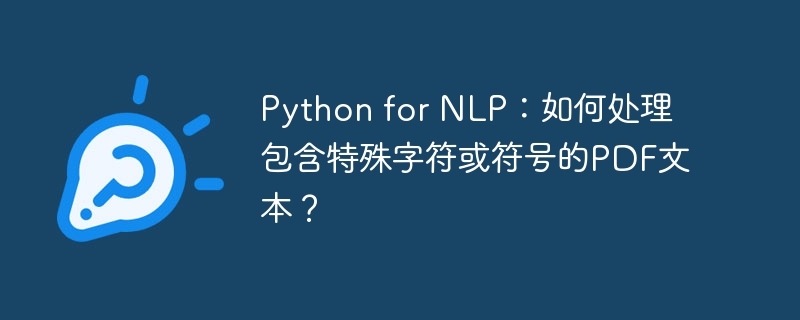
Python for NLP: How to handle PDF text containing special characters or symbols?
Abstract: PDF is a common document format, but PDF text containing special characters or symbols can be a challenge for natural language processing (NLP) tasks. This article will introduce how to use Python to process such PDF text and provide specific code examples.
- Introduction
Natural language processing (NLP) is an important research direction in the fields of computer science and artificial intelligence. In NLP tasks, we usually need to process and analyze text data. PDF is a common document format that contains rich text content. However, PDF text may contain special characters or symbols, which may be a challenge for NLP tasks.
- Python library installation
In order to process PDF text, we need to install some Python libraries. The following libraries need to be installed:
- PyPDF2: used to parse and extract PDF text content.
- NLTK (Natural Language Toolkit): used for text processing and analysis in NLP tasks.
- Pandas: for data processing and analysis.
These libraries can be installed using the following command:
pip install PyPDF2
pip install nltk
pip install pandas
Copy after login
- Parsing and extracting PDF text content
The following code example demonstrates how to use the PyPDF2 library to parse and extract PDF Text content:
import PyPDF2
def extract_text_from_pdf(pdf_path):
text = ""
with open(pdf_path, "rb") as f:
pdf = PyPDF2.PdfReader(f)
for page in pdf.pages:
text += page.extract_text()
return text
pdf_path = "example.pdf"
text = extract_text_from_pdf(pdf_path)
print(text)
Copy after login
- Handling special characters or symbols
When we extract PDF text content, we may encounter special characters or symbols, such as Unicode characters, spaces, newlines, etc. . These special characters or symbols may interfere with the performance of NLP tasks. The following code example demonstrates how to handle these special characters or symbols:
import re
# 清除特殊字符或符号
def clean_text(text):
clean_text = re.sub(r"[^ws]", "", text)
return clean_text
cleaned_text = clean_text(text)
print(cleaned_text)
Copy after login
In the above code, we have used regular expressions to clear special characters or symbols. re.sub(r"[^ws]", "", text)
This line of code will match all characters except letters, numbers, underscores and spaces and replace them with the empty string .
- Text Processing and Analysis
Once we have extracted and cleaned the PDF text content, we can use the NLTK library for further text processing and analysis. The following code example demonstrates how to use the NLTK library for text tokenization and word frequency counting:
from nltk.tokenize import word_tokenize
from nltk.probability import FreqDist
# 文本标记化
tokens = word_tokenize(cleaned_text)
# 词频统计
fdist = FreqDist(tokens)
print(fdist.most_common(10))
Copy after login
In the above code, we have used the word_tokenize
function pair in the NLTK library Text is tokenized, splitting the text into words or tokens. Then, we use the FreqDist
function to count the word frequency of each word and output the top 10 words with the highest frequency.
- Conclusion
This article introduces how to use Python to process PDF text that contains special characters or symbols. By using the PyPDF2 library to parse and extract PDF text content, and using the NLTK library for text processing and analysis, we can efficiently handle such PDF text. I hope the content of this article will be helpful to readers who deal with PDF text in NLP tasks.
References:
- PyPDF2: https://github.com/mstamy2/PyPDF2
- NLTK: https://www.nltk. org/
- Pandas: https://pandas.pydata.org/
The above is the detailed content of Python for NLP: How to handle PDF text containing special characters or symbols?. For more information, please follow other related articles on the PHP Chinese website!