


How to implement disk space monitoring through PHP script in Linux system
How to implement disk space monitoring through PHP scripts in Linux systems
Introduction:
Disk space monitoring is a very important task in system management. For Linux systems, we can implement automated disk space monitoring by writing PHP scripts. This article will introduce how to use PHP to obtain disk information, make judgments and alarms based on set thresholds, and provide specific code examples.
Step 1: Obtain disk information through PHP
To obtain disk information, we can use the shell command df of the Linux system. The following is an example of a PHP function that obtains disk information and prints it:
function get_disk_space() { $output = shell_exec('df -h'); $lines = explode(" ", trim($output)); $data = array(); foreach ($lines as $line) { $cols = preg_split('/s+/', $line); if (sizeof($cols) > 1 && is_numeric($cols[1])) { $data[] = array( 'filesystem' => $cols[0], 'size' => $cols[1], 'used' => $cols[2], 'avail' => $cols[3], 'percent' => $cols[4], 'mounted' => $cols[5], ); } } return $data; } $disk_space = get_disk_space(); print_r($disk_space);
In the above code, the df -h command is executed through the shell_exec function to obtain the disk information, and the results are divided by lines. Then, by looping through each row, use regular expressions to split the data of each row into an array and filter out the data with disk information. Finally, an array containing all disk information is returned.
Step 2: Judgment and alarm based on threshold value
In disk space monitoring, we usually set a threshold. When the disk usage space exceeds the threshold, an alarm needs to be issued in time. The following is an example function for judgment and alarm:
function check_disk_space($threshold) { $disk_space = get_disk_space(); foreach ($disk_space as $disk) { if (intval($disk['percent']) >= $threshold) { $message = "磁盘空间警报:文件系统 {$disk['filesystem']} 使用超过阈值 {$threshold}%"; // 发送警报邮件或其他操作 // ... echo $message . " "; } } } $threshold = 80; // 设置阈值为80% check_disk_space($threshold);
In the above code, we obtain disk information by calling the get_disk_space function. Then, traverse the disk information array, use the intval function to convert the disk usage percentage into an integer, and compare it with the set threshold. If the threshold is exceeded, an alert action is triggered, such as sending an alert email or performing other actions for disk space alerts.
Summary:
By writing the above code, we can use PHP scripts to monitor disk space in Linux systems. First, we call the df command through the shell_exec function to obtain the disk information, parse and save it into an array. Then, by setting the threshold, it is judged whether the set warning line is exceeded and the corresponding alarm operation is triggered. This method can help system administrators monitor disk space in a timely manner and prevent problems caused by insufficient disk space.
Note: The above code examples are for reference only, please make appropriate adjustments according to specific needs and environment.
The above is the detailed content of How to implement disk space monitoring through PHP script in Linux system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


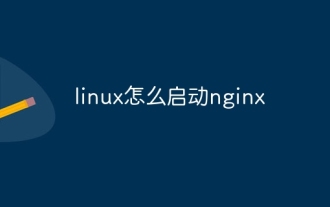
Steps to start Nginx in Linux: Check whether Nginx is installed. Use systemctl start nginx to start the Nginx service. Use systemctl enable nginx to enable automatic startup of Nginx at system startup. Use systemctl status nginx to verify that the startup is successful. Visit http://localhost in a web browser to view the default welcome page.
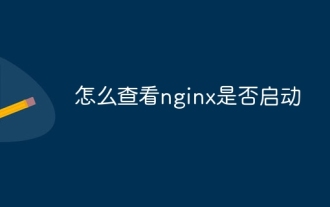
How to confirm whether Nginx is started: 1. Use the command line: systemctl status nginx (Linux/Unix), netstat -ano | findstr 80 (Windows); 2. Check whether port 80 is open; 3. Check the Nginx startup message in the system log; 4. Use third-party tools, such as Nagios, Zabbix, and Icinga.
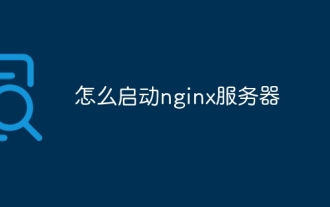
Starting an Nginx server requires different steps according to different operating systems: Linux/Unix system: Install the Nginx package (for example, using apt-get or yum). Use systemctl to start an Nginx service (for example, sudo systemctl start nginx). Windows system: Download and install Windows binary files. Start Nginx using the nginx.exe executable (for example, nginx.exe -c conf\nginx.conf). No matter which operating system you use, you can access the server IP
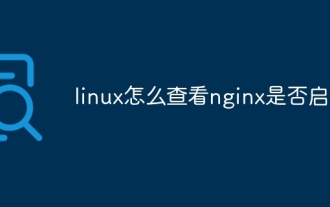
In Linux, use the following command to check whether Nginx is started: systemctl status nginx judges based on the command output: If "Active: active (running)" is displayed, Nginx is started. If "Active: inactive (dead)" is displayed, Nginx is stopped.
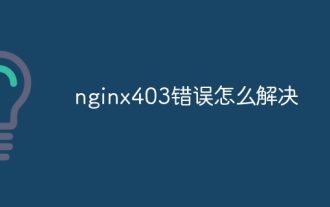
The server does not have permission to access the requested resource, resulting in a nginx 403 error. Solutions include: Check file permissions. Check the .htaccess configuration. Check nginx configuration. Configure SELinux permissions. Check the firewall rules. Troubleshoot other causes such as browser problems, server failures, or other possible errors.
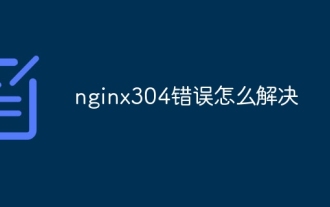
Answer to the question: 304 Not Modified error indicates that the browser has cached the latest resource version of the client request. Solution: 1. Clear the browser cache; 2. Disable the browser cache; 3. Configure Nginx to allow client cache; 4. Check file permissions; 5. Check file hash; 6. Disable CDN or reverse proxy cache; 7. Restart Nginx.
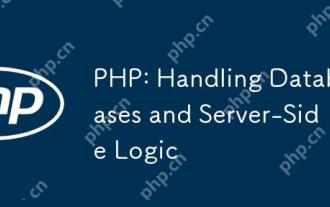
PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
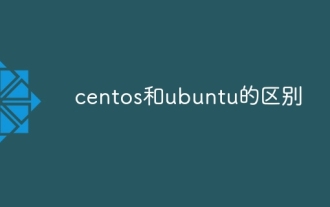
The key differences between CentOS and Ubuntu are: origin (CentOS originates from Red Hat, for enterprises; Ubuntu originates from Debian, for individuals), package management (CentOS uses yum, focusing on stability; Ubuntu uses apt, for high update frequency), support cycle (CentOS provides 10 years of support, Ubuntu provides 5 years of LTS support), community support (CentOS focuses on stability, Ubuntu provides a wide range of tutorials and documents), uses (CentOS is biased towards servers, Ubuntu is suitable for servers and desktops), other differences include installation simplicity (CentOS is thin)
