


How to optimize the cache update mechanism through php functions?
How to optimize the cache update mechanism through php functions?
Caching is an important part of improving website performance. In PHP development, we often use cache to reduce the load on the database and server and improve the access speed of the website. However, in the process of caching, we also face the problem of consistency between cache and data, especially when the data is updated. In order to maintain the consistency of cache and data, we can solve this problem by optimizing the cache update mechanism. This article will introduce how to optimize the cache update mechanism through PHP functions and provide specific code examples.
First of all, we need to understand the commonly used caching mechanisms and related PHP functions. In PHP, there are two commonly used caching mechanisms: file caching and memory caching. File caching saves data in files, while memory caching saves data in memory. Depending on the specific needs and performance requirements, we can choose the appropriate caching mechanism.
The following is a sample code using file caching:
function getFromCache($key) { $filename = 'cache/' . md5($key); if (file_exists($filename) && (time() - filemtime($filename) < 3600)) { return unserialize(file_get_contents($filename)); } return false; } function saveToCache($key, $data) { $filename = 'cache/' . md5($key); file_put_contents($filename, serialize($data)); } function clearCache($key) { $filename = 'cache/' . md5($key); if (file_exists($filename)) { unlink($filename); } }
In the above code, we define three functions: getFromCache, saveToCache and clearCache. The getFromCache function is used to get data from the cache, the saveToCache function is used to save the data to the cache, and the clearCache function is used to clear the cache.
Next, we need to consider how to update the cache. When data is updated, we need to save the new data to the cache and clear the old cache. In order to achieve this function, we can add the corresponding cache update code to the code that updates the data.
The following is a cache update sample code using file caching:
function updateData($newData) { // 更新数据库中的数据 // ... // 清除缓存 clearCache('data'); // 将新的数据保存到缓存中 saveToCache('data', $newData); }
In the above code, the updateData function is used to update data. After updating the data, we first call the clearCache function to clear the cache, and then call the saveToCache function to save the new data into the cache.
In addition to file caching, we can also use memory caching to optimize the cache update mechanism. In PHP, we can use extensions such as memcache or redis to implement memory caching.
The following is a sample code using memcache:
function getFromCache($key) { $memcache = new Memcache; $memcache->connect('localhost', 11211); return $memcache->get($key); } function saveToCache($key, $data) { $memcache = new Memcache; $memcache->connect('localhost', 11211); $memcache->set($key, $data, 0, 3600); } function clearCache($key) { $memcache = new Memcache; $memcache->connect('localhost', 11211); $memcache->delete($key); }
In the above code, we use the memcache extension to implement cache read, write and clear operations. When using memcache, we need to first create a Memcache object and call the connect function to connect to the memcache server.
To sum up, by using a suitable caching mechanism and optimizing the cache update mechanism, we can improve the performance and user experience of the website. In actual development, we need to choose an appropriate caching mechanism based on specific needs and performance requirements, and update the cache reasonably. Through the code examples provided in this article, we hope to help readers better understand and use the cache update mechanism.
The above is the detailed content of How to optimize the cache update mechanism through php functions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


