


Highly concurrent big data processing: implemented using Golang WaitGroup and coroutines
Highly concurrent big data processing: using Golang WaitGroup and coroutine implementation
Abstract: In today's information age, big data processing has become an important issue for various enterprises and organizations. core needs. In order to achieve high-concurrency big data processing, using Golang's WaitGroup and coroutines is an efficient and simple method. This article will introduce how to use Golang's WaitGroup and coroutines to implement high-concurrency big data processing, and attach specific code examples.
Keywords: high concurrency, big data processing, Golang, WaitGroup, coroutine
- Introduction
Nowadays, with the rapid development of Internet technology, big data has become The core needs of all walks of life. Applications that handle big data need to have high concurrency capabilities in order to be able to process large amounts of data efficiently. In the context of this growing demand, using Golang's WaitGroup and coroutines can help us achieve high-concurrency big data processing. - Golang WaitGroup
Golang's WaitGroup is a synchronization primitive that can be used to wait for the completion of a group of coroutines. When we start a group of coroutines, we can wait for the completion of these coroutines through WaitGroup to ensure that all coroutines have been executed before continuing to perform other operations. WaitGroup has three main methods: Add(), Done() and Wait().
- Add(): Add the number of coroutines to wait to WaitGroup through the Add() method;
- Done(): Notify WaitGroup of one through the Done() method The coroutine has been completed;
- Wait(): Wait for the completion of all coroutines added to the WaitGroup through the Wait() method.
- Coroutine
Coroutine is a lightweight thread that can run on an independent stack and is managed by a user-mode scheduler. In Golang, we can easily use the keyword go to start a coroutine. The startup of the coroutine will not block the main thread and tasks can be executed concurrently. This allows us to efficiently process large amounts of data in a concurrent manner. - Use Golang WaitGroup and coroutines to implement high-concurrency big data processing
Below we will use an example to show how to use Golang's WaitGroup and coroutines to implement high-concurrency big data processing.
package main import ( "fmt" "sync" ) func processData(data int, wg *sync.WaitGroup) { defer wg.Done() // 模拟数据处理过程 // 这里可以做一些复杂的计算、访问数据库等操作 result := data * 2 fmt.Printf("处理数据 %d,结果为 %d ", data, result) } func main() { var wg sync.WaitGroup // 设置要处理的数据集合 dataList := []int{1, 2, 3, 4, 5} // 设置WaitGroup等待的协程数量 wg.Add(len(dataList)) // 启动协程进行数据处理 for _, data := range dataList { go processData(data, &wg) } // 等待所有协程完成 wg.Wait() fmt.Println("所有数据处理完成") }
In the above code, we first define a processData()
function to simulate the data processing process. In the main function, we create a WaitGroup to wait for the completion of all coroutines. Then, we set the number of waiting coroutines through the Add()
method, and then use the keyword go to start the coroutine for data processing. Finally, wait for all coroutines to complete by calling the Wait()
method.
The above example shows how to use Golang's WaitGroup and coroutines to achieve high-concurrency big data processing. By using a WaitGroup to wait for all coroutines to complete, we can ensure that the data processing process is not interrupted and continue operations after all data processing is completed.
- Conclusion
In big data processing, achieving high concurrency is the key to improving system performance, and using Golang's WaitGroup and coroutines is an efficient and simple method. By using WaitGroup to wait for the completion of all coroutines, we can process large amounts of data with high concurrency and improve the response speed and efficiency of the system. Using Golang's WaitGroup and coroutines can make it easier for us to achieve high-concurrency big data processing needs.
Reference:
- Go Concurrency Patterns: https://blog.golang.org/concurrency-patterns
- Go Language Specification: https: //golang.org/ref/spec
(Word count: 737 words)
The above is the detailed content of Highly concurrent big data processing: implemented using Golang WaitGroup and coroutines. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


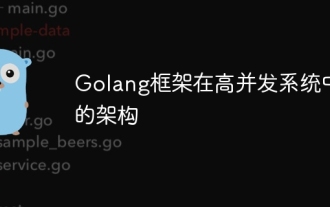
For high-concurrency systems, the Go framework provides architectural modes such as pipeline mode, Goroutine pool mode, and message queue mode. In practical cases, high-concurrency websites use Nginx proxy, Golang gateway, Goroutine pool and database to handle a large number of concurrent requests. The code example shows the implementation of a Goroutine pool for handling incoming requests. By choosing appropriate architectural patterns and implementations, the Go framework can build scalable and highly concurrent systems.
