How to use PHP scripts for Linux system management
How to use PHP scripts for Linux system management
Using PHP scripts in Linux system management can greatly simplify management operations and improve efficiency. The PHP language has good compatibility and powerful functions, and can easily implement management tasks on Linux systems. This article will introduce how to use PHP scripts for Linux system management and provide specific code examples.
1. Use PHP to execute Shell commands
PHP provides the functions exec() and system() for executing Shell commands. You can use these functions to execute Linux system commands. The following is an example of using PHP to execute a Shell command:
<?php $command = "ls -l"; $result = shell_exec($command); echo $result; ?>
The above code will execute the ls -l command and output the results to the page. Use this method to execute any command that can be executed in the terminal and get the results.
2. Operating files and directories
In Linux system management, we often need to operate files and directories. PHP provides a series of functions to complete these operations, such as mkdir(), rmdir(), chdir(), etc. Here are some common examples of file and directory operations:
- Create directory:
<?php $dir = "/path/to/new/dir"; if (!file_exists($dir)) { mkdir($dir, 0777, true); echo "目录创建成功!"; } else { echo "目录已存在!"; } ?>
- Delete directory:
<?php $dir = "/path/to/dir"; if (file_exists($dir)) { rmdir($dir); echo "目录删除成功!"; } else { echo "目录不存在!"; } ?>
- Switch directory:
<?php $dir = "/path/to/dir"; if (file_exists($dir)) { chdir($dir); echo "目录切换成功!"; } else { echo "目录不存在!"; } ?>
- Create file:
<?php $file = "/path/to/new/file.txt"; if (!file_exists($file)) { fopen($file, "w"); echo "文件创建成功!"; } else { echo "文件已存在!"; } ?>
- Delete file:
<?php $file = "/path/to/file.txt"; if (file_exists($file)) { unlink($file); echo "文件删除成功!"; } else { echo "文件不存在!"; } ?>
3. Management Process
In Linux systems, we often need to manage and monitor processes. PHP provides functions such as proc_open() and proc_close() to manage processes. The following are some common examples of process management operations:
- Start the process:
<?php $command = "/path/to/program"; $descriptorspec = array( 0 => array("pipe", "r"), // 标准输入 1 => array("pipe", "w"), // 标准输出 2 => array("pipe", "w") // 标准错误输出 ); $process = proc_open($command, $descriptorspec, $pipes); if (is_resource($process)) { echo "进程启动成功!"; proc_close($process); } else { echo "进程启动失败!"; } ?>
- Terminate the process:
<?php $pid = 1234; // 进程PID exec("kill $pid", $output, $retval); if ($retval == 0) { echo "进程终止成功!"; } else { echo "进程终止失败!"; } ?>
4. Management system configuration
In Linux system management, we often need to modify the system configuration file. PHP provides some functions to read and write configuration files, such as file_get_contents() and file_put_contents(). The following is an example of modifying the system configuration file:
<?php $file = "/etc/php.ini"; // 配置文件路径 if (file_exists($file)) { $content = file_get_contents($file); $content = str_replace("memory_limit = 128M", "memory_limit = 256M", $content); file_put_contents($file, $content); echo "配置文件修改成功!"; } else { echo "配置文件不存在!"; } ?>
The above are some basic operations and sample codes for Linux system management using PHP scripts. Through these operations, we can easily implement management tasks on Linux systems and improve work efficiency. Of course, there are more functions that can be implemented using PHP, and the code needs to be written according to the specific tasks and needs. I hope this article can be helpful to everyone.
