Testing and debugging practices under the Django framework
Testing and debugging practices under the Django framework
Introduction:
When developing web applications, testing and debugging are indispensable steps. As a popular development framework, Django provides many useful tools and functions that can help us conduct effective testing and debugging. This article will introduce some best practices for testing and debugging under the Django framework and provide some specific code examples.
1. Unit Testing
Unit testing is a testing method used to verify that the smallest unit of code in an application works as expected. In Django, you can use the Django testing framework to write unit tests.
The following is a simple example showing how to write a Django unit test.
from django.test import TestCase from django.urls import reverse from myapp.models import MyModel class MyModelTestCase(TestCase): def setUp(self): self.my_model = MyModel.objects.create(name='Test') def test_model_creation(self): self.assertEqual(self.my_model.name, 'Test')
In the above example, we first imported the necessary libraries and modules. Then, a test class named MyModelTestCase
is created, which inherits from the TestCase
class.
setUp
method is used to create and set up the test environment before each test method is executed. In this example, we create an object named my_model
and store it in an instance variable for use by the test method.
test_model_creation
method is an actual test method. We use the assertEqual
assertion to verify that the name
property of the my_model
object is equal to 'Test'
.
One way to run unit tests is to use a command line tool to run the ./manage.py test
command.
2. Integration testing
Integration testing is a testing method used to verify that the interactions between various components, modules, and dependencies work as expected. In Django, you can use Django's integration testing tool to write integration tests.
The following is a simple example showing how to write a Django integration test.
from django.test import TestCase from django.urls import reverse from django.contrib.auth.models import User class MyViewTestCase(TestCase): def setUp(self): self.user = User.objects.create(username='testuser') self.client.force_login(self.user) def test_my_view(self): response = self.client.get(reverse('my-view')) self.assertEqual(response.status_code, 200)
In the above example, we first imported the necessary libraries and modules. Then, a test class named MyViewTestCase
is created, which inherits from the TestCase
class.
setUp
method is used to create and set up the test environment before each test method is executed. In this example, we create a user named user
and force login to the user through the force_login
method.
test_my_view
method is an actual test method. We use the client
object to simulate a request, use the reverse
method to get the URL, and verify whether the response status code is 200.
The method of running integration tests is similar to running unit tests. Use the command line tool to run the ./manage.py test
command.
3. Debugging
During the development process, debugging is a commonly used technology to identify and solve bugs. Django provides some useful debugging tools and techniques to help us quickly locate problems.
- Use the
print
statement:
Using theprint
statement in the code is a simple and effective debugging method. By outputting the value of a variable, the execution path of the code can be traced.
def my_function(): ... print(my_variable) ...
- Use Django's debugging tools:
Django provides some useful debugging tools to make debugging more convenient.
- Django Debug Toolbar is a commonly used debugging tool that provides detailed request and response information, as well as SQL query and performance analysis and other functions.
- Django shell is an interactive Python shell that can interact with the Django environment and test and debug code in it.
Conclusion:
By using the testing and debugging functions provided by the Django framework, we can effectively verify and debug the code of the web application. Whether they are unit tests or integration tests, they can help us identify potential problems and ensure that the application works as expected. Debugging tools and techniques help quickly locate and resolve bugs. By taking full advantage of these testing and debugging methods, we can improve code quality and provide a better user experience.
Reference materials:
- Django official documentation: https://docs.djangoproject.com/
- Django Debug Toolbar official documentation: https://django- debug-toolbar.readthedocs.io/
The above is the detailed content of Testing and debugging practices under the Django framework. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


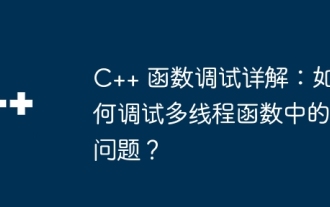
C++ multi-thread debugging can use GDB: 1. Enable debugging information compilation; 2. Set breakpoints; 3. Use infothreads to view threads; 4. Use thread to switch threads; 5. Use next, stepi, and locals to debug. Actual case debugging deadlock: 1. Use threadapplyallbt to print the stack; 2. Check the thread status; 3. Single-step the main thread; 4. Use condition variables to coordinate access to solve the deadlock.