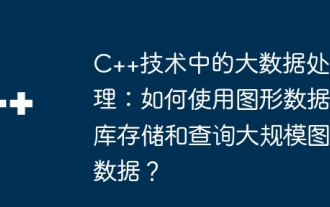
C++ technology can handle large-scale graph data by leveraging graph databases. Specific steps include: creating a TinkerGraph instance, adding vertices and edges, formulating a query, obtaining the result value, and converting the result into a list.
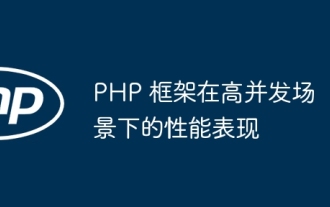
In high-concurrency scenarios, according to benchmark tests, the performance of the PHP framework is: Phalcon (RPS2200), Laravel (RPS1800), CodeIgniter (RPS2000), and Symfony (RPS1500). Actual cases show that the Phalcon framework achieved 3,000 orders per second during the Double Eleven event on the e-commerce website.
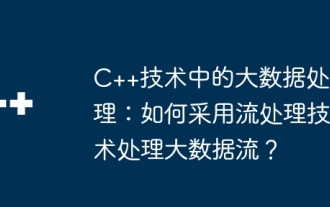
Stream processing technology is used for big data processing. Stream processing is a technology that processes data streams in real time. In C++, Apache Kafka can be used for stream processing. Stream processing provides real-time data processing, scalability, and fault tolerance. This example uses ApacheKafka to read data from a Kafka topic and calculate the average.
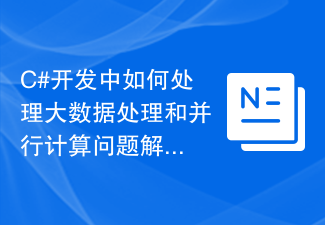
How to deal with big data processing and parallel computing problem solving in C# development requires specific code examples In the current information age, the amount of data is growing exponentially. For developers, dealing with big data and parallel computing has become an important task. In C# development, we can use some technologies and tools to solve these problems. This article will introduce some common workarounds and specific code examples. 1. Use the parallel library C# provides a parallel library (Parallel), which is designed to simplify the use of parallel programming.
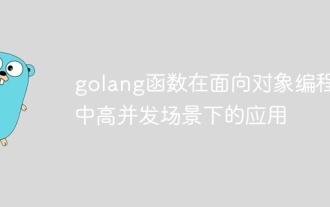
In high-concurrency scenarios of object-oriented programming, functions are widely used in the Go language: Functions as methods: Functions can be attached to structures to implement object-oriented programming, conveniently operating structure data and providing specific functions. Functions as concurrent execution bodies: Functions can be used as goroutine execution bodies to implement concurrent task execution and improve program efficiency. Function as callback: Functions can be passed as parameters to other functions and be called when specific events or operations occur, providing a flexible callback mechanism.
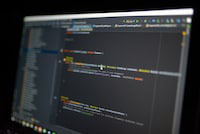
Asynchronous programming, English Asynchronous Programming, means that certain tasks in the program can be executed concurrently without waiting for other tasks to complete, thereby improving the overall operating efficiency of the program. In Python, the asyncio module is the main tool for implementing asynchronous programming. It provides coroutines, event loops, and other components required for asynchronous programming. Coroutine: Coroutine is a special function that can be suspended and then resumed execution, just like a thread, but a coroutine is more lightweight and consumes less memory than a thread. The coroutine is declared with the async keyword and execution is suspended at the await keyword. Event loop: Event loop (EventLoop) is the key to asynchronous programming
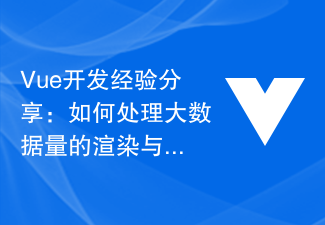
Vue development experience sharing: How to handle the rendering and optimization of large amounts of data. With the rapid development of Internet technology, the increasing amount of data has become a common problem. In front-end development, using the Vue framework to build web applications has become a common choice. However, when we face large amounts of data, Vue's rendering performance may be affected, leading to application performance degradation. This article will share some experience in handling large data volume rendering and optimization, hoping to be helpful to Vue developers. Use a virtual list (Vir
