples. 1. Implementation of the goods receipt function The goods receipt function refers to the process of receiving goods from suppliers and warehousing them. In the warehouse management system, we can implement the receiving function through the following steps. 1.1Create goods category header
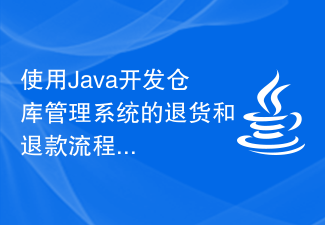
Use Java to develop the return and refund process of the warehouse management system. Title: The return and refund process of the Java warehouse management system and code examples 1. Introduction With the rapid development of e-commerce, warehouse management systems have become indispensable in the modern logistics industry. a part of. Among them, the return and refund process is an important function in the warehouse management system. This article will introduce how to use Java language to develop a complete return and refund process in a warehouse management system, and provide relevant code examples. 2. Return process design User initiates return
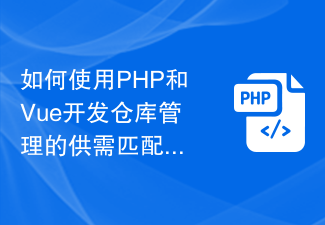
How to use PHP and Vue to develop the supply and demand matching function of warehouse management In logistics and supply chain management, warehouse management is an important task. An effective warehouse management system can improve logistics efficiency, reduce costs, and ensure inventory accuracy. For better warehouse management, supply and demand matching functionality is an essential feature. This article will introduce how to use PHP and Vue to develop the supply and demand matching function of warehouse management, and provide specific code examples. 1. Demand analysis Before developing the supply and demand matching function of warehouse management, we first need to
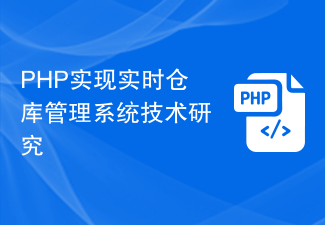
With the continuous development of the e-commerce industry, warehouse management has become one of the important aspects of enterprise supply chain management. Traditional warehouse management methods can no longer meet the needs of enterprises. How to achieve efficient and accurate warehouse management has become an urgent problem that enterprises need to solve. This article will discuss and analyze the technical research on implementing real-time warehouse management system in PHP. 1. Overview of Warehouse Management System Warehouse management system is a systematic management platform established by enterprises to manage and control inventory. It mainly includes warehouse management, goods warehousing, goods outgoing, inventory counting and supply.
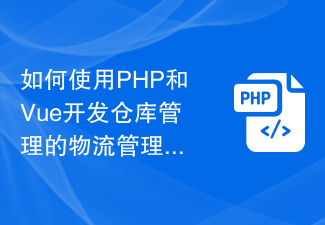
How to use PHP and Vue to develop the logistics management function of warehouse management. With the rapid development of e-commerce, the logistics management function of warehouse management has become more and more important. In this article, I will introduce how to use PHP and Vue to develop a simple and practical warehouse management system, and provide specific code examples. Environment preparation Before starting development, we need to prepare some development environment. First, make sure you have the PHP and Vue development environments installed on your computer. You can download and install XAMPP, WAMP or
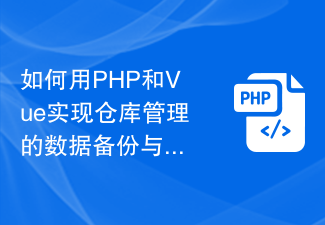
How to use PHP and Vue to implement the data backup and recovery functions of warehouse management requires specific code examples. In modern warehouse management systems, data backup and recovery are one of the indispensable functions. Warehouse management involves a large amount of data, including inventory information, warehousing records, outbound records, etc. Therefore, ensuring data security and reliability is crucial. In this article, we will introduce how to use PHP and Vue to implement the data backup and recovery functions of warehouse management, and give specific code examples. 1. Data backup function creates databases and tables
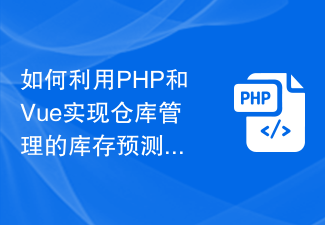
How to use PHP and Vue to implement the inventory forecasting function of warehouse management requires specific code examples [Introduction] In the warehouse management system, inventory forecasting is a crucial part, which can help warehouse managers prepare stocking and sales plans in advance. , thereby improving the operational efficiency and profits of the warehouse. This article will introduce how to use PHP and Vue, two popular development tools, to implement the inventory prediction function in warehouse management, and give specific code examples. [Background] Warehouse management systems usually involve the purchase, sales, inventory and other processes of goods.