The above is the detailed content of How to use PHP scripts for Linux system management. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


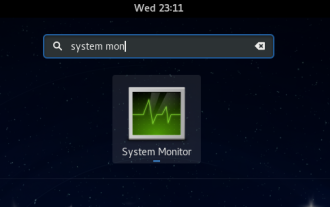
There are many questions that Linux beginners often ask, "Does Linux have a Task Manager?", "How to open the Task Manager on Linux?" Users from Windows know that the Task Manager is very useful. You can open the Task Manager by pressing Ctrl+Alt+Del in Windows. This task manager shows you all the running processes and the memory they consume, and you can select and kill a process from the task manager program. When you first use Linux, you will also look for something that is equivalent to a task manager in Linux. A Linux expert prefers to use the command line to find processes, memory consumption, etc., but you don't have to
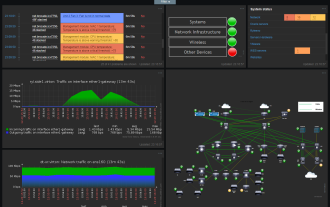
Zabbix's support for Chinese is not very good, but sometimes we still choose Chinese for management purposes. In the web interface monitored by Zabbix, the Chinese under the graphic icon will display small squares. This is incorrect and requires downloading fonts. For example, "Microsoft Yahei", "Microsoft Yahei.ttf" is named "msyh.ttf", upload the downloaded font to /zabbix/fonts/fonts and modify the two characters in the /zabbix/include/defines.inc.php file at define('ZBX_GRAPH_FONT_NAME','DejaVuSans');define('ZBX_FONT_NAME'
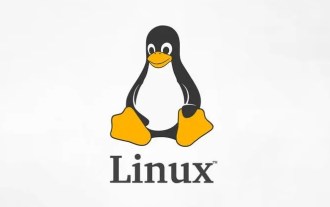
Did you know, how to check the creation date of an account on a Linux system? If you know, what can you do? Did you succeed? If yes, how to do it? Basically Linux systems don't track this information, so what are the alternative ways to get this information? You may ask why am I checking this? Yes, there are situations where you may need to review this information and it will be helpful to you at that time. You can use the following 7 methods to verify. Use /var/log/secure Use aureport tool Use .bash_logout Use chage command Use useradd command Use passwd command Use last command Method 1: Use /var/l
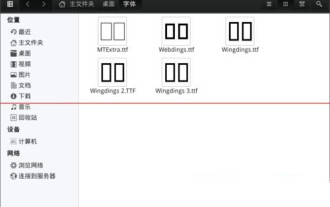
1. Find the fonts wingdings, wingdings2, wingdings3, Webdings, and MTExtra from the Internet. 2. Enter the main folder, press Ctrl+h (show hidden files), and check if there is a .fonts folder. If not, create one. 3. Copy the downloaded fonts such as wingdings, wingdings2, wingdings3, Webdings, and MTExtra to the .fonts folder in the main folder. Then start wps to see if there is still a "System missing font..." reminder dialog box. If not, just Success! Notes: wingdings, wingdin
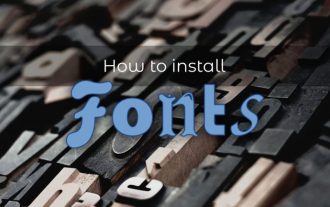
System-wide installation If you install a font system-wide, it will be available to all users. The best way to do this is to use RPM packages from the official software repositories. Before starting, open the "Software" tool in Fedora Workstation, or other tools using the official repository. Select the "Add-ons" category in the selection bar. Then select "Fonts" within the category. You'll see the available fonts similar to the ones in the screenshot below: When you select a font, some details will appear. Depending on several scenarios, you may be able to preview some sample text for the font. Click the "Install" button to add it to your system. Depending on system speed and network bandwidth, this process may take some time to complete
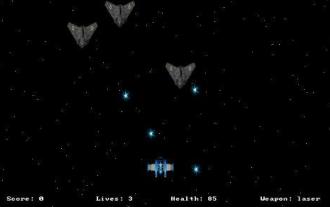
Different CPU architectures mean that running DOS on the Raspberry Pi is not easy, but it is not much trouble. FreeDOS may be familiar to everyone. It is a complete, free and well-compatible operating system for DOS. It can run some older DOS games or commercial software, and can also develop embedded applications. As long as the program can run on MS-DOS, it can run on FreeDOS. As the initiator and project coordinator of FreeDOS, many users will ask me questions as an insider. The question I get asked most often is: "Can FreeDOS run on a Raspberry Pi?" This question is not surprising. After all, Linux runs very well on the Raspberry Pi
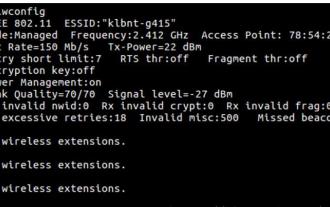
How to use one network cable to connect two ubuntu hosts to the Internet 1. Prepare host A: ubuntu16.04 and host B: ubuntu16.042. Host A has two network cards, one is connected to the external network and the other is connected to host B. Use the iwconfig command to view all network cards on the host. As shown above, the network cards on the author's A host (laptop) are: wlp2s0: This is a wireless network card. enp1s0: Wired network card, the network card connected to host B. The rest has nothing to do with us, no need to care. 3. Configure the static IP of A. Edit the file #vim/etc/network/interfaces to configure a static IP address for interface enp1s0, as shown below (where #==========
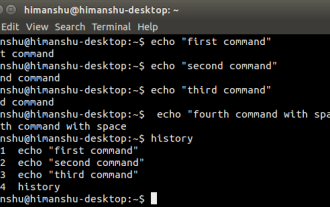
If you are a Linux command line user, sometimes you may not want certain commands to be recorded in your command line history. There could be many reasons, for example, you hold a certain position in a company and you have certain privileges that you don't want others to abuse. Or maybe there are some particularly important commands that you don't want to execute by mistake while browsing the history list. However, is there a way to control which commands go into the history list and which don't? Or in other words, can we enable incognito mode like a browser in a Linux terminal? The answer is yes, and depending on the specific goals you want, there are many ways to achieve it. In this article, we’ll discuss some proven methods. Note: All commands appearing in this article have been tested under Ubuntu. different
