


Tips for implementing image recognition and search using Elasticsearch in PHP development
Techniques for implementing image recognition and search using Elasticsearch in PHP development
Introduction: With the development of machine learning and artificial intelligence, image recognition technology has been widely used in various fields. Wide range of applications. In PHP development, using Elasticsearch to implement image recognition and search is an efficient and powerful way. This article will introduce how to use Elasticsearch to implement image recognition and search, and attach specific code examples to help readers better understand and practice.
1. Preparation work
Before we start, we need to do some preparation work. First, make sure you have a PHP environment and Elasticsearch installed. You can use Composer to install the Elasticsearch client library, for example "elasticsearch/elasticsearch": ">=6.0".
2. Principle of Image Recognition
Image recognition refers to processing and analyzing images through computers to identify specific objects or features in the images. Elasticsearch is an open source search and analysis engine with flexible data processing and search capabilities. Combining the two, we can implement image recognition and search functions.
3. Build the index
First, we need to build the image data into an index. An index is a data structure in Elasticsearch that is used to organize and store data. We can use Elasticsearch's RESTful API to send image data to Elasticsearch in JSON format to build the index.
The specific code examples are as follows:
require 'vendor/autoload.php'; $client = ElasticsearchClientBuilder::create()->build(); $params = [ 'index' => 'images', 'body' => [ 'mappings' => [ 'properties' => [ 'image' => [ 'type' => 'binary', ], 'tags' => [ 'type' => 'keyword', ], ], ], ], ]; $response = $client->indices()->create($params);
The above code snippet creates an index named images
and defines two fields image
(used to store image data) and tags
(used to mark relevant tag information of images).
4. Upload image data
Next, we need to upload the image data to Elasticsearch. You can use Elasticsearch's RESTful API to send image data to Elasticsearch through HTTP requests.
The specific code examples are as follows:
$imageData = file_get_contents('/path/to/image.jpg'); $params = [ 'index' => 'images', 'body' => [ 'image' => base64_encode($imageData), 'tags' => ['sunset', 'beach'], ], ]; $response = $client->index($params);
The above code snippet stores the image data in Elasticsearch in base64
encoding, and uses the tags
field Associate related label information.
5. Image Search
After the image data is uploaded, we can perform image search through Elasticsearch. Using Elasticsearch's search API, we can search using the characteristics of images and return the image data that best matches the search results.
The specific code examples are as follows:
$params = [ 'index' => 'images', 'body' => [ 'query' => [ 'match' => [ 'tags' => 'sunset', ], ], ], ]; $response = $client->search($params);
The above code snippet will use the tags
field to search and match the image data with the tag sunset
.
6. Image recognition skills
In order to improve the accuracy of image recognition, we can combine machine learning algorithms and use existing image recognition models for image recognition. You can use deep learning frameworks such as TensorFlow and Caffe to train and export your own models, and then use the models with Elasticsearch.
The specific code examples are as follows:
require 'vendor/autoload.php'; $graph = new TensorFlowGraph(); $graph->import(new TensorFlowFilesystemLoader('path/to/model.pb')); $tensor = $graph->createTensorFromPath('path/to/image.jpg'); $session = new TensorFlowSession($graph); $output = $session->return([$tensor]); $prediction = $output[0]->data(); $params = [ 'index' => 'images', 'body' => [ 'query' => [ 'match' => [ 'prediction' => $prediction, ], ], ], ]; $response = $client->search($params);
The above code snippet uses the TensorFlow framework to import the trained model, predict the image data, and use the prediction results for Elasticsearch image search.
Summary: By using PHP and Elasticsearch, we can implement image recognition and search functions. First, we need to build the index and then upload the image data to Elasticsearch. Next, we can use Elasticsearch for image search. To improve image recognition accuracy, we can also combine machine learning algorithms with existing image recognition models. The above is an introduction to the techniques of image recognition and search using Elasticsearch in PHP development. I hope it will be helpful to readers.
(Note: The above code examples are only for reference and understanding. In actual applications, please modify and optimize according to specific needs.)