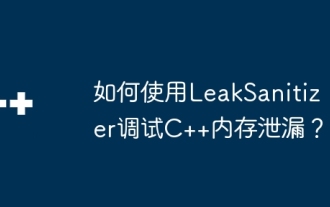
How to use LeakSanitizer to debug C++ memory leaks? Install LeakSanitizer. Enable LeakSanitizer via compile flag. Run the application and analyze the LeakSanitizer report. Identify memory allocation types and allocation locations. Fix memory leaks and ensure all dynamically allocated memory is released.
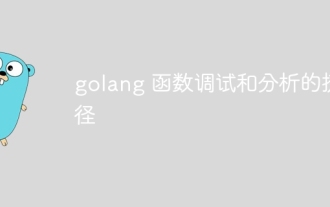
This article introduces shortcuts for Go function debugging and analysis, including: built-in debugger dlv, which is used to pause execution, check variables, and set breakpoints. Logging, use the log package to record messages and view them during debugging. The performance analysis tool pprof generates call graphs and analyzes performance, and uses gotoolpprof to analyze data. Practical case: Analyze memory leaks through pprof and generate a call graph to display the functions that cause leaks.
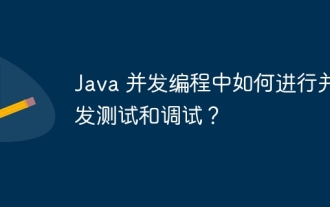
Concurrency testing and debugging Concurrency testing and debugging in Java concurrent programming are crucial and the following techniques are available: Concurrency testing: Unit testing: Isolate and test a single concurrent task. Integration testing: testing the interaction between multiple concurrent tasks. Load testing: Evaluate an application's performance and scalability under heavy load. Concurrency Debugging: Breakpoints: Pause thread execution and inspect variables or execute code. Logging: Record thread events and status. Stack trace: Identify the source of the exception. Visualization tools: Monitor thread activity and resource usage.
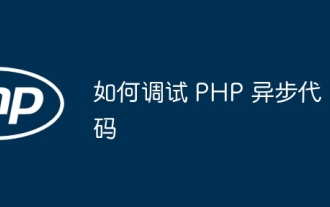
Tools for debugging PHP asynchronous code include: Psalm: a static analysis tool that can find potential errors. ParallelLint: A tool that inspects asynchronous code and provides recommendations. Xdebug: An extension for debugging PHP applications by enabling a session and stepping through the code. Other tips include using logging, assertions, running code locally, and writing unit tests.
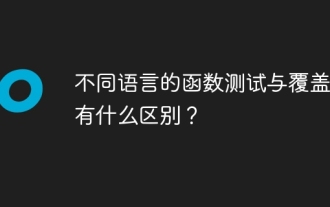
Functional testing verifies function functionality through black-box and white-box testing, while code coverage measures the portion of code covered by test cases. Different languages (such as Python and Java) have different testing frameworks, coverage tools and features. Practical cases show how to use Python's Unittest and Coverage and Java's JUnit and JaCoCo for function testing and coverage evaluation.
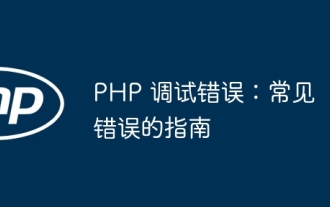
Common PHP debugging errors include: Syntax errors: Check the code syntax to make sure there are no errors. Undefined variable: Before using a variable, make sure it is initialized and assigned a value. Missing semicolons: Add semicolons to all code blocks. Function is undefined: Check that the function name is spelled correctly and make sure the correct file or PHP extension is loaded.
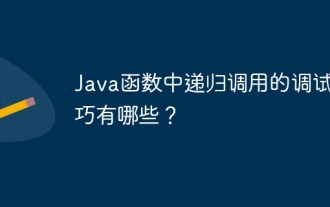
The following techniques are available for debugging recursive functions: Check the stack traceSet debug pointsCheck if the base case is implemented correctlyCount the number of recursive callsVisualize the recursive stack
