How to automate scripting operations on Linux using Java
How to use Java to write scripts to automate operations on Linux
In Linux systems, we can use Java to write scripts to automate operations. Java is a cross-platform programming language with powerful object-oriented features and rich class library support. In this article, we will learn how to use Java to write scripts to automate operations on Linux, and give specific code examples.
First, we need to install the Java Development Kit (JDK) on Linux. We can install it through the following steps:
-
Open the terminal and enter the following command to check whether the JDK is installed:
java -version
Copy after loginIf the JDK is already installed in the system, it will be displayed Installed Java version information. If it is not installed, you need to execute the following command to install JDK:
sudo apt update sudo apt install default-jdk
Copy after login - After the installation is complete, we can use any text editor to write Java scripts. Create a new file with the extension
.java
, for exampleScript.java
. In Java script, we first need to import the Java class library, for example:
import java.io.BufferedReader; import java.io.InputStreamReader;
Copy after loginNext, we can write our own automated operation logic. For example, we can write a script to execute a Linux terminal command and read the output, and then print the output to the console. The following is a sample code:
public class Script { public static void main(String[] args) { try { String command = "ls -l"; Process process = Runtime.getRuntime().exec(command); BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream())); String line; while ((line = reader.readLine()) != null) { System.out.println(line); } process.waitFor(); } catch (Exception e) { e.printStackTrace(); } } }
Copy after loginIn the above example, we use the
Runtime.getRuntime().exec(command)
method to execute thels -l
command , and useBufferedReader
to read the output of the command. We then useSystem.out.println(line)
to print each line of output to the console.After saving and exiting the file, we can use the following command to compile the Java script:
javac Script.java
Copy after loginAfter successful compilation, we can use the following command To run the script:
java Script
Copy after loginAfter execution, we will see the details of the files and folders of the current directory listed in the terminal.
In addition to executing Linux commands, we can also use Java scripts to implement other automated operations, such as file operations, network communications, error handling, etc. Java's rich class library allows us to easily implement these functions.
To summarize, it is feasible to automate operations on a Linux system by writing scripts in Java. We just need to install the JDK and write the appropriate Java code. Using Java's object-oriented programming features and rich class library support, we can implement various automated operations. The above is a simple example, you can write more complex scripts according to your needs to achieve more functions. Hope this article is helpful for writing scripts in Java for automation on Linux!
References:
- https://www.oracle.com/java/technologies/javase-jdk11-downloads.html
- https://www .w3schools.com/java/java_files_create.asp
The above is the detailed content of How to automate scripting operations on Linux using Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
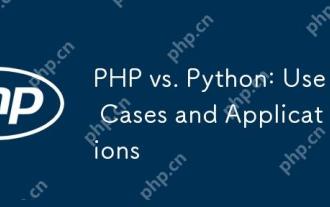
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
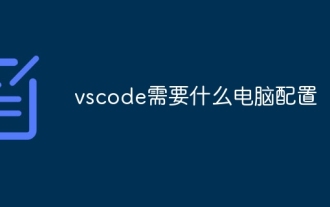
VS Code system requirements: Operating system: Windows 10 and above, macOS 10.12 and above, Linux distribution processor: minimum 1.6 GHz, recommended 2.0 GHz and above memory: minimum 512 MB, recommended 4 GB and above storage space: minimum 250 MB, recommended 1 GB and above other requirements: stable network connection, Xorg/Wayland (Linux)
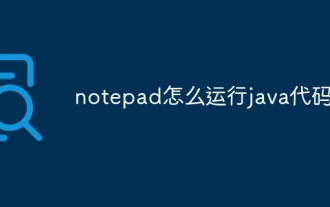
Although Notepad cannot run Java code directly, it can be achieved by using other tools: using the command line compiler (javac) to generate a bytecode file (filename.class). Use the Java interpreter (java) to interpret bytecode, execute the code, and output the result.
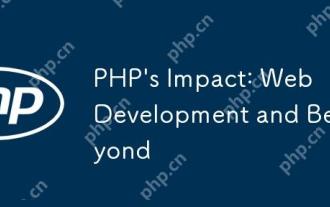
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
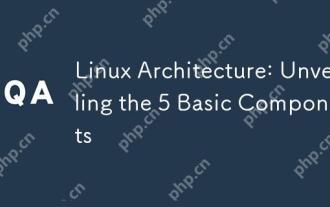
The five basic components of the Linux system are: 1. Kernel, 2. System library, 3. System utilities, 4. Graphical user interface, 5. Applications. The kernel manages hardware resources, the system library provides precompiled functions, system utilities are used for system management, the GUI provides visual interaction, and applications use these components to implement functions.
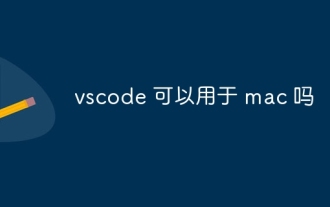
VS Code is available on Mac. It has powerful extensions, Git integration, terminal and debugger, and also offers a wealth of setup options. However, for particularly large projects or highly professional development, VS Code may have performance or functional limitations.
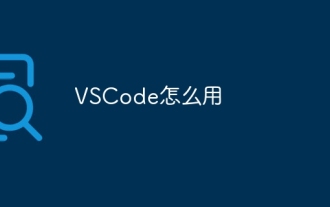
Visual Studio Code (VSCode) is a cross-platform, open source and free code editor developed by Microsoft. It is known for its lightweight, scalability and support for a wide range of programming languages. To install VSCode, please visit the official website to download and run the installer. When using VSCode, you can create new projects, edit code, debug code, navigate projects, expand VSCode, and manage settings. VSCode is available for Windows, macOS, and Linux, supports multiple programming languages and provides various extensions through Marketplace. Its advantages include lightweight, scalability, extensive language support, rich features and version
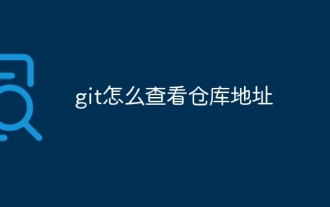
To view the Git repository address, perform the following steps: 1. Open the command line and navigate to the repository directory; 2. Run the "git remote -v" command; 3. View the repository name in the output and its corresponding address.
