


React Component Library Development Guide: How to Build Reusable UI Components
React Component Library Development Guide: How to Build Reusable UI Components
With the rapid development and widespread application of React, more and more developers are starting to Realize the importance of building reusable UI components. A good UI component library can effectively improve development efficiency, maintain project consistency, and can be easily referenced and used by other developers. This article will provide some guidelines and specific code examples to help developers build their own React component library.
- Design a good component structure
Before you start writing specific UI components, be sure to consider the overall structure of the component. A good component structure should be scalable, testable and maintainable. Here are some design principles and best practices:
- Split components into smaller parts: A component should only focus on one thing, and should be as simple and independent as possible. If a component is too complex, try splitting it into smaller subcomponents.
- Use configurable properties: Configure the appearance and behavior of the component by passing properties to make it more flexible and extensible. For example, you can control color, size, style, etc. through properties.
- Follow the single responsibility principle: each component should only focus on one thing and try to avoid introducing too much business logic. This makes components more reusable and easier to maintain and test in different scenarios.
- Using PropTypes for type checking
In React, PropTypes is a powerful tool for validating the types of component properties. By using PropTypes, you can better define a component's interface and be able to warn when a component uses incorrect property types. The following are some common PropTypes types:
- string: String type
- number: Number type
- bool: Boolean type
- object: Object type
- array: Array type
- func: Function type
It is very simple to define PropTypes in the properties of the component. For example, assuming we have a Button component, we can use the following code to define its property type:
import PropTypes from 'prop-types'; const Button = (props) => { // ... }; Button.propTypes = { text: PropTypes.string, onClick: PropTypes.func, disabled: PropTypes.bool, };
- Provide default properties
It is very convenient to define default properties for the component Good practice. This can reduce the user's burden when referencing components and make the use of components more concise and intuitive. Here is an example of setting a default property:
const Button = (props) => { // ... }; Button.defaultProps = { text: 'Click me', onClick: () => {}, disabled: false, };
- Use the correct context
When developing React components, sometimes you need to share data between components. A common approach is to use context to pass data. However, after React v16.3, it is officially recommended to use Context API instead of context. The following is an example of using the Context API to share data:
First, create a Context object in the parent component:
import React from 'react'; const MyContext = React.createContext();
Then, use the Provider component in the parent component to pass the data:
<MyContext.Provider value={myData}> // 子组件 </MyContext.Provider>
Finally, use the Consumer component in a child component to receive data:
<MyContext.Consumer> {data => ( // 使用data来访问共享的数据 )} </MyContext.Consumer>
- Write clear documentation and examples
Good documentation and examples are An essential part of a successful React component library. Users need to clearly understand the purpose, properties, and usage of each component. Here are some suggestions:
- Provide a description and purpose of the component. This can help users quickly understand the function and applicable scenarios of the component.
- Provide code examples and demonstrations. By providing runnable code examples and demonstrations, users can better understand how to use components and achieve desired results.
- Provide property documentation. The documentation should clearly list all properties of the component and describe the purpose and type of each property.
- Provides frequently asked questions and answers. Users often encounter some common problems, and providing frequently asked questions and answers can help users solve problems faster.
Conclusion
Building a high-quality, reusable React component library is not an easy task, but by following the above guidelines and best practices, and constantly By accumulating experience, you can build a powerful and scalable component library, improve the development efficiency of your team, and provide valuable tools and resources to more developers. I hope this article can help you in the process of building a React component library.