The above is the detailed content of Tips for implementing image recognition and search using Elasticsearch in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
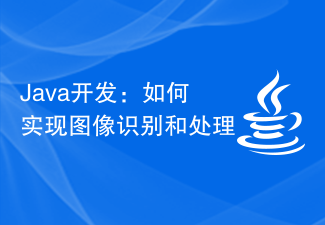
Java Development: A Practical Guide to Image Recognition and Processing Abstract: With the rapid development of computer vision and artificial intelligence, image recognition and processing play an important role in various fields. This article will introduce how to use Java language to implement image recognition and processing, and provide specific code examples. 1. Basic principles of image recognition Image recognition refers to the use of computer technology to analyze and understand images to identify objects, features or content in the image. Before performing image recognition, we need to understand some basic image processing techniques, as shown in the figure
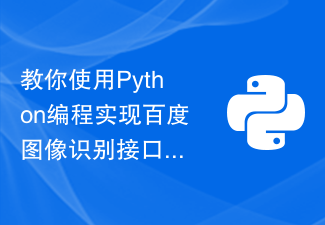
Teach you to use Python programming to implement the docking of Baidu's image recognition interface and realize the image recognition function. In the field of computer vision, image recognition technology is a very important technology. Baidu provides a powerful image recognition interface through which we can easily implement image classification, labeling, face recognition and other functions. This article will teach you how to use the Python programming language to realize the image recognition function by connecting to the Baidu image recognition interface. First, we need to create an application on Baidu Developer Platform and obtain
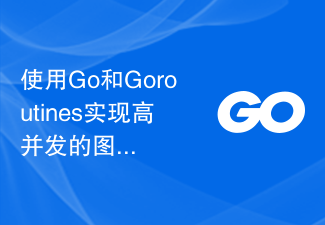
Using Go and Goroutines to implement a highly concurrent image recognition system Introduction: In today's digital world, image recognition has become an important technology. Through image recognition, we can convert information such as objects, faces, scenes, etc. in images into digital data. However, for recognition of large-scale image data, speed often becomes a challenge. In order to solve this problem, this article will introduce how to use Go language and Goroutines to implement a high-concurrency image recognition system. Background: Go language

How to do image processing and recognition in Python Summary: Modern technology has made image processing and recognition an important tool in many fields. Python is an easy-to-learn and use programming language with rich image processing and recognition libraries. This article will introduce how to use Python for image processing and recognition, and provide specific code examples. Image processing: Image processing is the process of performing various operations and transformations on images to improve image quality, extract information from images, etc. PIL library in Python (Pi
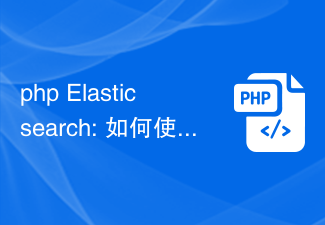
PHPElasticsearch: How to use dynamic mapping to achieve flexible search capabilities? Introduction: Search functionality is an integral part of developing modern applications. Elasticsearch is a powerful search and analysis engine that provides rich functionality and flexible data modeling. In this article, we will focus on how to use dynamic mapping to achieve flexible search capabilities. 1. Introduction to dynamic mapping In Elasticsearch, mapping (mapp
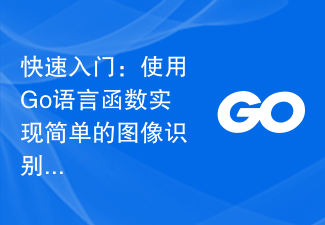
Quick Start: Use Go language functions to implement simple image recognition functions In today's technological development, image recognition technology has become a hot topic. As a fast and efficient programming language, Go language has the ability to implement image recognition functions. This article will provide readers with a quick start guide by using Go language functions to implement simple image recognition functions. First, we need to install the Go language development environment. You can download the appropriate version on the Go language official website (https://golang.org/)
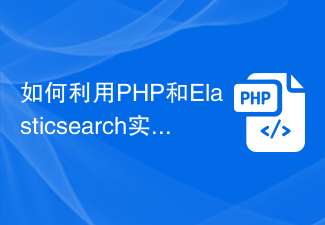
How to use PHP and Elasticsearch to achieve highlighted search results Introduction: In the modern Internet world, search engines have become the main way for people to obtain information. In order to improve the readability and user experience of search results, highlighting search keywords has become a common requirement. This article will introduce how to use PHP and Elasticsearch to achieve highlighted search results. 1. Preparation Before starting, we need to ensure that PHP and Elasticsearch have been installed and configured correctly.
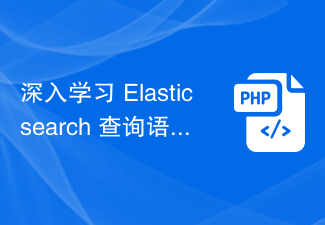
In-depth study of Elasticsearch query syntax and practical introduction: Elasticsearch is an open source search engine based on Lucene. It is mainly used for distributed search and analysis. It is widely used in full-text search of large-scale data, log analysis, recommendation systems and other scenarios. When using Elasticsearch for data query, flexible use of query syntax is the key to improving query efficiency. This article will delve into the Elasticsearch query syntax and give it based on actual cases.