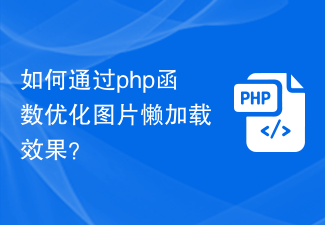
How to optimize the lazy loading effect of images through PHP functions? With the development of the Internet, the number of images in web pages is increasing, which puts pressure on page loading speed. In order to improve user experience and reduce loading time, we can use image lazy loading technology. Lazy loading of images can delay the loading of images. Images are only loaded when the user scrolls to the visible area, which can reduce the loading time of the page and improve the user experience. When writing PHP web pages, we can optimize the lazy loading effect of images by writing some functions. Details below
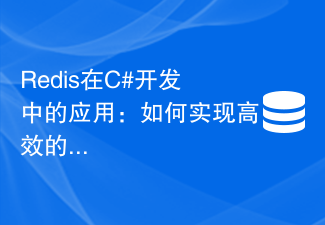
Application of Redis in C# development: How to achieve efficient cache updates Introduction: In Web development, caching is one of the common means to improve system performance. As a high-performance Key-Value storage system, Redis can provide fast caching operations, which brings a lot of convenience to our applications. This article will introduce how to use Redis in C# development to achieve efficient cache updates. Installation and configuration of Redis Before starting, we need to install Redis and configure it accordingly. you can
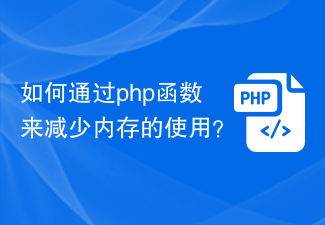
How to reduce memory usage through PHP functions. In development, memory usage is a very important consideration. If a large amount of memory is used in a program, it may cause slowdowns or even program crashes. Therefore, reasonably managing and reducing memory usage is an issue that every PHP developer should pay attention to. This article will introduce some methods to reduce memory usage through PHP functions, and provide specific code examples for readers' reference. Use the unset() function to release variables in PHP. When a variable is no longer needed, use
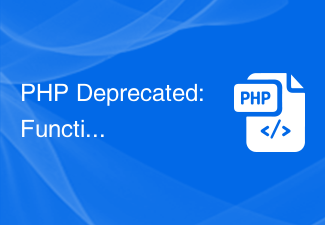
PHPDeprecated: Functionereg_replace()isdeprecated-Solution When developing in PHP, we often encounter the problem of some functions being declared deprecated. This means that in the latest PHP versions, these functions may be removed or replaced. One common example is the ereg_replace() function. ereg_replace
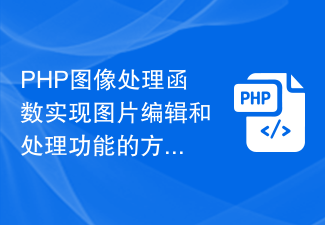
PHP image processing functions are a set of functions specifically used to process and edit images. They provide developers with rich image processing functions. Through these functions, developers can implement operations such as cropping, scaling, rotating, and adding watermarks to images to meet different image processing needs. First, I will introduce how to use PHP image processing functions to achieve image cropping function. PHP provides the imagecrop() function, which can be used to crop images. By passing the coordinates and size of the cropping area, we can crop the image

PHP function introduction: strtr() function In PHP programming, the strtr() function is a very useful string replacement function. It is used to replace specified characters or strings in a string with other characters or strings. This article will introduce the usage of strtr() function and give some specific code examples. The basic syntax of the strtr() function is as follows: strtr(string$str, array$replace) where $str is the original word to be replaced.
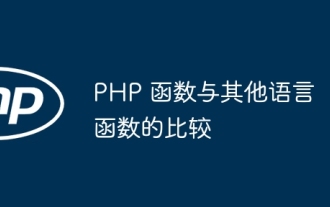
PHP functions have similarities with functions in other languages, but also have some unique features. Syntactically, PHP functions are declared with function, JavaScript is declared with function, and Python is declared with def. In terms of parameters and return values, PHP functions accept parameters and return a value. JavaScript and Python also have similar functions, but the syntax is different. In terms of scope, functions in PHP, JavaScript and Python all have global or local scope. Global functions can be accessed from anywhere, and local functions can only be accessed within their declaration scope.
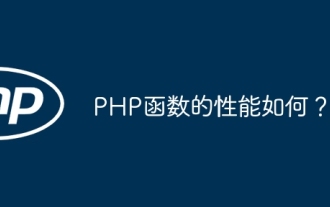
The performance of different PHP functions is crucial to application efficiency. Functions with better performance include echo and print, while functions such as str_replace, array_merge, and file_get_contents have slower performance. For example, the str_replace function is used to replace strings and has moderate performance, while the sprintf function is used to format strings. Performance analysis shows that it only takes 0.05 milliseconds to execute one example, proving that the function performs well. Therefore, using functions wisely can lead to faster and more efficient applications.