The above is the detailed content of React Component Library Development Guide: How to Build Reusable UI Components. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

How to build a real-time chat application using React and WebSocket Introduction: With the rapid development of the Internet, real-time communication has attracted more and more attention. Live chat apps have become an integral part of modern social and work life. This article will introduce how to build a simple real-time chat application using React and WebSocket, and provide specific code examples. 1. Technical preparation Before starting to build a real-time chat application, we need to prepare the following technologies and tools: React: one for building
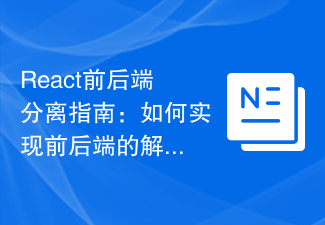
React front-end and back-end separation guide: How to achieve front-end and back-end decoupling and independent deployment, specific code examples are required In today's web development environment, front-end and back-end separation has become a trend. By separating front-end and back-end code, development work can be made more flexible, efficient, and facilitate team collaboration. This article will introduce how to use React to achieve front-end and back-end separation, thereby achieving the goals of decoupling and independent deployment. First, we need to understand what front-end and back-end separation is. In the traditional web development model, the front-end and back-end are coupled
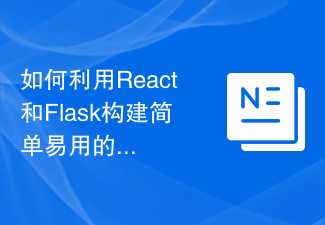
How to use React and Flask to build simple and easy-to-use web applications Introduction: With the development of the Internet, the needs of web applications are becoming more and more diverse and complex. In order to meet user requirements for ease of use and performance, it is becoming increasingly important to use modern technology stacks to build network applications. React and Flask are two very popular frameworks for front-end and back-end development, and they work well together to build simple and easy-to-use web applications. This article will detail how to leverage React and Flask
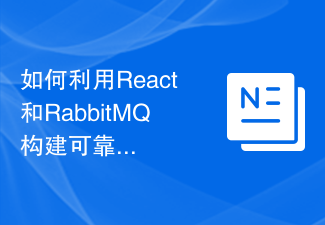
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
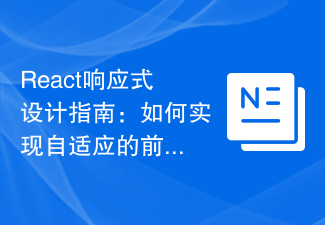
React Responsive Design Guide: How to Achieve Adaptive Front-end Layout Effects With the popularity of mobile devices and the increasing user demand for multi-screen experiences, responsive design has become one of the important considerations in modern front-end development. React, as one of the most popular front-end frameworks at present, provides a wealth of tools and components to help developers achieve adaptive layout effects. This article will share some guidelines and tips on implementing responsive design using React, and provide specific code examples for reference. Fle using React
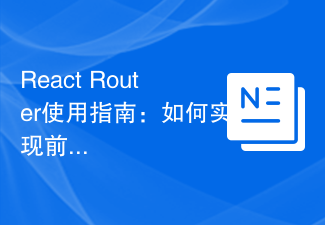
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
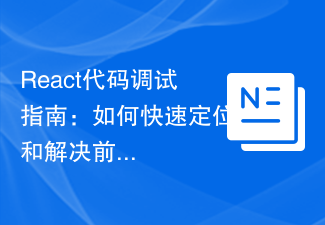
React code debugging guide: How to quickly locate and resolve front-end bugs Introduction: When developing React applications, you often encounter a variety of bugs that may crash the application or cause incorrect behavior. Therefore, mastering debugging skills is an essential ability for every React developer. This article will introduce some practical techniques for locating and solving front-end bugs, and provide specific code examples to help readers quickly locate and solve bugs in React applications. 1. Selection of debugging tools: In Re
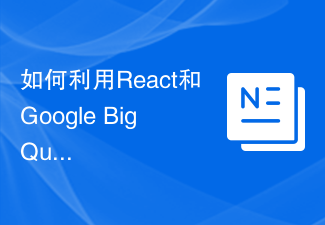
How to use React and Google BigQuery to build fast data analysis applications Introduction: In today's era of information explosion, data analysis has become an indispensable link in various industries. Among them, building fast and efficient data analysis applications has become the goal pursued by many companies and individuals. This article will introduce how to use React and Google BigQuery to build a fast data analysis application, and provide detailed code examples. 1. Overview React is a tool for building
