


Python for NLP: How to extract and analyze image description text from PDF files?
Python for NLP: How to extract and analyze image description text from PDF files?
Abstract: This article will introduce how to use the PDF library and OCR (Optical Character Recognition) library in Python to extract image description text (Image Description Text) from PDF files and conduct further analysis and processing. We will explain each step of the implementation process through specific code examples.
- Introduction
With the rapid development of machine learning and natural language processing technology, people's demand for extracting information from multimedia data is also increasing. Among them, extracting image description text from PDF files is a common task. For example, in scientific research, we may need to extract picture descriptions of experimental results from scientific papers for further analysis and verification. - Preparation
Before starting, we need to install the following Python libraries: - PyPDF2: for reading and processing PDF files.
- Pytesseract: An OCR library for recognizing text in images.
- Wand: A Python library for image processing.
You can use the pip command to install these libraries:
1 |
|
Extract images
First, we need to extract all the images from the PDF file, and save it locally. The following is a code example to obtain the image list:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
import PyPDF2
from wand.image import Image
filename =
'example.pdf'
pdf = PyPDF2.PdfFileReader(open(filename,
'rb'
))
images = []
for
page_num in range(pdf.numPages):
image_blob = pdf.getPage(page_num).extract_images()
for
img in image_blob:
images.append(img[0])
# 保存图片
for
idx, img in enumerate(images):
img_file =
'image_{}.png'
.format(idx)
try
:
img.save(filename=img_file)
except Exception
as
e:
print
(e)
Copy after loginImage text recognition
Next, we use the Pytesseract library to perform OCR on the saved image and extract the text in the image .1
2
3
4
5
6
7
8
import pytesseract
image_text = []
for
img_file in image_files:
text = pytesseract.image_to_string(Image.open(img_file))
image_text.append(text)
print
(image_text)
Copy after loginText analysis and processing
Finally, we can further analyze and process the image description text. For example, we can calculate the word frequency of each image description text to obtain common words and phrases. Here is a sample code for calculating the 5 most frequent words in each image description text:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
import re
from collections import Counter
# 合并所有图片描述文本
all_text =
' '
.join(image_text)
# 去除标点符号和多余空格
clean_text = re.sub(r
'[^ws]'
,
''
, all_text)
clean_text = re.sub(r
's+'
,
' '
, clean_text)
# 统计词频
words = clean_text.split()
word_freq = Counter(words)
top_words = word_freq.most_common(5)
print
(top_words)
Copy after loginConclusion
In this article, we introduced how to use Python The PDF library and OCR library in the tool extract and analyze image description text from PDF files. We demonstrate each step of the implementation process with specific code examples. I hope this article can help you better understand and apply Python in practical applications in NLP.References:
- https://pypi.org/project/PyPDF2/
- https://pypi.org/project/pytesseract/
- https://pypi.org/project/Wand/
The above is the detailed content of Python for NLP: How to extract and analyze image description text from PDF files?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
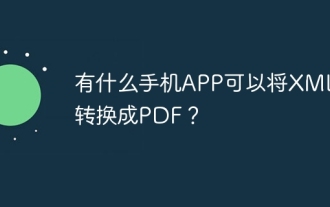
An application that converts XML directly to PDF cannot be found because they are two fundamentally different formats. XML is used to store data, while PDF is used to display documents. To complete the transformation, you can use programming languages and libraries such as Python and ReportLab to parse XML data and generate PDF documents.
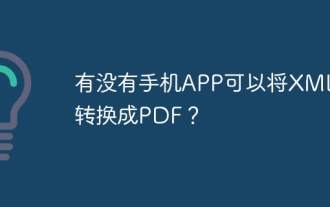
There is no APP that can convert all XML files into PDFs because the XML structure is flexible and diverse. The core of XML to PDF is to convert the data structure into a page layout, which requires parsing XML and generating PDF. Common methods include parsing XML using Python libraries such as ElementTree and generating PDFs using ReportLab library. For complex XML, it may be necessary to use XSLT transformation structures. When optimizing performance, consider using multithreaded or multiprocesses and select the appropriate library.
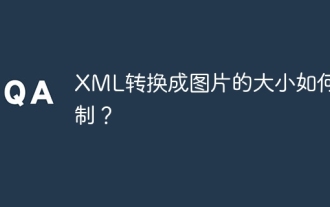
To generate images through XML, you need to use graph libraries (such as Pillow and JFreeChart) as bridges to generate images based on metadata (size, color) in XML. The key to controlling the size of the image is to adjust the values of the <width> and <height> tags in XML. However, in practical applications, the complexity of XML structure, the fineness of graph drawing, the speed of image generation and memory consumption, and the selection of image formats all have an impact on the generated image size. Therefore, it is necessary to have a deep understanding of XML structure, proficient in the graphics library, and consider factors such as optimization algorithms and image format selection.
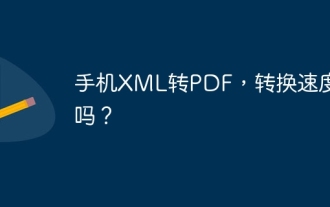
The speed of mobile XML to PDF depends on the following factors: the complexity of XML structure. Mobile hardware configuration conversion method (library, algorithm) code quality optimization methods (select efficient libraries, optimize algorithms, cache data, and utilize multi-threading). Overall, there is no absolute answer and it needs to be optimized according to the specific situation.
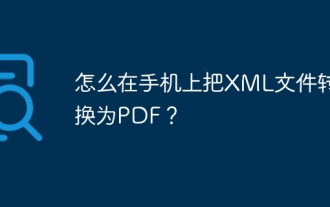
It is impossible to complete XML to PDF conversion directly on your phone with a single application. It is necessary to use cloud services, which can be achieved through two steps: 1. Convert XML to PDF in the cloud, 2. Access or download the converted PDF file on the mobile phone.
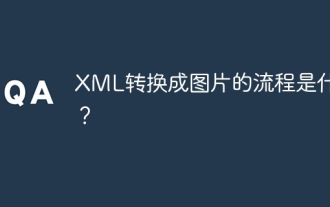
To convert XML images, you need to determine the XML data structure first, then select a suitable graphical library (such as Python's matplotlib) and method, select a visualization strategy based on the data structure, consider the data volume and image format, perform batch processing or use efficient libraries, and finally save it as PNG, JPEG, or SVG according to the needs.
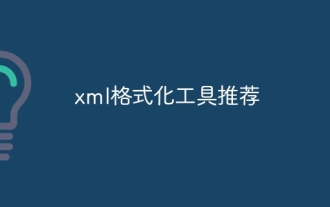
XML formatting tools can type code according to rules to improve readability and understanding. When selecting a tool, pay attention to customization capabilities, handling of special circumstances, performance and ease of use. Commonly used tool types include online tools, IDE plug-ins, and command-line tools.
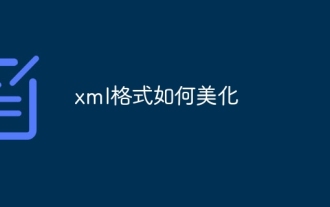
XML beautification is essentially improving its readability, including reasonable indentation, line breaks and tag organization. The principle is to traverse the XML tree, add indentation according to the level, and handle empty tags and tags containing text. Python's xml.etree.ElementTree library provides a convenient pretty_xml() function that can implement the above beautification process.
